How to Sort Array of Objects by Single Key With Date Value
- What a JavaScript Array Is
- What a JavaScript Object Is
- What an Array of Objects Is
-
What
array.sort
Prototype in Javascript Is - Sort an Array of Objects With the Date Value
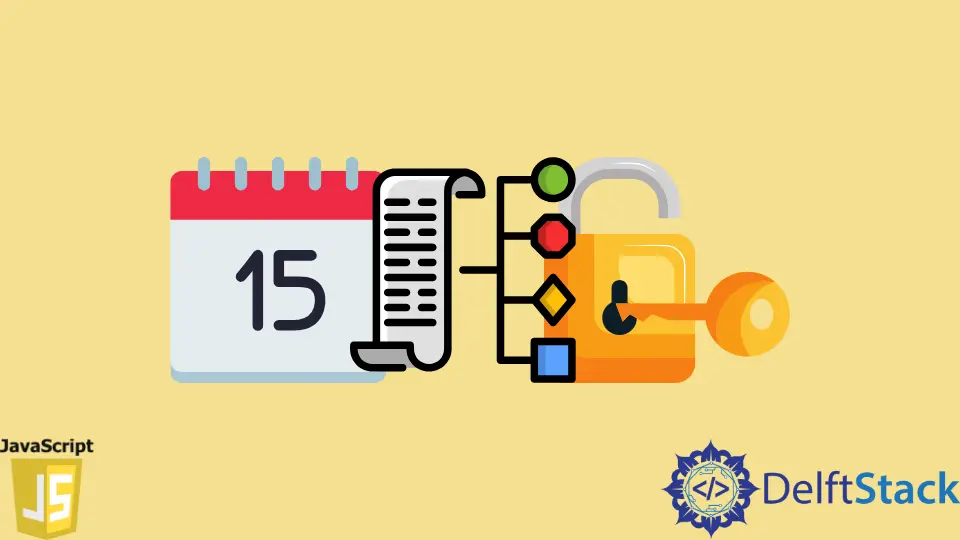
In this article, we will discuss ways to sort an array of objects by single key with date value and understand the following:
- What an Array is
- What an Object is
- What an array of Objects is
- What
array.sort
Prototype in JavaScript is - How to sort an array of objects with the date value
What a JavaScript Array Is
In JavaScript, an array stores multiple data types in a single variable. Unlike some other programming languages, a JavaScript array can hold different data types in the same array.
Each item in an array is stored in the RAM one after the other.
const randomArray = ['Tahseen', 1, 0.3, true];
console.log(randomArray);
// logs: Tahseen , 1 , 0.3 , true
Every item in an array can be accessed by passing its index to the array variable,
console.log(randomArray[0]);
// logs: Tahseen
What a JavaScript Object Is
A JavaScript Object is a collection of key-value pairs.
A key
is a characteristic or a property of an object. Meanwhile, a value
is that of that corresponding property.
const Car = {
company: 'Tesla',
model: 'Model 3',
year: 2017
};
A value of JavaScript objects property can be retrieved by combining the object name with the property name; we can see that in the following code segment.
console.log(Car.company);
// logs: Tesla
What an Array of Objects Is
An array of objects is an array in which each item inside the JavaScript Array is an Object. An array of objects is similar to any other JavaScript array, with the distinction that each item is a JavaScript object.
Let’s check the following example for better understanding:
const MyAppointments = [
{
'with': 'Doctor Tauseef',
'designation': 'Dentist',
'reason': 'Toothache',
'date': '2021-12-01T06:25:24Z',
},
{
'with': 'Abdullah Abid',
'designation': 'Software Engineer',
'reason': 'An Idea for a App',
'date': '2021-12-01T06:25:24Z',
},
{
'with': 'Muhammad Haris',
'designation': 'Painter',
'reason': 'Need to pain the house',
'date': '2021-13-01T06:25:24Z',
},
]
What array.sort
Prototype in Javascript Is
The Array.prototype.sort()
method without any arguments is used to sort an array in ascending order. But keep in mind that the function converts array elements to string and compares them based on their sequence of UTF-16 code units, so it might not look sorted to a normal user.
const Months = ['March', 'Jan', 'Feb', 'Dec'];
Months.sort();
console.log(Months);
// logs: ["Dec", "Feb", "Jan", "March"]
Alternatively, a compare function can be provided as a callback function to the array.sort
, which will sort the array in the following criteria:
Compare Function(x,y) | Soring Order |
---|---|
if return value greater than zero |
place y before x |
if return value less than zero |
place x before y |
if return value equals zero |
keep the same sequence |
const Numbers = [4, 2, 5, 1, 3];
Numbers.sort((x, y) => x - y);
console.log(Numbers);
// logs: [1, 2, 3, 4, 5]
Sort an Array of Objects With the Date Value
You can sort an array of objects by date using the same sort method discussed above.
let MyAppointments = [
{
'with': 'Doctor Tauseef',
'designation': 'Dentist',
'reason': 'Toothache',
'appointment_date': '2021-12-01T06:25:24Z',
},
{
'with': 'Abdullah Abid',
'designation': 'Software Engineer',
'reason': 'An Idea for a App',
'appointment_date': '2021-12-09T06:25:24Z',
},
{
'with': 'Muhammad Haris',
'designation': 'Painter',
'reason': 'Need to pain the house',
'appointment_date': '2021-12-05T06:25:24Z',
},
]
MyAppointments.sort(function(x, y) {
var firstDate = new Date(x.appointment_date),
SecondDate = new Date(y.appointment_date);
if (firstDate < SecondDate) return -1;
if (firstDate > SecondDate) return 1;
return 0;
});
console.log(MyAppointments);
This will compare each date and return -1
if the first date is less than the second. If the first date is greater than the second date, it will return 1
; otherwise, it will return 0
.
The above code segment logs the following array.
[{
with: 'Doctor Tauseef',
designation: 'Dentist',
reason: 'Toothache',
appointment_date: '2021-12-01T06:25:24Z'
},
{
with: 'Muhammad Haris',
designation: 'Painter',
reason: 'Need to pain the house',
appointment_date: '2021-12-05T06:25:24Z'
},
{
with: 'Abdullah Abid',
designation: 'Software Engineer',
reason: 'An Idea for a App',
appointment_date: '2021-12-09T06:25:24Z'
}]
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript