How to Convert String to Hex in Java
-
Convert String to Hex by Using Array of
char
andInteger.toHexString()
-
Convert String to Hex Using an Array of
byte
and String Formatter - Convert String to Hex in Java Using Apache Commons Codec
-
Convert String to Hex in Java Using
BigInteger
- Conclusion
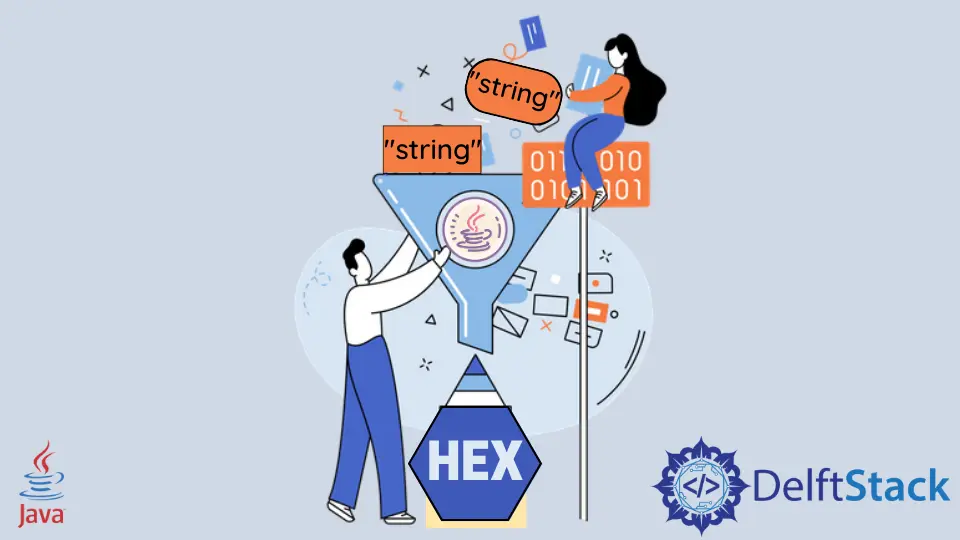
In programming, there are various scenarios where you might need to convert a string into its hexadecimal representation. This task is particularly important in fields like data encoding, cryptography, and data storage.
Java, being a versatile language, provides multiple methods to achieve this goal. In this article, we will explore four distinct approaches to converting a string into its hexadecimal form in Java.
Convert String to Hex by Using Array of char
and Integer.toHexString()
The Integer.toHexString()
method in Java is a straightforward way to convert integers to their hexadecimal string representation. You can pass an integer value to this method, and it will return a string representing the hexadecimal value of that integer.
However, converting a string to hexadecimal requires working with individual characters in the string.
Here’s the basic idea of how we can convert a string to hexadecimal using Integer.toHexString()
:
-
Convert the provided string into an array of characters (
char[]
). -
Iterate through each character in the array.
-
For each character, convert it into its corresponding hexadecimal representation using
Integer.toHexString()
.
Let’s break down the process into code snippets and explanations:
public static String stringToHex(String input) {
char[] characters = input.toCharArray();
StringBuilder hexString = new StringBuilder();
for (char c : characters) {
int intValue = (int) c;
String hexValue = Integer.toHexString(intValue);
hexString.append(hexValue);
}
return hexString.toString();
}
Now, let’s discuss each step in detail.
First, the toCharArray()
method is used to convert the input string into an array of characters. This step is necessary to process each character in the string individually.
Using (int) c
, we cast each character to an integer to obtain its Unicode code point. This step allows us to convert characters to their corresponding integer values.
Then, by using the Integer.toHexString()
method, we convert the integer value to its hexadecimal representation as a string.
Next, we append the hexadecimal value to a StringBuilder
using hexString.append(hexValue)
. Utilizing a StringBuilder
is more efficient for concatenating strings repeatedly in a loop, as it reduces memory overhead.
Finally, we convert the StringBuilder
to a string using the toString()
method and return the result.
Here’s how you can use the stringToHex
method to convert a string to its hexadecimal representation:
public class StringToHexExample {
public static String stringToHex(String input) {
char[] characters = input.toCharArray(); // Convert the input string to an array of characters
StringBuilder hexString =
new StringBuilder(); // Create a StringBuilder to store the hexadecimal representation
for (char c : characters) {
int intValue = (int) c; // Convert the character to its integer value
String hexValue =
Integer.toHexString(intValue); // Convert the integer to its hexadecimal representation
hexString.append(hexValue); // Append the hexadecimal value to the StringBuilder
}
return hexString.toString(); // Convert the StringBuilder to a string and return the result
}
public static void main(String[] args) {
String input = "Hello, World!";
String hexadecimalString = stringToHex(input);
System.out.println("Original String: " + input);
System.out.println("Hexadecimal String: " + hexadecimalString);
}
}
When you run this code, it will output:
Original String: Hello, World!
Hexadecimal String: 48656c6c6f2c20576f726c6421
As you can see, the stringToHex
method effectively converts the input string into its hexadecimal representation.
Convert String to Hex Using an Array of byte
and String Formatter
Java’s String.format()
method provides a versatile way to format and create strings. It allows you to specify a format string, which includes placeholders for various data types, such as integers and floating-point numbers.
In the context of converting a string to hexadecimal, we can use the %02X
format specifier to represent each byte as a two-character uppercase hexadecimal value.
Here’s the basic approach for converting a string to hexadecimal using String.format()
:
-
Convert the string to an array of bytes.
-
Iterate through each byte in the array.
-
Format each byte as a two-character hexadecimal value using
String.format()
.
Let’s break down the process into code snippets and explanations:
public static String stringToHex(String input) {
byte[] bytes = input.getBytes(); // Convert the input string to an array of bytes
StringBuilder hexString =
new StringBuilder(); // Create a StringBuilder to store the hexadecimal representation
for (byte b : bytes) {
hexString.append(String.format("%02X",
b)); // Format the byte as a two-character hexadecimal value and append to the StringBuilder
}
return hexString.toString(); // Convert the StringBuilder to a string and return the result
}
Now, let’s discuss each step in detail.
We convert the input string into an array of bytes using the getBytes()
method. This step is necessary to process each byte of the string individually.
Next, the %02X
format specifier within String.format()
is used to format each byte as a two-character uppercase hexadecimal value. It ensures that each hexadecimal value is represented as two characters and is in uppercase.
We then use hexString.append(...)
to append the formatted hexadecimal value to a StringBuilder
. Using a StringBuilder
is more memory-efficient when concatenating strings in a loop.
Finally, we convert the StringBuilder
to a string using the toString()
method and return the result.
Here’s how you can use the stringToHex
method to convert a string to its hexadecimal representation:
public class StringToHexExample {
public static String stringToHex(String input) {
byte[] bytes = input.getBytes();
StringBuilder hexString = new StringBuilder();
for (byte b : bytes) {
hexString.append(String.format("%02X", b));
}
return hexString.toString();
}
public static void main(String[] args) {
String input = "Hello, World!";
String hexadecimalString = stringToHex(input);
System.out.println("Original String: " + input);
System.out.println("Hexadecimal String: " + hexadecimalString);
}
}
When you run this code, it will output:
Original String: Hello, World!
Hexadecimal String: 48656C6C6F2C20576F726C6421
As you can see, the stringToHex
method effectively converts the input string into its hexadecimal representation using the %02X
format specifier and the String.format()
method.
Convert String to Hex in Java Using Apache Commons Codec
Apache Commons Codec is a part of the Apache Commons project, which provides open-source, reusable libraries for various programming tasks. The Codec library focuses on encoding and decoding data in various formats, including Base64, hexadecimal, URL encoding, and more.
It simplifies data manipulation and is widely used in Java development for encoding and decoding operations.
Before you can use Apache Commons Codec, you need to add it to your Java project. You can do this using a build tool like Apache Maven or Gradle or by manually downloading and including the library.
If you’re using Apache Maven, you can include Apache Commons Codec as a dependency in your project’s pom.xml
file:
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.15</version> <!-- Replace with the latest version -->
</dependency>
For Gradle users, add the following to your build.gradle
file:
implementation group: 'commons-codec', name: 'commons-codec', version: '1.15' // Replace with the latest version
After adding the dependency, make sure to update your project so that Gradle or Maven downloads the library.
