How to Convert JavaScript Object to JSON
-
Viewing JavaScript Object With
console.log(jsObject)
-
Using the
JSON.stringify()
to Convert JSON Object to a String - Using Custom-Written Code to Convert JavaScript Objects to JSON
-
Getting a JavaScript Object Back From a
JSON
String
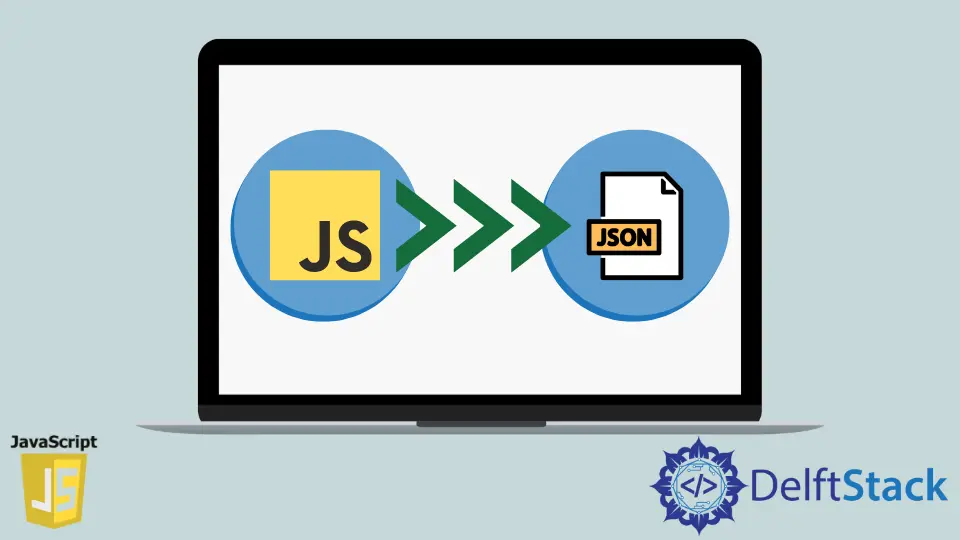
JSON is a commonly used data transfer format for representing objects in javascript. We use JSON format as a standard format in most client-server communications for transferring data. The JSON notation is easy to use and interpret as it is a human-readable format of a JavaScript object. It is easy to convert a javascript object to a JSON format. We can convert it in the following ways.
Viewing JavaScript Object With console.log(jsObject)
The console.log
function is built to output messages to the web console. We can view the messages by using the developer tools of a web browser. The conosle.log()
function accepts an object, message, and even substrings. Hence, it is best suited to help us understand the look of a JavaScript object. When we console.log
a variable holding an object, we get its visual JSON representation. The following code depicts the representation of a javascript object using the console.log
.
var a = {};
a.id = 1;
a.name = 'icy-cream';
a.flavor = 'vanilla';
console.log(a);
Output:
{id: 1, name: "icy-cream", flavor: "vanilla"}
In the above code, console.log(a)
outputs the JSON format of object a
. We will receive the same JSON representation across all browsers for the javascript object as the conosle.log()
is supported on all browsers, including the unfriendly internet explorer. Through the console.log()
option, we can only view the JSON representation of an object and may not edit it or use it for other programmatic data handling other than just seeing it in the web console.
Using the JSON.stringify()
to Convert JSON Object to a String
Another way to get the JSON representation for a javascript object is by using the JSON.stringify()
method. We can use a JavaScript object’s JSON
format and assign it to variables using the JSON.stringify()
method. JSON.stringify()
converts the javascript object and returns the JSON value for the object as string data.
Syntax
JSON.stringify(<JSObject>)
Parameters
The function takes the JavaScript object as a parameter, accepts a replacer
function, and space count
as optional parameters.
- We give our target JavaScript object to convert to JSON as the first parameter to the
JSON.stringify()
function. - We use the
replacer
function to alter the JSON object. Using it, we can specify the attributes we wish to filter out from the object before converting it into aJSON
format. - The
space count
parameter is a numeric or a string value specifying the number of space characters to be put in the output JSON string to make it in a human-readable format.
Return Value
JSON.stringify()
returns the string JSON format of the JavaScript object.
Usage
Let us use the same object that we used in the previous section. But this time, we will use the JSON.stringify()
to convert it into a JSON string. Refer to the following code.
var a = {};
a.id = 1;
a.name = 'icy-cream';
a.flavor = 'vanilla';
console.log(JSON.stringify(a));
console.log(JSON.stringify(a, null, 0));
console.log(JSON.stringify(a, null, 5));
Output:
{"id":1,"name":"icy-cream","flavor":"vanilla"}
{"id":1,"name":"icy-cream","flavor":"vanilla"}
{
"id": 1,
"name": "icy-cream",
"flavor": "vanilla"
}
Using a number in the third parameter to the JSON.stringify()
function, we get a better readable JSON
string as an output. The method will format the JSON
by adding the specified number of spaces before each key-value pair of the JSON. For instance, in JSON.stringify(a, null, 5)
, the id
parameter of the object a
is placed after five space characters.
Using Custom-Written Code to Convert JavaScript Objects to JSON
If we are to write our code for converting the JavaScript object to a JSON format, we will have to use the Object.keys()
function. Object.keys()
is a javascript method that extracts the keys
of an object and returns an array containing the keys
. Hence, with our custom code, we combine the keys
and the values
of the javascript object and encompass them within the {}
curly braces to get the JSON representation of the javascript object. Let us look at the code below.
var a = {};
a.id = 1;
a.name = 'icy-cream';
a.flavor = 'vanilla';
var keys = Object.keys(a);
var JSONOut = '{';
for (let i = 0; i < keys.length; i++) {
JSONOut = JSONOut + `"${keys[i]}":"${a[keys[i]]}",`;
}
JSONOut = JSONOut + '}';
console.log(JSONOut);
Output:
{"id":"1","name":"icy-cream","flavor":"vanilla",}
Getting a JavaScript Object Back From a JSON
String
In most web applications, we store the javascript objects as a JSON string into the database so that it is easy to use them to render the same UI back later. We may store the JSON in the database as a JSON
string. How do we get the JSON back or the JavaScript object corresponding to the JSON string pulled from the database using a REST API? JavaScript has the JSON.parse()
method for converting the JSON back to a JavaScript object. Refer to the following code.
var response = `{
"id": 1,
"name": "icy-cream",
"flavor": "vanilla"
}`
console.log(JSON.parse(response));
var a = JSON.parse(response);
a.id = 20;
console.log(a);
Output:
{id: 1, name: "icy-cream", flavor: "vanilla"}
{id: 20, name: "icy-cream", flavor: "vanilla"}
Using the above code, we converted the JSON string back to a javascript object by the JSON.parse()
method and assigned it to a variable. Changing the value of the id
attribute of the same javascript object also changes the attribute value. Hence, we can convert the string JSON to a valid javascript object which we can handle programmatically in the code. Note that we can use the tilde character to accept a string with multiple line breaks.
Related Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn