How to Convert Double to Int in Java
- How to Convert a Double to an Int in Java Using Type Casting
-
How to Convert a Double to an Int in Java Using the
round()
Method -
How to Convert a Double to an Int in Java Using the
intValue()
Method - Conclusion
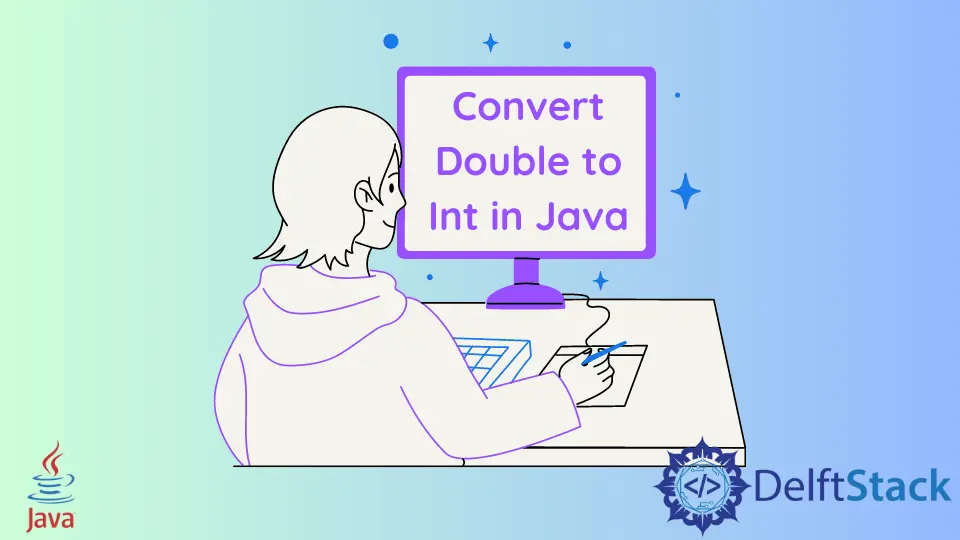
In Java programming, managing numeric data types is a fundamental aspect of developing robust and efficient applications. One common task developers encounter is the conversion of double
values to int
.
Whether it’s for handling monetary values, user input, or any scenario requiring whole numbers, having a solid understanding of the available conversion methods is crucial.
In this article, we will discuss how to cast a double
to an int
in Java using three distinct methods. Each method comes with its own set of advantages, catering to different precision and rounding requirements.
How to Convert a Double to an Int in Java Using Type Casting
One straightforward method for converting a double
data type to an int
is through type casting. Type casting is the process of converting a variable from one data type to another.
In Java, there are two types of casting: implicit casting (widening) and explicit casting (narrowing). Implicit casting is done automatically by the compiler when there is no risk of data loss, while explicit casting requires manual intervention and may result in data loss.
When converting a double
data type to an int
, you are essentially truncating the decimal part of the number. Keep in mind that this process may result in the loss of precision, as integers cannot represent decimal values. To perform this conversion, you can use explicit casting.
Syntax for Type Casting
int intValue = (int) doubleValue;
Here, intValue
is the integer variable, and doubleValue
is the double variable that needs to be converted.
Code Example
Let’s dive into a practical example:
public class DoubleToIntConversion {
public static void main(String[] args) {
double doubleValue = 123.456;
// Using type casting to convert double to int
int intValue = (int) doubleValue;
// Displaying the results
System.out.println("Original double value: " + doubleValue);
System.out.println("Converted int value: " + intValue);
}
}
In the Java program above, we start by declaring a double
variable named doubleValue
with an example value of 123.456
. The conversion to an int
is then performed using type casting:
int intValue = (int) doubleValue; // convert double to int
The (int)
syntax is the casting operation, indicating that we want to treat the doubleValue
as an integer. The result is stored in the variable intValue
.
Subsequently, we print both the original value and the converted int
value using System.out.println()
.
Code Output:
In this output, you can observe that the values after the decimal point of the original double
value are successfully truncated, and the converted int
value retains only the integer portion.
How to Convert a Double to an Int in Java Using the round()
Method
A more refined method for converting a double
to an int
involves using the round()
method from the Math
class. This method converts a floating-point value to an integer value by rounding it off to the nearest integer, providing a more nuanced conversion compared to simple type casting.
It follows the standard rounding rules: if the fractional part is 0.5 or greater, the number is rounded up; otherwise, it is rounded down. This method returns the nearest long
value of the given double, so an explicit cast to int
is required to convert it to an integer value.
The Math.round()
method is particularly useful when you want to round off the decimal part to the nearest integer.
Syntax for Using the round()
Method
int intValue = (int) Math.round(d);
Here, intValue
is the integer variable, and d
is the double variable that needs to be converted.
Code Example 1
Let’s explore this method with a practical example:
public class DoubleToIntConversion {
public static void main(String[] args) {
double d = 123.456;
// Using the round() method to convert double to int
int intValue = (int) Math.round(d); // returns long value
// Displaying the results
System.out.println("Original double value: " + d);
System.out.println("Converted int value using round(): " + intValue);
}
}
In this Java program, we start with the declaration of a double
variable named d
with the example value 123.456
. The conversion to an int
using the round()
method is then performed:
int intValue = (int) Math.round(d); // convert double to int
In this line, Math.round(d)
returns the rounded value of d
, and the result is cast to an integer. The converted value is stored in the variable intValue
.
The subsequent System.out.println()
statements display both the original double
value and the converted int
value.
Code Output:
This output demonstrates that the Math.round()
method has rounded the 123.456
value to the nearest integer 123
.
One advantage of using the round()
method is that it helps mitigate precision loss compared to simple type casting. The rounding process takes into account the fractional part of the double
value, providing a more accurate representation when converting to an integer.
Code Example 2
public class PrecisionInRoundingExample {
public static void main(String[] args) {
double d = 9.99;
// Java program using the Math.round() method to convert double to int
int intValue = (int) Math.round(d);
System.out.println("Original double value: " + d);
System.out.println("Converted int value using round(): " + intValue);
}
}
Code Output:
In this example, the Math.round()
method rounds 9.99
to 10
, providing a more accurate representation of the intended conversion.
How to Convert a Double to an Int in Java Using the intValue()
Method
In Java, the Double
wrapper class provides a convenient method called intValue()
for converting a double
value to an int
. This built-in method simplifies the conversion process by directly removing the digits after the decimal point and returning the integer part of the given double
number.
It’s an efficient way to obtain the integer representation of a floating-point number. This method is particularly useful when the precision loss is not a concern and you want a straightforward conversion.
Syntax for Using the intValue()
Method
int intValue = doubleValue.intValue();
Here, intValue
is the integer variable, and doubleValue
is the Double
object or double variable that needs to be converted.
Code Example
Let’s explore the usage of the intValue()
method with a practical example:
public class DoubleToIntConversion {
public static void main(String[] args) {
Double doubleValue = 123.456; // using Double wrapper class
// Using the intValue() method to convert Double to int
int intValue = doubleValue.intValue();
// Displaying the results
System.out.println("Original double value: " + doubleValue);
System.out.println("Converted int value using intValue(): " + intValue);
}
}
In this Java program, we declare a Double
object named doubleValue
with the example value 123.456
. The conversion to an int
using the intValue()
method is then performed:
int intValue = doubleValue.intValue(); // convert double to int
The intValue()
method is called on the Double
object, and it directly returns the integer part of the doubleValue
. The converted value is stored in the variable intValue
.
The subsequent System.out.println()
statements display both the original double
value and the converted int
value.
Code Output:
This output illustrates that the intValue()
method of the Double
class effectively extracts the integer part of the original double
value 123.456
.
It’s important to note that using the intValue()
method directly truncates the decimal part of the double
value without rounding. This means that if the double
value has a fractional part, it will be discarded during the conversion.
Conclusion
In this article, we explored three distinct approaches: type casting, the use of the round()
method, and the convenient intValue()
method provided by the Double
class.
Type casting stands out as the most direct method, truncating the decimal part and retaining the integer portion. For scenarios where precision in rounding is crucial, the round()
method from the Math
class becomes a valuable tool, ensuring accurate conversion to the nearest integer.
On the other hand, the intValue()
method from the Double
class simplifies the process with an object-oriented approach, directly returning the integer part of the double
value.
Ultimately, the choice of method depends on the specific requirements of your application. Whether you prioritize simplicity, precision, or an object-oriented approach, understanding these techniques empowers you to make informed decisions when converting double
to int
in your Java programs.
To further improve your Java coding skills, the next relevant topic you can explore is converting a hexadecimal string to an int
in Java.
Related Article - Java Double
- How to Convert Int to Double in Java
- Double in Java
- How to Compare Doubles in Java
- Float and Double Data Type in Java
- How to Convert Double to String in Java
- How to Convert Double to Float in Java