To use Apache Commons Codec for hexadecimal encoding, you need to import the Hex
class from the library:
import org.apache.commons.codec.binary.Hex;
Now, you can create a method that takes a string as input and returns its hexadecimal representation. The core of the conversion is done by the Hex.encodeHex
method provided by Apache Commons Codec:
public static String stringToHex(String input) {
byte[] bytes = input.getBytes(); // Convert the input string to an array of bytes
char[] hexChars = Hex.encodeHex(bytes); // Encode the byte array as hexadecimal
return new String(hexChars); // Convert the char array back to a string and return the result
}
This method accepts an input string, converts it to a byte array, encodes the bytes as hexadecimal, and finally converts the hexadecimal bytes back to a string.
You can now use the stringToHex
method to convert a string to its hexadecimal representation.
Here’s a complete working example of how to use it:
import org.apache.commons.codec.binary.Hex;
public class StringToHexExample {
public static String stringToHex(String input) {
byte[] bytes = input.getBytes(); // Convert the input string to an array of bytes
char[] hexChars = Hex.encodeHex(bytes); // Encode the byte array as hexadecimal
return new String(hexChars); // Convert the char array back to a string and return the result
}
public static void main(String[] args) {
String input = "Hello, World!";
String hexadecimalString = stringToHex(input);
System.out.println("Original String: " + input);
System.out.println("Hexadecimal String: " + hexadecimalString);
}
}
When you run this code, it will output:
Original String: Hello, World!
Hexadecimal String: 48656c6c6f2c20576f726c6421
This output demonstrates the successful conversion of the input string to its hexadecimal representation using Apache Commons Codec.
Convert String to Hex in Java Using BigInteger
The BigInteger
class in Java provides a way to represent integers of arbitrary precision. This class includes a method, toString(int radix)
, which allows us to convert numbers to a specified radix, such as hexadecimal (base 16
).
Here are the steps to convert a string to its hexadecimal representation using BigInteger
:
-
Firstly, you’ll convert the string to its hexadecimal representation using the
BigInteger
class. -
To convert the string to a hexadecimal number, you’ll need to get the byte array of the string and then create a
BigInteger
object from this byte array. -
Finally, you’ll use the
toString(16)
method of theBigInteger
class to obtain the hexadecimal representation of the number.
Take a look at the example below:
import java.math.BigInteger;
public class StringToHexUsingBigInteger {
public static String stringToHex(String input) {
byte[] bytes = input.getBytes();
BigInteger bigInt = new BigInteger(1, bytes);
String hexadecimalString = bigInt.toString(16);
return hexadecimalString;
}
public static void main(String[] args) {
String input = "Hello, World!";
String hexadecimalString = stringToHex(input);
System.out.println("Original String: " + input);
System.out.println("Hexadecimal String: " + hexadecimalString);
}
}
Here’s how the code works:
The stringToHex
method takes an input string as its argument. Then, it converts the input string into a byte array using getBytes()
.
Next, it creates a BigInteger
instance named bigInt
from the byte array. The 1
passed as the first argument signifies that the byte array is positive.
Once that is done, it converts the BigInteger
to a hexadecimal string using the toString(16)
method. Finally, it returns the hexadecimal string.
The main
method is the entry point of the program. It defines the input string as Hello, World!
(you can change this string to any text you want to convert).
Then, it calls the stringToHex
method to obtain the hexadecimal representation of the input string. Lastly, it displays both the original string and its hexadecimal representation in the console using System.out.println
.
When you run the provided Java code, it will output:
Original String: Hello, World!
Hexadecimal String: 48656c6c6f2c20576f726c6421
This output demonstrates the successful conversion of the input string to its hexadecimal representation using the BigInteger
class in Java.
Conclusion
Converting a string to its hexadecimal representation is a common operation in Java, and it’s important to understand the various methods available to achieve this task. Depending on your specific use case and requirements, you can choose the approach that best suits your needs.
Whether you opt for the simplicity of using arrays of characters, the versatility of BigInteger
, the efficiency of byte
arrays with a StringBuilder
, or the convenience of Apache Commons Codec, you’ll be well-equipped to handle this essential operation in your Java projects.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java