How to Convert Object to Array in JavaScript
- Using Object.entries()
- Using Object.keys() and Array.map()
- Using Array.from() with Object.entries()
- Conclusion
- FAQ
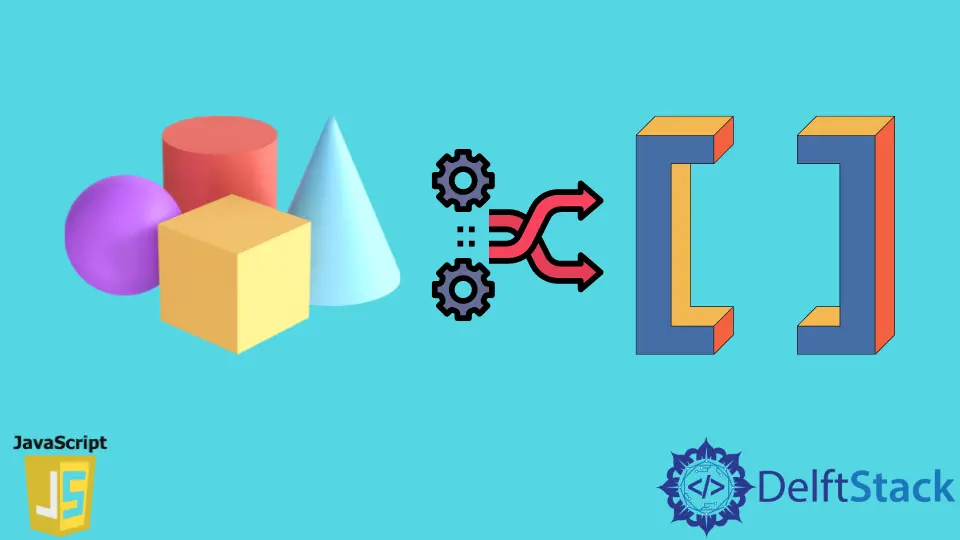
Converting an object to an array in JavaScript is a common task that developers often encounter. Whether you need to manipulate data or simply want to iterate through key-value pairs, understanding how to perform this conversion is essential.
In this tutorial, we will explore various methods to convert an object into an array of key-value pairs. By the end of this guide, you’ll have a solid grasp of the techniques available and be ready to implement them in your projects. So, let’s dive in and unlock the potential of JavaScript objects and arrays!
Using Object.entries()
One of the most straightforward methods to convert an object to an array in JavaScript is by using the Object.entries()
method. This method returns an array of a given object’s own enumerable string-keyed property [key, value] pairs.
Here’s how it works:
const obj = {
name: 'John',
age: 30,
city: 'New York'
};
const array = Object.entries(obj);
console.log(array);
Output:
[ ['name', 'John'], ['age', 30], ['city', 'New York'] ]
The Object.entries()
method takes the object obj
and transforms it into an array of arrays, where each inner array contains a key and its corresponding value. This is particularly useful when you want to work with both keys and values together. The resulting array structure makes it easy to iterate through, allowing for various operations such as mapping, filtering, or reducing.
Using Object.keys() and Array.map()
Another method to convert an object to an array involves using Object.keys()
in combination with Array.map()
. The Object.keys()
method returns an array of a given object’s own enumerable property names, which you can then map to their corresponding values.
Here’s an example:
const obj = {
name: 'Alice',
age: 28,
city: 'Los Angeles'
};
const array = Object.keys(obj).map(key => [key, obj[key]]);
console.log(array);
Output:
[ ['name', 'Alice'], ['age', 28], ['city', 'Los Angeles'] ]
In this approach, Object.keys(obj)
retrieves all the keys from the object. The map()
function then iterates through each key and creates an array where each element is an array of the key and its corresponding value. This method is quite flexible and allows for additional transformations if needed, providing a clear and concise way to convert objects to arrays.
Using Array.from() with Object.entries()
If you prefer a more functional programming style, you can also use Array.from()
along with Object.entries()
. This method creates a new, shallow-copied Array instance from an array-like or iterable object.
Here’s how you can do it:
const obj = {
product: 'Laptop',
price: 1200,
brand: 'Dell'
};
const array = Array.from(Object.entries(obj));
console.log(array);
Output:
[ ['product', 'Laptop'], ['price', 1200], ['brand', 'Dell'] ]
In this case, Object.entries(obj)
generates the key-value pairs, and Array.from()
converts those pairs into a new array. This method is particularly useful when you want to ensure that you are working with a true array, as it allows for additional array methods to be applied later.
Conclusion
In this article, we’ve explored multiple methods to convert an object to an array in JavaScript, including Object.entries()
, Object.keys()
with Array.map()
, and Array.from()
. Each method has its own advantages, depending on your specific use case. By mastering these techniques, you will enhance your ability to manipulate data effectively in your JavaScript applications. Now that you have the tools at your disposal, go ahead and experiment with these methods in your own projects!
FAQ
-
How can I convert an object to an array of values only?
You can useObject.values(obj)
to get an array of the object’s values. -
Is there a method to convert an object to a flat array?
Yes, you can useObject.entries(obj).flat()
to convert the object to a flat array of key-value pairs. -
Are there any performance differences between these methods?
While performance can vary based on the size of the object, in most cases, the difference is negligible for typical use cases. -
Can I convert nested objects to arrays using these methods?
These methods work on the top level of the object. For nested objects, you may need to implement a recursive function to flatten them. -
Which method is the most commonly used?
Object.entries()
is often favored for its simplicity and readability when converting objects to arrays.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript