How to Find Index of Object in JavaScript Array
-
Use
findIndex()
Method to Find the Index of the Object in an Array in JavaScript -
Use
lodash
Library to Find Index of Object in JavaScript Array
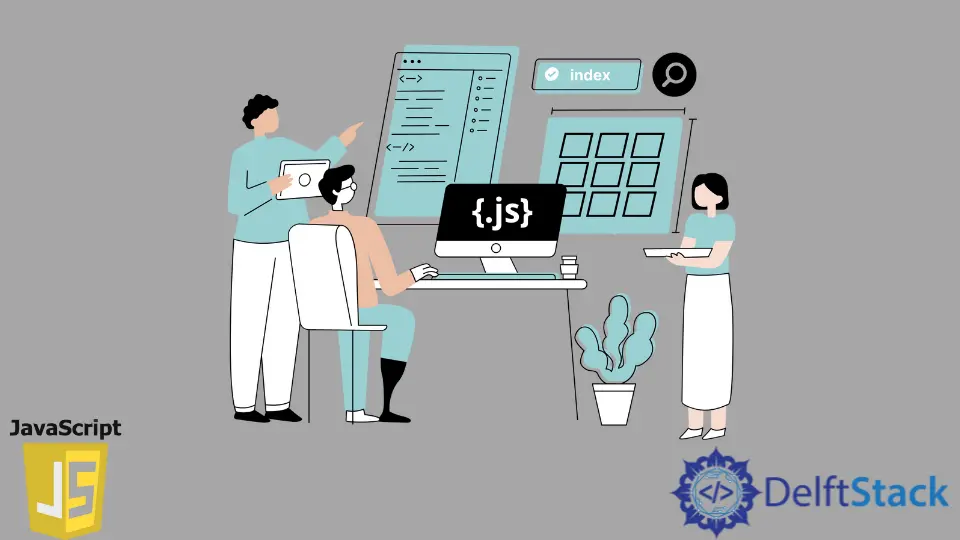
This article will explore the findIndex()
method and the use of lodash
to find the object’s index inside JavaScript Array.
Use findIndex()
Method to Find the Index of the Object in an Array in JavaScript
ES6 added a new method called findIndex()
to the Array.prototype
, which returns the first element in an array that passes the provided test. The findIndex()
method returns the element’s index that satisfies a testing function or -1
if no element passed the test. The findIndex()
is best for arrays with non-primitive types (e.g., objects) to find the index of an item.
We provide a callback as the first parameter in the Array.prototype.findIndex()
. This method is useful if the index of the arrays are non-primitive. We can use this method if the array index are more than just values. After returning the first element that matches the condition specified, findIndex()
stops checking the array for the remaining items in the array.
The syntax for findIndex()
is shown below.
array.findIndex(function(currentValue, index, arr))
Optionally, index
and arr
can be passed to the callback function. The index
option points to the current element’s index in the array, whereas arr
is the array object the current element belongs to. The findIndex()
returns -1
if the condition specified in the array does not match.
Suppose we have the following array with primitive types, and we would like to find the index of the item that has an age over 18. Then, we can use findIndex()
to find the index of the first item that matches the specified condition.
Example Code:
const ages = [3, 10, 17, 23, 52, 20];
let index = ages.findIndex(age => age > 18);
console.log(index);
Output:
3
Since the first item that matches the specified condition has an index of 3 as it is above 18, we get the value of the index as 3.
Now we can use the same logic to find the index of object that matches the condition we specify with the findIndex()
method. We can use findIndex()
to find the index of a person who has obtained a B grade.
Example Code:
const Result =
[
{
name: 'John',
grade: 'A',
},
{
name: 'Ben',
grade: 'C',
},
{
name: 'Anthony',
grade: 'B',
},
{
name: 'Tim',
grade: 'B-',
},
]
const index = Result.findIndex((element) => element.grade === 'B');
console.log(index)
Output:
2
Here, the findIndex()
is used with the Result
array, which holds our data in the form of JavaScript objects. We have implemented the arrow function, which was also introduced with ES6 as a call-back function with the findIndex()
method. We have passed element
to the call back function, which holds the object’s current value in the array we loop through.
Use lodash
Library to Find Index of Object in JavaScript Array
We can use the lightweight library lodash
, making JavaScript easier by taking the hassle out of working with arrays, numbers, objects, strings, and more. We can download the lodash.js
file from the official website and load it on top of our website, as shown below.
<script src="lodash.js"> </script>
We can install it locally as npm i --save lodash
or yarn add lodash
. With everything all set up, we can use the _.findIndex()
method. The syntax is shown below.
_.findIndex(array, [predicate = _.identity], [fromIndex = 0])
Here, arguments array
indicate the array we need to process. The option [predicate=_.identity]
is the function invoked per iteration. The third option [fromIndex=0]
is optional and can be used to set the starting point to start the iteration.
We use the array created in the method above for the demonstration of the lodash
method.
Code Example:
var index = _.findIndex(Result, {grade: 'B'})
console.log(index);
Output:
2
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript