How to Convert Object to String in JavaScript
-
Using JavaScript Inbuilt Function
JSON.stringify()
-
Using
console.log()
and%o
-
Convert JavaScript Object to String Using the
Object.enteries()
-
Convert a JavaScript Object to JSON String Using the
Object.enteries()
and Object Destructuring -
Convert JavaScript Object to a JSON String Using
Object.keys()
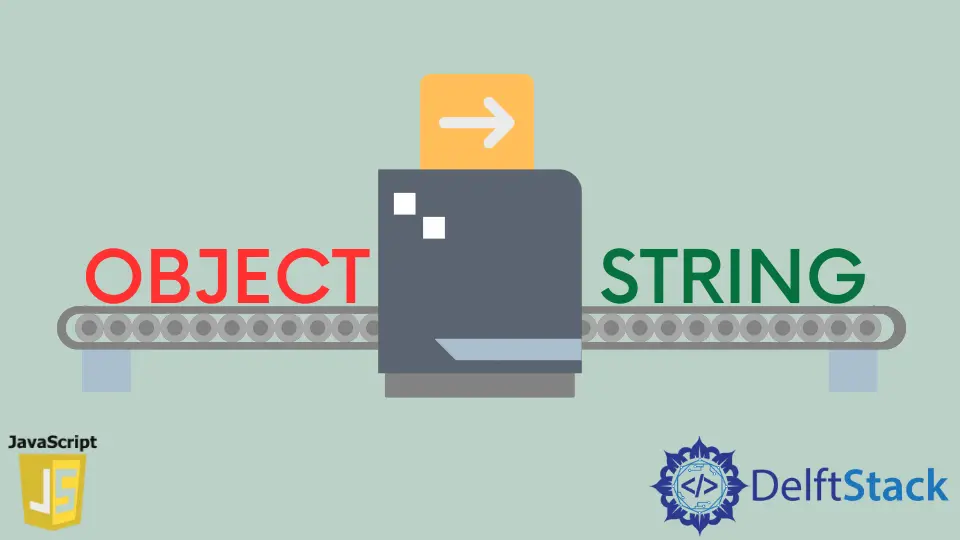
JavaScript objects consist of attributes in the form of key-value pairs. If we log them alongside a string, we see [object Object]
. It hides the object attributes underneath. While coding, we will need to convert the JavaScript objects to a string data type. Specifically when it comes to storing data into the localstorage
or a database. There are various ways in which we can convert a javascript object to a string.
Using JavaScript Inbuilt Function JSON.stringify()
JSON.stringify()
is a powerful and commonly used function to convert a javascript object to a JSON string. We can also use it to style the JSON representation for better readability. The function accepts three parameters:
javascriptObject
: The first parameter is a mandatory parameter where we pass the source JavaScript object that we need to convert to a JSON string.replacerFunction
: The second parameter is optional. It accepts a function by which we can alter the source javascript object. We can extract those object parameters which we wish to see in the final stringified JSON output. If we pass anull
value in this parameter, the function will include all the parameters from the source javascript object in its stringified JSON output.numberOfSpaces
: The final parameter is the number of spaces to be introduced before each parameter while the stringification is in process. It is a numeric parameter. If the parameter value is more than1
, it will format the stringified JSON with one or more spaces before each object parameter. It is purely intended for formatting purposes to make the output in a human-readable form.
Refer to the following code for usage.
var item = {};
item.id = 1;
item.name = 'icy-cream';
item.flavor = 'vanilla';
console.log('Item: ' + item);
console.log('Item: ' + JSON.stringify(item));
console.log('Item: ' + JSON.stringify(item, null, 2));
Output:
Item: [object Object]
Item: {"id":1,"name":"icy-cream","flavor":"vanilla"}
Item: {
"id": 1,
"name": "icy-cream",
"flavor": "vanilla"
}
The first log outputs a masked version of the javascript object. The second log has the string representation of the JSON object, but it is not formatted. The last log is the formatted version of the javascript object, which is quite readable.
Using console.log()
and %o
console.log()
is an inbuilt javascript function for logging values to the browser console. It accepts three parameters. The first parameter is the JavaScript object; the second parameter is the message to be displayed; the final parameter is a substring of the message to be logged in the web console. The following code shows converting javascript objects to a string using the console.log
method.
var item = {};
item.id = 1;
item.name = 'icy-cream';
item.flavor = 'vanilla';
console.log('Item: ' + item);
console.log('Item: %o', item);
Output:
Item: [object Object]
Item: {id: 1, name: "icy-cream", flavor: "vanilla"}
In the above code, the first command console.log("Item: " + item);
logs the object as [object Object]
. It hides the object parameters. Whereas, if we add %o
and pass the object as an argument, we can see the inner contents of the JavaScript object. We can also use %O
for logging the JavaScript object.
Note that the %o
in console.log
allows us to view the object in the console. But we cannot use it to convert the value and store it in some variable for further use.
Convert JavaScript Object to String Using the Object.enteries()
Object.enteries()
is an inbuilt JavaScript function. It splits the object into an array of [key, value]
pairs. Hence, we can iterate over such an array and manually convert the JavaScript object to a string.
var item = {};
item.id = 1;
item.name = 'icy-cream';
item.flavor = 'vanilla';
var stringifiedObj = Object.entries(item).map(x => x.join(':')).join('\n');
console.log('Item: ' + item);
console.log(`Item: \n{\n${stringifiedObj}\n}`);
Output:
Item: [object Object]
Item:
{
id:1
name:icy-cream
flavor:vanilla
}
In the code we first used the Object.enteries()
function to split the object into an array of small parameter-arrays. Next we convert sub array into a key:value
format by applying the javascript inbuilt join(":")
function. It converts the [key, value]
array output by the Object.enteries
to the key:value
format. And we finally log the JavaScript object in its string representation. It is a concise method which employs just a single piece of code, Object.entries(item).map(x=>x.join(":")).join("\n")
for the conversion process.
Convert a JavaScript Object to JSON String Using the Object.enteries()
and Object Destructuring
Another way of using the Object.enteries()
for the conversion is to use it alongside the object destructuring
concept of javascript. Using this technique, we destructure the array of key-value pairs output by the Object.enteries()
, iterate over it using a for loop
, and assign it to temporary variables param
and value
. We store the result of the iteration into the str
variable. Finally, we log the variable str
using the console.log()
. The code is as follows.
var item = {};
item.id = 1;
item.name = 'icy-cream';
item.flavor = 'vanilla';
var str = '';
for (const [p, val] of Object.entries(item)) {
str += `${p}:${val}\n`;
}
console.log(
'Item: ' +
'{' + str + '}');
Output:
Item: {id:1
name:icy-cream
flavor:vanilla
}
Convert JavaScript Object to a JSON String Using Object.keys()
We can use the javascript inbuilt function Object.keys()
for manually converting the JavaScript object to a JSON string. The Object.keys()
returns an array containing all the keys of a JavaScript object. We can then iterate over them using a for
loop and form the string version of the JavaScript object. The final result can be logged or stored in a variable. The code is as follows.
var item = {};
item.id = 1;
item.name = 'icy-cream';
item.flavor = 'vanilla';
var str = '';
for (key of Object.keys(item)) {
str += `${key}:${item[key]}\n`;
}
console.log(
'Item: ' +
'{' + str + '}');
Output:
Item: {id:1
name:icy-cream
flavor:vanilla
}
The concept is similar to that of using the Object.enteries()
to get the parameters of the javascript object. Object.enteries()
returns the key-value pairs as an array, whereas the Object.keys
returns the string array of object keys which are further processed by looping over the output arrays and forming the string representation of the javascript object.
Related Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn