How to Convert Array to String Without Commas in JavaScript
-
Use the
join()
Method With Blank Value("")
to Convert Array to String Without Commas in JavaScript -
Use the
join()
Method With Blank Space(" ")
to Separate the Characters of the String
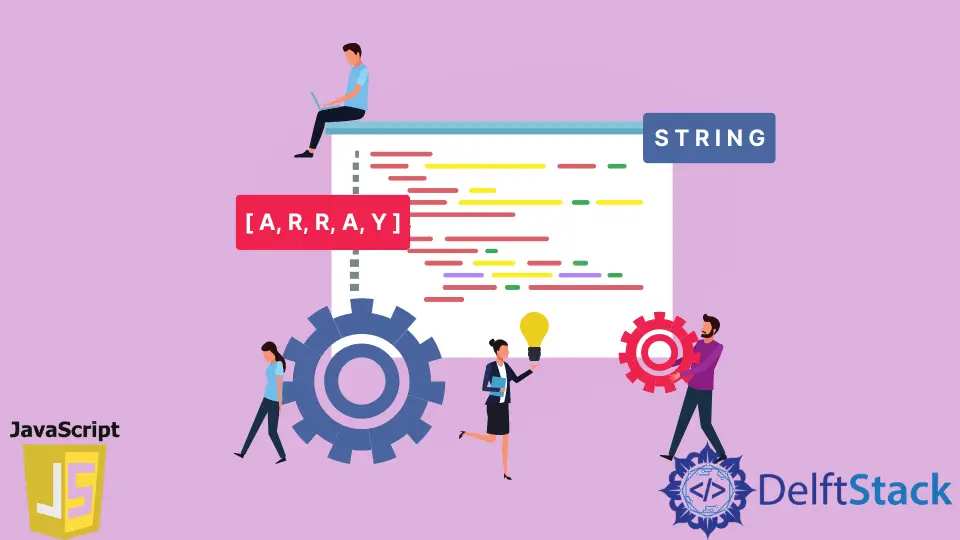
In JavaScript, we have the toString()
and join()
methods to directly convert an array to a string. Also, we can convert it to a string by fetching one value from the array and later typecasting and assigning it to another string to print the whole array.
Here, our motive is to eliminate any separator available in the array. Generally, the separator used in the array is a comma (,)
.
The toString()
method does convert the whole array to a string but does not work on the commas. Consequently, we get a string with commas.
But the join()
method can disregard the commas, and separators or intermediate signs can be appended. The examples defined below will provide a clear idea of how it works.
Use the join()
Method With Blank Value ("")
to Convert Array to String Without Commas in JavaScript
When initiated without any parameter, the join()
method prints the separators in the string. However, the common practice is to set a blank value as the parameter.
This convention is often expected to be the default way of converting an array to a string. Let’s check the code fence.
Code Snippet:
var arr = [1, 2, 3, 4];
console.log(arr.join(''));
console.log(typeof (arr.join('')));
Output:
Use the join()
Method With Blank Space (" ")
to Separate the Characters of the String
The usual way defines the join()
method without any parameter will keep the commas in output. But if we wish to exclude commas, we use a blank value as a parameter.
This made our output look congested, and it lacked grace. So, it can be a preferable fact to choose a blank space instead of a blank value.
Let’s hop on to the code lines.
Code Snippet:
var arr = [1, 2, 3, 4];
console.log(arr.join(' '));
console.log(typeof (arr.join(' ')));
console.log(arr.join());
Output:
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript