How to Convert Hex to String in Java
-
Convert Hex to String in Java Using
Integer.parseInt
andCharacter.toChars
-
Convert Hex to String in Java Using
BigInteger
Class - Convert Hex to String in Java Using Apache Commons Codec
- Conclusion
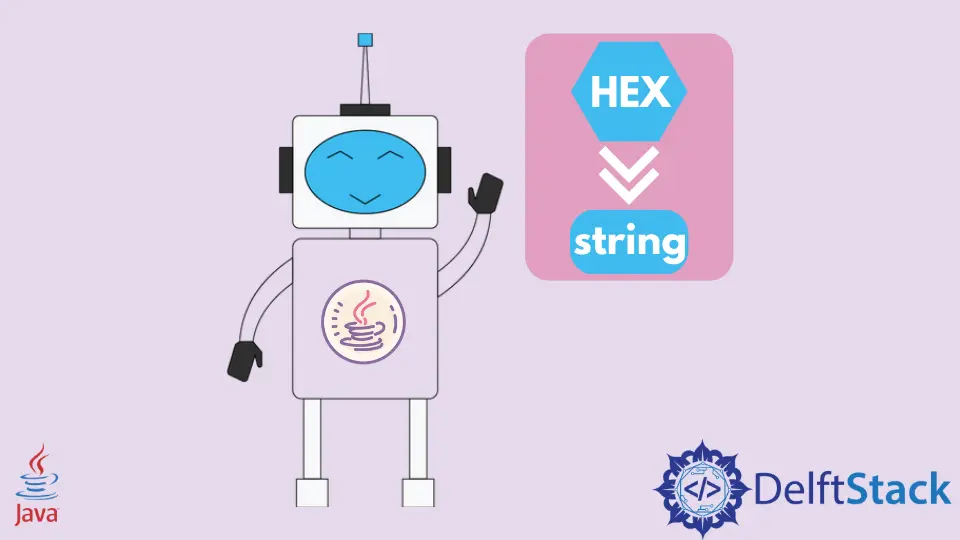
Hexadecimal representation is a common format for expressing binary data, and converting hexadecimal strings to regular text strings is a frequent task in Java programming.
In this article, we’ll explore different approaches to achieve this conversion, providing insights into the underlying concepts and considerations.
Convert Hex to String in Java Using Integer.parseInt
and Character.toChars
One approach to convert a hexadecimal string to a human-readable string involves using the Integer.parseInt
method to convert the hex string to an integer and then utilizing the Character.toChars
method to transform the integer into a corresponding Unicode character.
Let’s break down the process of converting a hex string to a string using Integer.parseInt
and Character.toChars
in Java:
-
Start by obtaining the hex string that you want to convert. This string should consist of valid hexadecimal characters (
0-9
,A-F
, ora-f
).
String hexString =
"48656c6c6f2120546869732069732064656c6674737461636b2e636f6d"; // Example hex string
-
Next, split the hex string into pairs of characters using a regular expression.
String[] hexPairs = hexString.split("(?<=\\G.{2})");
-
Iterate through each pair of hexadecimal characters, convert them to integers using
Integer.parseInt
, and then convert each integer to its corresponding Unicode character usingCharacter.toChars
. Append each character to aStringBuilder
.
StringBuilder resultBuilder = new StringBuilder();
for (String hexPair : hexPairs) {
int decimalValue = Integer.parseInt(hexPair, 16);
resultBuilder.append(Character.toChars(decimalValue));
}
-
Finally, construct a string from the
StringBuilder
using thetoString
method.
String resultString = resultBuilder.toString();
Here’s the complete Java code for the conversion process:
public class HexToStringConverter {
public static void main(String[] args) {
String hexString = "48656c6c6f2120546869732069732064656c6674737461636b2e636f6d";
String[] hexPairs = hexString.split("(?<=\\G.{2})");
StringBuilder resultBuilder = new StringBuilder();
for (String hexPair : hexPairs) {
int decimalValue = Integer.parseInt(hexPair, 16);
resultBuilder.append(Character.toChars(decimalValue));
}
String resultString = resultBuilder.toString();
System.out.println("Before the conversion: " + hexString);
System.out.println("After the conversion: " + resultString);
}
}
Output:
Before the conversion: 48656c6c6f2120546869732069732064656c6674737461636b2e636f6d
After the conversion: Hello! This is delftstack.com
As you can see, converting a hex string to a string in Java using Integer.parseInt
and Character.toChars
involves breaking the hex string into pairs, converting each pair to integers, and then converting each integer to its corresponding Unicode character. This approach allows for an accurate conversion of hex values to human-readable strings.
Note that you need to handle exceptions appropriately, such as NumberFormatException
when parsing the hex string, to create a robust solution for your application.
Convert Hex to String in Java Using BigInteger
Class
Converting a hexadecimal string to a human-readable string in Java can be achieved using the versatile BigInteger
class. This approach is particularly useful when dealing with large hexadecimal values.
Let’s explore the step-by-step process of converting a hex string to a string using the BigInteger
class in Java:
-
Like the previous section, begin by obtaining the hex string that you intend to convert. Ensure that the hex string consists of valid hexadecimal characters.
String hexString =
"48656c6c6f2120546869732069732064656c6674737461636b2e636f6d"; // Example hex string
-
Then, use the
BigInteger
class to create aBigInteger
object from the hex string. Specify 16 as the radix to indicate that the input is in hexadecimal.
BigInteger bigInteger = new BigInteger(hexString, 16);
-
Convert the
BigInteger
object to a string using thetoString
method.
String resultString = new String(bigInteger.toByteArray());
Here’s the complete Java code for the conversion process:
import java.math.BigInteger;
public class HexToStringConverter {
public static void main(String[] args) {
String hexString = "48656c6c6f2120546869732069732064656c6674737461636b2e636f6d";
BigInteger bigInteger = new BigInteger(hexString, 16);
String resultString = new String(bigInteger.toByteArray());
System.out.println("Before the conversion: " + hexString);
System.out.println("After the conversion: " + resultString);
}
}
Output:
Before the conversion: 48656c6c6f2120546869732069732064656c6674737461636b2e636f6d
After the conversion: Hello! This is delftstack.com
By following this method, you can effortlessly convert hex values to human-readable strings, especially when working with substantial hexadecimal inputs.
Convert Hex to String in Java Using Apache Commons Codec
Converting a hexadecimal string to a human-readable string in Java can be efficiently achieved using the Apache Commons Codec library.
Apache Commons Codec is a library that provides implementations of common encoders and decoders. The library is part of the Apache Commons project and aims to provide robust, efficient, and reusable code for encoding and decoding various data formats.
Before using Apache Commons Codec for hex-to-string conversion, you need to include the library in your project. You can download the library from the Apache Commons website (https://commons.apache.org/proper/commons-codec/) or use a build tool like Maven or Gradle to manage dependencies.
If you are using Maven, add the following dependency to your pom.xml
:
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>1.15</version> <!-- Use the latest version available -->
</dependency>
For Gradle, include the following in your build.gradle
file:
implementation 'commons-codec:commons-codec:1.15' // Use the latest version available
Now, let’s explore how to use Apache Commons Codec to convert hexadecimal strings to regular strings in Java.
-
Similar to the previous examples, begin by obtaining the hex string that you intend to convert. Ensure that the hexadecimal string is valid.
String hexString =
"48656c6c6f2120546869732069732064656c6674737461636b2e636f6d"; // Example hex string
-
Then, use the
decodeHex
method from theHex
class in the Apache Commons Codec library to decode the hex string into a byte array.
byte[] byteArray = org.apache.commons.codec.binary.Hex.decodeHex(hexString.toCharArray());
-
Convert the resulting byte array to a string using the
String
class constructor.
String resultString = new String(byteArray);
Here’s the complete Java code for the conversion process:
import org.apache.commons.codec.DecoderException;
import org.apache.commons.codec.binary.Hex;
public class HexToStringConverter {
public static void main(String[] args) {
String hexString = "48656c6c6f2120546869732069732064656c6674737461636b2e636f6d";
try {
byte[] byteArray = Hex.decodeHex(hexString.toCharArray());
String resultString = new String(byteArray);
System.out.println("Before the conversion: " + hexString);
System.out.println("After the conversion: " + resultString);
} catch (DecoderException e) {
System.err.println("Error decoding hex string: " + e.getMessage());
}
}
}
Output:
Before the conversion: 48656c6c6f2120546869732069732064656c6674737461636b2e636f6d
After the conversion: Hello! This is delftstack.com
In this code, we added a try-catch
block around the Hex.decodeHex
method to catch the potential DecoderException
. If an exception occurs during the decoding process, it will print an error message.
You can customize the exception-handling logic based on your application’s requirements. When using the Apache Commons Codec library, it is important to be sure that you include the Apache Commons Codec library in your project.
Conclusion
Converting hexadecimal strings to regular strings in Java involves choosing an appropriate method based on your specific requirements and the Java version you are using. Whether you opt for built-in functions or external libraries like Apache Commons Codec, understanding the underlying concepts and considerations will help you make informed decisions for your coding tasks.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook