How to Filter Object Arrays Based on Attributes in JavaScript
- General Call-Back Function to Filter an Object Array Based on Attributes in JavaScript
- Use Arrow Function to Filter an Object Array Based on Attributes in JavaScript
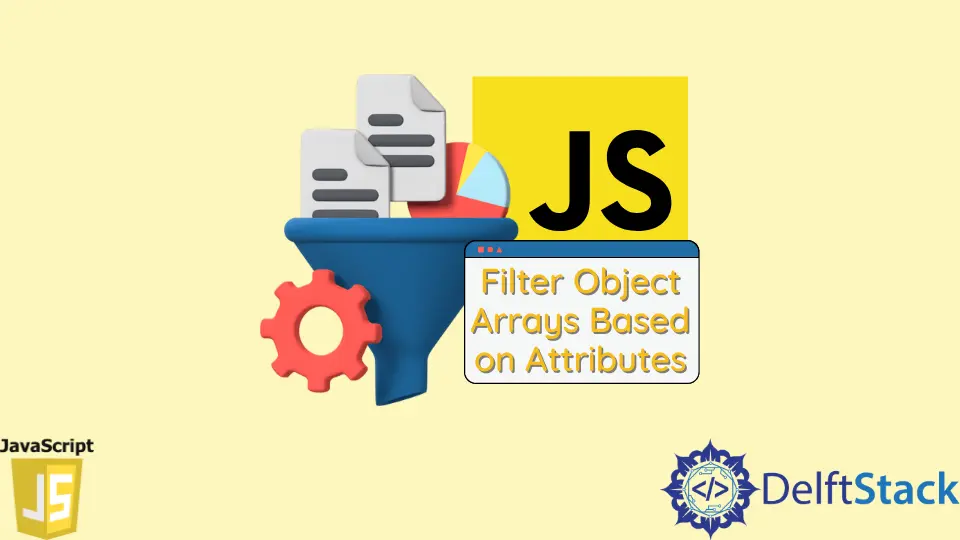
The filter()
method is the easiest way to grab a result of preference.
Other than that, you can loop through the entire object array and declare conditions explicitly. Technically, the less-code and easy access technique is by the filter
method.
Here, we will focus on two ways of using a filter on an object array. We will direct a call-back function to a variable or object to store the filtered result.
This call-back function will have the general structure of a function. Later, we will also try to solve the case with an arrow function and check if the outcome is similar.
General Call-Back Function to Filter an Object Array Based on Attributes in JavaScript
This demonstration will initialize an array with multiple attributes, aka key-value
pairs. The strategy is to access the object first and then access the array.
Next, we will grab an attribute and apply a condition to it. When we use the filter
method, the exact match upon the conditions will get filtered.
Let’s hop on to the code lines.
Code Snippet:
var feature = {
'models': [
{
'name': 'Raven',
'age': '22',
'height': '176',
'gender': 'Female',
'hair': 'Brown',
},
{
'name': 'Alex',
'age': '23',
'height': '185',
'gender': 'Male',
'hair': 'Black',
},
{
'name': 'Eden',
'age': '25',
'height': '169',
'gender': 'Female',
'hair': 'Black',
}
]
} var extract = feature.models.filter(function(el) {
return el.age < 25 && el.height >= 170;
});
console.log(extract);
Output:
Here, the feature.models.filter
accesses the object and array accordingly. Also, the filter
method is fired to put the constraints inside it.
As we can see, the age<25 && height>170
extracts the possible matched models detailing.
Use Arrow Function to Filter an Object Array Based on Attributes in JavaScript
An arrow function is a shorthand way of the normal function structure conventions. We will see if we can use an arrow function to filter an object array.
Code Snippet:
var feature = {
'models': [
{
'name': 'Raven',
'age': '22',
'height': '176',
'gender': 'Female',
'hair': 'Brown',
},
{
'name': 'Alex',
'age': '23',
'height': '185',
'gender': 'Male',
'hair': 'Black',
},
{
'name': 'Eden',
'age': '25',
'height': '169',
'gender': 'Female',
'hair': 'Black',
}
]
} var extract = feature.models.filter(x => x.age < 25 && x.hair == 'Black');
console.log(extract);
Output:
According to the code above, filtering out the necessary result with an arrow function filter
method is clearer.
This procedure only requires a variable (here x
) to point out the attributes. Also, this coding style is used to shorten codes and issued by the latest ES6 convention.
Related Article - JavaScript Object
- How to Search Objects From an Array in JavaScript
- How to Get the Object's Value by a Reference to the Key
- How to Find Object in Array by Property Value in JavaScript
- How to Print Objects in JavaScript
- How to Destroy Object in JavaScript
- Nested Objects in JavaScriptn
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript