JavaScript Array.findIndex() Method
-
Syntax of JavaScript
array.findIndex()
: -
Example Code: Use the
array.findIndex()
Method to Get the First Element With a Value Over 20 -
Example Code: Use the
array.findIndex()
Method to Find the First Index With an Even Number of Less Than 10
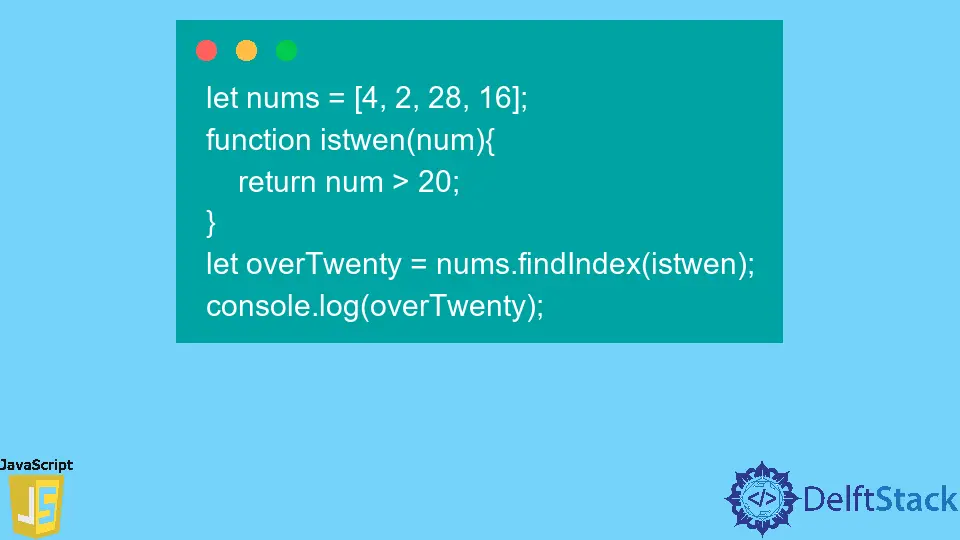
In JavaScript, the array.findIndex()
method runs the specified function and returns the first index from the given array that matches the condition.
Syntax of JavaScript array.findIndex()
:
refarr.findIndex(function(currentValue));
refarr.findIndex(function(currentValue, index, arr), this);
Parameters
callback function() |
To specify a condition and run the function for each element. |
currentValue |
The current value is required to find the first index that matches the given condition. |
index |
The index of the element is optional. |
arr |
The element’s array is optional. |
this |
This optional parameter is used in the callback function to invoke the array object. |
Return
This method returns the first index matching the condition specified in the function.
Example Code: Use the array.findIndex()
Method to Get the First Element With a Value Over 20
In JavaScript, we can use the array.findIndex()
method to get the first element from the array that matches a particular condition. We need to use a function and the current value as parameters in the array.findIndex()
method.
If an element matches the condition, the function will return that element’s index position as the output. In this example, we used the array.findIndex()
method to get the first index with a value over 20.
let nums = [4, 2, 28, 16];
function istwen(num){
return num > 20;
}
let overTwenty = nums.findIndex(istwen);
console.log(overTwenty);
Output:
2
Example Code: Use the array.findIndex()
Method to Find the First Index With an Even Number of Less Than 10
We can set different conditions in the function using the array.findIndex()
method to find an even number in the array.
In the example, we created an array with different numbers. To find the first element in the array that matches the condition, we will use all the required parameters in the array.findIndex()
method.
const nums = [1,15,20,6];
const findindex = nums.findIndex(lessEven);
function lessEven(value, index, arr){
return value < 10 && value % 2 == 0;
}
console.log(findindex);
Output:
3
The array.findIndex()
method finds the first element that matches all the conditions specified in its function.