How to Convert Hex String to Int in Java
-
Java Convert a Short Hex String to
int
UsingInteger.decode()
-
Java Convert a Long Hex String to
int
UsingLong.parseLong()
-
Java Convert a Very Long Hex String to
int
UsingBigInteger
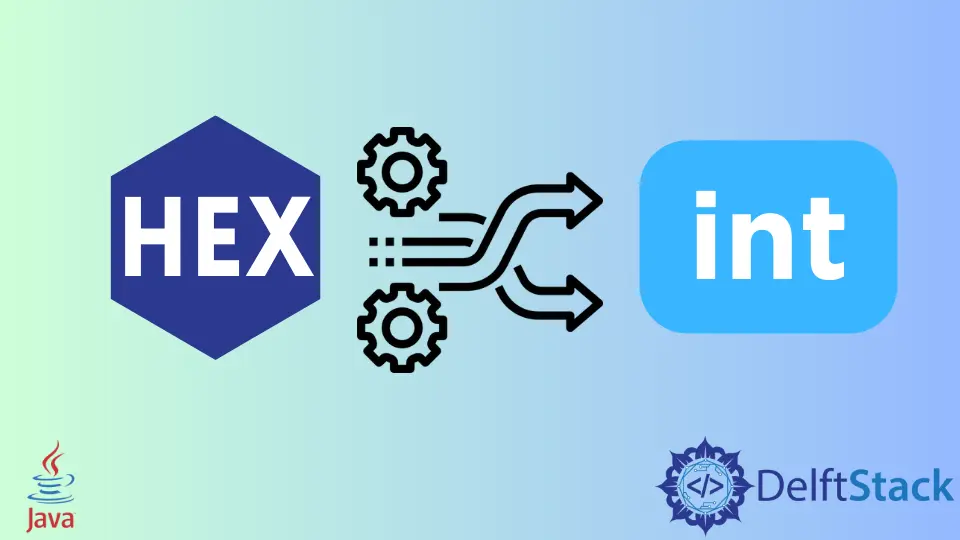
This article will introduce the three methods that we can use to convert a hex string into an int
. Hex or hexadecimal is a 16-base numbering system that means that there are 16 possible symbols representing numbers. The hex values used to represent numbers are 0-9 in decimal and A-F representing 10-15 in the decimal system.
In the following examples, we will have a look at the steps to convert a given hexadecimal string of different lengths to an int
in Java.
Java Convert a Short Hex String to int
Using Integer.decode()
In the first example, we are using the decode()
function of the Integer
class that takes a String
and then returns a primitive int
.
In Java, an int
can store the minimum value of -231 to the maximum value of 231-1. If we want our result in the int
type, we cannot work on long strings. Further examples in this article will show how we can convert longer hexadecimal values into a numeric value.
public class Main {
public static void main(String[] args) {
String hex = "0x2fd";
int convertedValue = Integer.decode(hex);
System.out.print(convertedValue);
}
}
Output:
765
Java Convert a Long Hex String to int
Using Long.parseLong()
As we discussed above, we cannot convert large hex values to primitive int
because it can return value out of an int
capacity. This is why in this example we are using Long
that has a larger capacity of -263 to 263-1.
The parseLong()
method of Long
takes two parameters, a string and a base value, to parse the string to a primitive long integer.
public class Main {
public static void main(String[] args) {
String hex = "AA0F245C";
long l = Long.parseLong(hex, 16);
System.out.println(l);
}
}
Output:
2853119068
Java Convert a Very Long Hex String to int
Using BigInteger
Hexadecimal values can be used for various purposes, and one of them is when we want to encrypt something, this hex value can be very long. If we want to convert this hex to a numeric value for some reason, then we can use BigInteger
, which can hold large integers, as its name indicates.
In the following example, we pass our hex value to the constructor of BigInteger
with the base value. We can also get the int
value from BigInteger.intValue()
, but it will only return the starting 32 bits.
import java.math.BigInteger;
public class Main {
public static void main(String[] args) {
String hex = "4D21C5BA77D06212F39E16BF2756E9811125F7FC";
BigInteger bigInteger = new BigInteger(hex, 16);
System.out.println(bigInteger);
}
}
Output:
440345438517096076415390637616379300179635206140
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Int
- How to Convert Int to Char in Java
- How to Convert Int to Double in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java
- How to Convert Int to Byte in Java