JavaScript Array.sort() Method
-
Syntax of JavaScript
array.sort()
: -
Example Code: Use the
array.sort()
Method to Sort the Elements of the Given Array -
Example Code: Use the
array.sort()
Method WithcompareFunction
to Sort Numbers in Ascending Order -
Example Code: Use the
array.sort()
Method to Sort the Array of Numbers in Descending Order
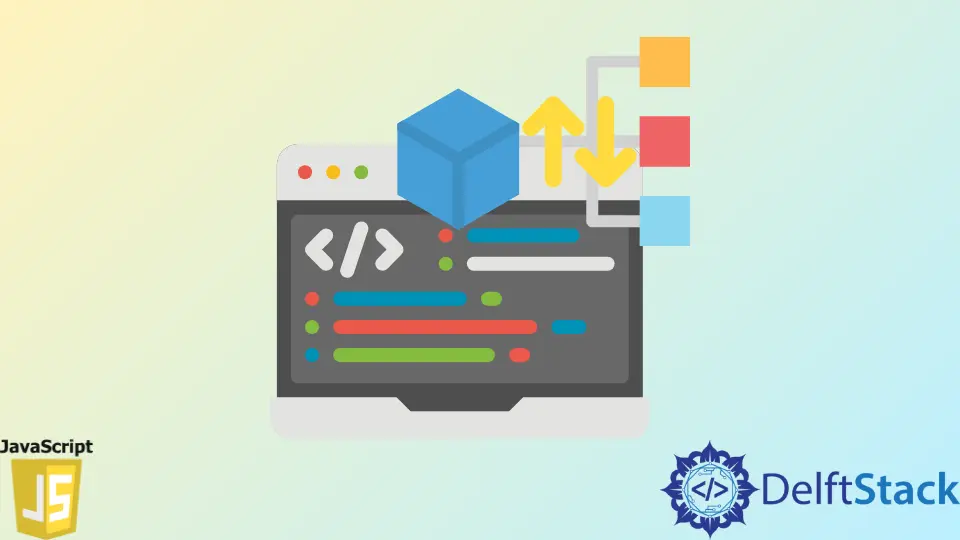
In JavaScript, the array.sort()
method sorts the elements of an array in alphabetical order. This method can also be used to sort the elements based on an argument mentioned in compareFunction
.
Syntax of JavaScript array.sort()
:
refarray.sort();
refarray.sort(function compareFunction(value1,value2){/* ... */});
Parameters
compareFunction |
It compares the two elements according to custom conditions and returns the value accordingly. |
value1 |
It is the first element to compare. |
value2 |
It is the second element to compare. |
Return
This method sorts the array elements and returns the reference to the original array.
How the Returned Value From compareFunction
Sorts the Array Elements
When users don’t pass the compareFunction
parameter of the array.sort()
method, it sorts the array in ascending order by default.
If the compareFunction
returns the value >0
, array.sort()
method sorts the value2
before value1
, and if it returns the value <0
, array.sort()
method sorts the value1
before value2
. If the returned value from compareFunction
is 0
, it keeps the same order.
Example Code: Use the array.sort()
Method to Sort the Elements of the Given Array
In the example below, we have used the array.sort()
method to sort the array of strings. Users can see in the output that JavaScript
is sorted before Javascript
as it contains the uppercase S
.
const arr = ["Javascript","JavaScript","C++"];
arr.sort();
console.log(arr);
Output:
[C++, JavaScript, Javascript]
Example Code: Use the array.sort()
Method With compareFunction
to Sort Numbers in Ascending Order
When we use the array.sort()
method to sort the numbers, we might get incorrect output because 2 is more than 1, and 10 is considered less than 2.
In this example, we have created an array and used the array.sort()
method to get the output. Then, we used compareFunction
as a parameter with the array.sort()
method.
We compared the numbers using the a-b
condition in the function. In the first output, we got 10 before 2 because we didn’t pass any function to compare the numbers.
const arr = [4, 10, 1, 5, 2];
console.log(arr.sort());
arr.sort(function(a, b){
return a-b
});
console.log(arr);
Output:
[1, 10, 2, 4, 5]
[1, 2, 4, 5, 10]
Example Code: Use the array.sort()
Method to Sort the Array of Numbers in Descending Order
In the example, we have created the array of numbers. We have also used the compareFunction
parameter of the array.sort()
method to sort the array in descending order.
The compareFunction
returns the positive, negative, or 0 value according to the condition b-a
, and it will sort the array in descending order.
const array = [40, 50, 32, 15, 22];
array.sort(function compareFunction(a, b){
return b - a;
});
console.log(array);
Output:
[ 50, 40, 32, 22, 15 ]
The array.sort()
method sorts the elements and returns the list of the given array in alphabetical and ascending order.