How to Cast Variables in Java
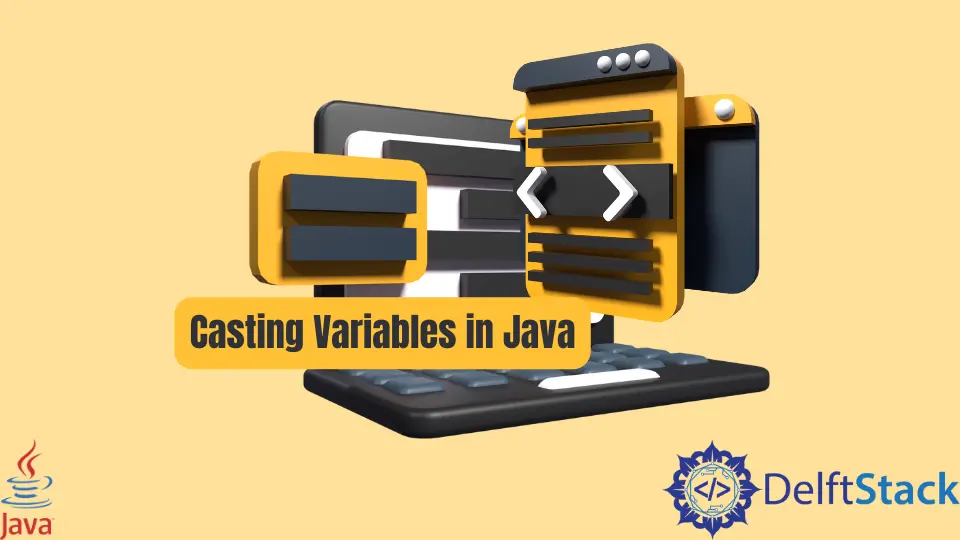
This tutorial introduces how to cast variables or convert a variable to another type in Java.
Casting is used to cast/convert one value/variable to another type in a programming language. Java supports a rich set of data types such as int
, float
, double
, boolean
, etc., and during writing code, it may be required to cast variables.
Java supports two types of casting, implicit and explicit casting. If we cast int
into long
or double
, then Java does this implicitly because the long
and double
use more bytes than int
, and we do its reverse (i.e., convert double
to int
) then it may lead to data loss due to integer capacity.
So, Java allows implicit casting between lower to higher data types but explicit casting for higher to lower types. Let’s understand with some examples.
Implicit Casting in Java
Here, in this example, we cast int
to float
type, implicit casting. Since Java allows implicit casting without much code, this code works fine.
public class SimpleTesting {
public static void main(String[] args) {
int a = 23;
System.out.println("int " + a);
// int to float - implicit casting
float f = a;
System.out.println("float " + f);
}
}
Output:
int 23
float 23.0
Explicit Casting in Java
In some cases, Java does require explicit casting because of data loss.
For example, if we cast a float
to int
, then the value after the decimal point gets truncated; that’s why Java does not do this implicitly and throws a compile-time error. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
float a = 23;
System.out.println("float " + a);
// float to int - explicit casting
int f = a;
System.out.println("int " + f);
}
}
Output:
Type mismatch: cannot convert from float to int
This compile-time error is a warning to the programmer to avoid this data loss. If the programmer still wants to cast, Java allows a cast operator that encloses the type name in function parenthesis.
This compiler compiles and executes the code but sees the value is truncated. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
float a = 23.5f;
System.out.println("float " + a);
// float to int - explicit casting
int f = (int) a;
System.out.println("int " + f);
}
}
Output:
float 23.50
int 23
We can also use the cast()
method to convert the object value to a primitive value. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Integer a = 23;
System.out.println("integer " + a);
// float to int - explicit casting
int i = (Integer.class.cast(a));
System.out.println("int " + i);
}
}
Output:
integer 23
int 23
Avoid CastException
in Java
Casting Java objects is also a major concern that requires proper class type before casting. For example, if we cast a float
type with double
, Java does not allow this and throws ClassCastException
to the console.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
Number num = new Float(15.5);
System.out.println(num);
Double d = (Double) num;
System.out.println(d);
}
}
Output:
15.5
Exception in thread "main" java.lang.ClassCastException