How to Convert Char Array to Int in Java
-
Convert Char Array to Int in Java Using
Integer.parseInt()
-
Convert Char Array to Int in Java Using
String.valueOf()
andInteger.parseInt()
-
Convert Char Array to Int in Java Using
StringBuilder
andInteger.parseInt()
- Convert Char Array to Int in Java Using a Loop
-
Convert Char Array to Int in Java Using
CharArrayReader
andStreamTokenizer
- Conclusion
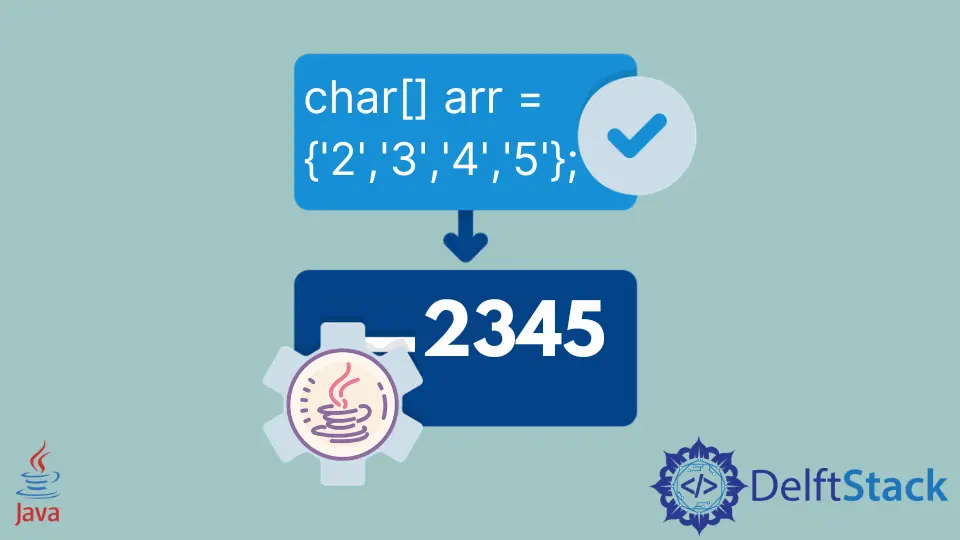
Converting a character array (char[]
) to an integer (int
) is a common operation in Java, especially when dealing with input from various sources.
In this article, we will explore different methods to achieve this conversion, covering various approaches to suit different scenarios.
Convert Char Array to Int in Java Using Integer.parseInt()
One straightforward and commonly used approach to convert a char array to an int involves the use of the Integer.parseInt()
method. This method is part of the Integer
class in Java and is designed to parse a string argument as a signed decimal integer.
It takes a string representation of an integer as input and returns the corresponding int
value. This method is particularly useful when dealing with numeric input that is represented as a string.
Let’s dive into the process of converting a char array to an int using Integer.parseInt()
:
-
First, you need a character array that contains the numeric characters you want to convert to an integer.
For example:
char[] charArray = {'1', '2', '3', '4', '5'};
-
Then, use the
String
constructor to create a string representation of the character array:String str = new String(charArray);
-
Next, apply the
Integer.parseInt()
method to parse the string and obtain the integer result:int result = Integer.parseInt(str);
Here’s the complete code combining the above steps:
public class CharArrayToIntExample {
public static void main(String[] args) {
char[] charArray = {'1', '2', '3', '4', '5'};
String str = new String(charArray);
int result = Integer.parseInt(str);
System.out.println("Converted int value: " + result);
}
}
Output:
Converted int value: 12345
It’s important to note that Integer.parseInt()
may throw a NumberFormatException
if the string representation is not a valid integer. Therefore, it’s recommended to handle this exception appropriately in your code.
You can use a try-catch
block to manage potential errors:
try {
int result = Integer.parseInt(str);
System.out.println("Converted int value: " + result);
} catch (NumberFormatException e) {
System.err.println("Error: Invalid integer format");
e.printStackTrace();
}
Convert Char Array to Int in Java Using String.valueOf()
and Integer.parseInt()
An alternative approach involves using the String.valueOf()
method. This method is a versatile utility in Java that converts different types, including characters and arrays, into their string representations.
When applied to a char array, it creates a string containing the characters of the array.
Let’s walk through how to convert a char array to an int using String.valueOf()
and Integer.parseInt()
:
-
Begin by creating a character array containing the numeric characters you wish to convert.
For instance:
char[] charArray = {'1', '2', '3', '4', '5'};
-
Apply
String.valueOf()
to convert the char array to a string:String str = String.valueOf(charArray);
-
Now, utilize
Integer.parseInt()
to parse the string and obtain the integer result:int result = Integer.parseInt(str);
Here’s the result when you combine the above steps into a complete Java program:
public class CharArrayToIntExample {
public static void main(String[] args) {
char[] charArray = {'5', '6', '7', '8', '9'};
String str = String.valueOf(charArray);
int result = Integer.parseInt(str);
System.out.println("Converted int value: " + result);
}
}
Output:
Converted int value: 56789
As with any conversion operation, it’s crucial to consider potential exceptions. In this case, Integer.parseInt()
may throw a NumberFormatException
if the string representation is not a valid integer.
To handle this, you can use a try-catch block:
try {
int result = Integer.parseInt(str);
System.out.println("Converted int value: " + result);
} catch (NumberFormatException e) {
System.err.println("Error: Invalid integer format");
e.printStackTrace();
}
Using String.valueOf()
and Integer.parseInt()
provides a concise and effective method for converting a char array to an int in Java. This approach leverages the versatility of String.valueOf()
to transform the character array into a string, which is then easily parsed into an integer using Integer.parseInt()
.
As always, be mindful of potential exceptions and incorporate appropriate error-handling mechanisms to ensure the robustness of your code.
Convert Char Array to Int in Java Using StringBuilder
and Integer.parseInt()
If you prefer a more manual approach, you can use a StringBuilder
to build the string representation of the integer.
StringBuilder
is a mutable sequence of characters introduced in Java to efficiently build and manipulate strings. It provides methods for appending, inserting, and modifying character sequences, making it a powerful tool for string construction.
Let’s go through the steps to convert a char array to an int using StringBuilder
and Integer.parseInt()
:
-
Start by creating a character array with the numeric characters you want to convert.
For example:
char[] charArray = {'1', '2', '3', '4', '5'};
-
Initialize a
StringBuilder
and iterate through the char array, appending each character:StringBuilder sb = new StringBuilder(); for (char c : charArray) { sb.append(c); }
-
Now, apply
Integer.parseInt()
to parse the string obtained from theStringBuilder
and get the integer result:String str = sb.toString(); int result = Integer.parseInt(str);
Here is the complete Java program when you combine all the steps:
public class CharArrayToIntExample {
public static void main(String[] args) {
char[] charArray = {'1', '2', '3', '4', '5'};
StringBuilder sb = new StringBuilder();
for (char c : charArray) {
sb.append(c);
}
String str = sb.toString();
int result = Integer.parseInt(str);
System.out.println("Converted int value: " + result);
}
}
Output:
Converted int value: 12345
Similar to other methods, it’s important to handle potential exceptions, especially the NumberFormatException
that might be thrown by Integer.parseInt()
if the string representation is not a valid integer.
Use the same try-catch block as the previous section for proper error handling.
Convert Char Array to Int in Java Using a Loop
In this approach, a simple loop can be employed to iterate through the character array and build the integer.
The idea behind this approach is to iterate through the character array, treating each character as a digit in a numeric value. By using a loop, we can construct the integer value incrementally, avoiding the need for intermediate string conversions.
-
Begin by creating a character array containing the numeric characters you want to convert.
For example:
char[] charArray = {'1', '2', '3', '4', '5'};
-
Initialize the result to
0
and iterate through the char array, updating the result with each digit:int result = 0; for (char c : charArray) { result = result * 10 + (c - '0'); }
-
In each iteration, the result is multiplied by
10
to shift its digits to the left, and the value of the current character (converted to an integer using(c - '0')
) is added.
When you combine all these steps into a complete Java program, here’s the result:
public class CharArrayToIntExample {
public static void main(String[] args) {
char[] charArray = {'1', '2', '3', '4', '5'};
int result = 0;
for (char c : charArray) {
result = result * 10 + (c - '0');
}
System.out.println("Converted int value: " + result);
}
}
Output:
Converted int value: 12345
This loop approach is straightforward, but it assumes that the characters in the array represent a valid integer. For robustness, you might want to include additional checks or error handling, especially if the char array might contain non-numeric characters.
This method avoids the need for intermediate string representations, making it particularly useful for scenarios where direct numeric processing is required.
Convert Char Array to Int in Java Using CharArrayReader
and StreamTokenizer
For a more advanced approach, you can use CharArrayReader
and StreamTokenizer
classes.
The CharArrayReader
class is a character stream whose data originated from a character array. It provides an efficient way to read characters from a char array as if reading from a stream.
On the other hand, the StreamTokenizer
class takes an input stream and parses it into tokens based on specified rules. It can recognize various types of tokens, including numbers, words, and special characters.
Let’s see how to convert a char array to an int using CharArrayReader
and StreamTokenizer
.
-
Start by creating a character array containing the numeric characters you want to convert.
For example:
char[] charArray = {'1', '2', '3', '4', '5'};
-
Then, create a
CharArrayReader
using the char array:CharArrayReader reader = new CharArrayReader(charArray);
-
Next, create a
StreamTokenizer
using theCharArrayReader
and configure it to recognize numeric tokens:StreamTokenizer tokenizer = new StreamTokenizer(reader); tokenizer.parseNumbers();
-
Use the
nextToken()
method to read the next token and retrieve the integer value:int result = (int) tokenizer.nval;
Here’s the complete Java program when you combine these steps:
import java.io.CharArrayReader;
import java.io.IOException;
import java.io.StreamTokenizer;
public class CharArrayToIntExample {
public static void main(String[] args) {
char[] charArray = {'1', '2', '3', '4', '5'};
CharArrayReader reader = new CharArrayReader(charArray);
StreamTokenizer tokenizer = new StreamTokenizer(reader);
tokenizer.parseNumbers();
try {
tokenizer.nextToken();
int result = (int) tokenizer.nval;
System.out.println("Converted int value: " + result);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Converted int value: 12345
Since the StreamTokenizer
methods may throw IOException
, it’s essential to handle potential exceptions for a robust implementation. Hence, we used the following in the code snippet above:
try {
tokenizer.nextToken();
int result = (int) tokenizer.nval;
System.out.println("Converted int value: " + result);
} catch (IOException e) {
System.err.println("Error reading from the stream");
e.printStackTrace();
}
Using CharArrayReader
and StreamTokenizer
provides an advanced method for converting a char array to an int in Java. This approach is particularly useful when dealing with complex character data structures or when a more sophisticated tokenization is required.
While it may introduce additional complexity compared to simpler methods, it offers enhanced flexibility and functionality. As always, handle exceptions appropriately to ensure the reliability of your code.
Conclusion
In conclusion, converting a character array to an integer in Java can be achieved using various methods, each with its advantages and considerations. The choice of method depends on factors such as simplicity, performance, and specific use case requirements.
Whether you opt for the simplicity of Integer.parseInt()
or the flexibility of manual construction using loops, Java provides multiple avenues for performing this common operation. Choose the method that best fits your needs and enhances the readability and maintainability of your code.
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java