JavaScript Array.map() Method
-
Syntax of JavaScript
array.map()
: -
Example Code: Use the
array.map()
Method to Round the Numbers in a Given Array -
Example Code: Use the
array.map()
Method to Iterate Over Every Element and Join the Values -
Example Code: Use the
array.map()
Method With thethis
Parameter to Get a Specific Output
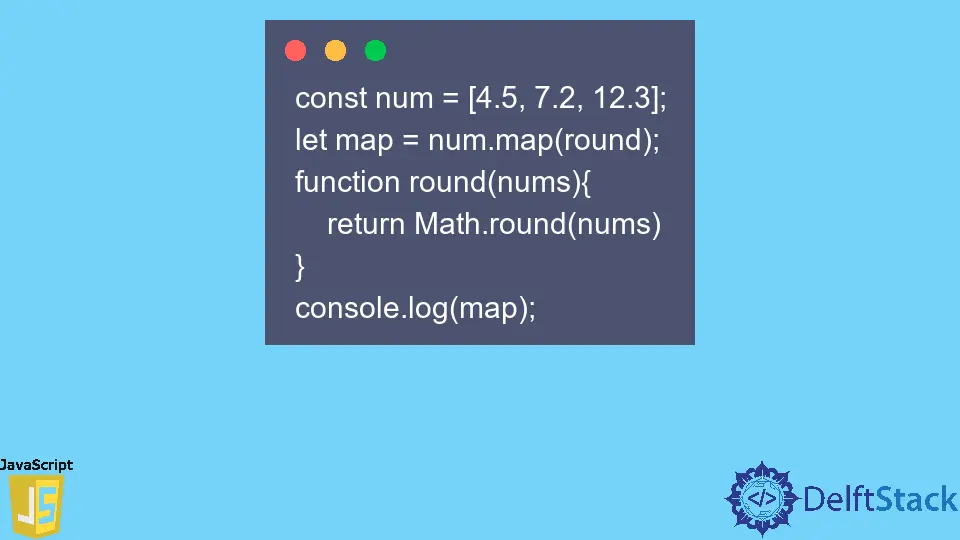
The array.map()
method can create a new array after running a specified function on every element of the given array. This method will not execute the function on empty elements and move to the next element of the given array.
Syntax of JavaScript array.map()
:
array.map(function(currentValue));
array.map(function(currentValue,index,arr),this);
Parameters
callback function() |
The function that will run for every array element to return a new array. |
currentValue |
The current element’s value will be used to create a new element for the array. |
index |
To use the current element’s index to execute the function. |
arr |
To move over the current array object. |
this |
Optionally used in the callback function to invoke array object. |
Return
After running a specified function, this method will return a new array that contains the results for each array element.
Example Code: Use the array.map()
Method to Round the Numbers in a Given Array
In JavaScript, we can use the array.map()
method to get the result for every element in an array after running a specified function.
In this example, we created an array with three floating numbers. We will round the numbers and get a new resultant array using the array.map()
method.
const num = [4.5, 7.2, 12.3];
let map = num.map(round);
function round(nums){
return Math.round(nums)
}
console.log(map);
Output:
[ 5, 7, 12 ]
Example Code: Use the array.map()
Method to Iterate Over Every Element and Join the Values
Every element of an array can contain different values. Using the array.map()
method, we can iterate over every element and join the values to create elements for the new array.
We have created an array that includes questions and answers in this example. We will use the array.map()
method with the currentValue
parameter to get both questions and answers values.
const array =[{Ques:"1",Ans:"False"},{Ques:"2",Ans:"True"},];
function getans(surv,index,arr){
return [surv.Ques,surv.Ans].join(": ");
}
console.log(array.map(getans));
Output:
['1: False', '2: True']
Example Code: Use the array.map()
Method With the this
Parameter to Get a Specific Output
Users can use the this
argument as an optional parameter with the array.map()
method.
In the example, we have passed the this
keyword as a parameter of array.map()
; we will use it inside the callback function. We have created the object of the Test
class and invoked the add
method by taking a reference of the object.
When we access the variable using the this
keyword inside the callback function, it refers to the variable of the reference object. Here, we are adding 3
to every array element.
class Test{
constructor() {
this.average = 3;
this.finalArr = [];
}
add(array) {
this.finalArr = array.map(function average(ele){
return ele + this.average;
},this);
}
}
const test = new Test();
test.add([2,6,10]);
console.log(test.finalArr);
Output:
[5, 9, 13]
The array.map()
method is supported on most browsers. Users can also use the array.map()
method to perform other operations on every array element, such as getting the square root of every element of the array.