List of Arrays in Java
-
What Is a
List
in Java -
What Are
Arrays
in Java -
What Is a
List
ofArrays
in Java -
Create a
List
ofArrays
in Java - Conclusion
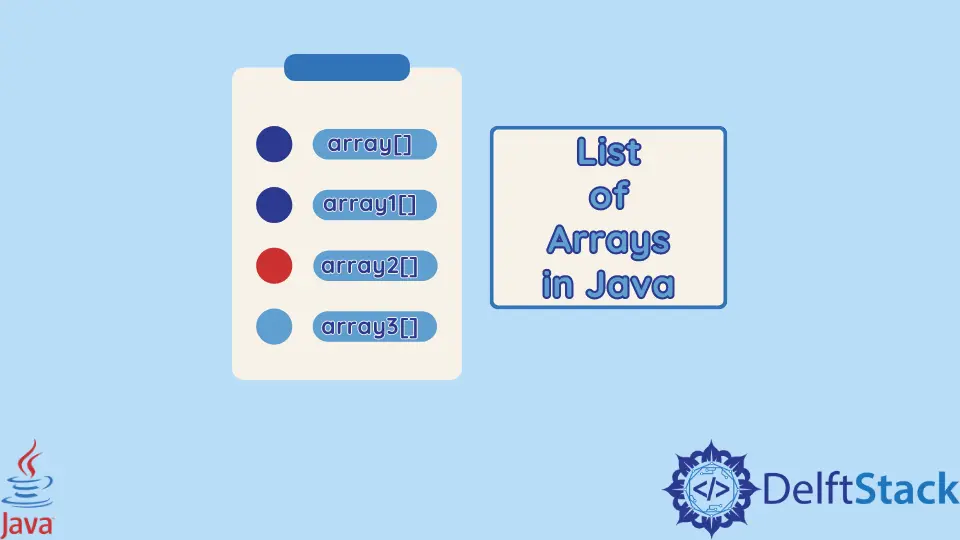
Java is a popular and powerful programming language used in multiple domains. Therefore, we often encounter situations where we want to store the data as an ordered collection.
This article provides a concise exploration of List
and Arrays
in Java, introduces the concept of List
of Arrays
, and outlines the procedure for creating and utilizing a List
of Arrays
.
What Is a List
in Java
In Java, a List
is a dynamic and ordered collection of elements. It allows you to store and manipulate data in a flexible manner.
Lists
can contain elements of different types, and their size can change during the program’s execution. Common implementations of List
in Java include ArrayList
and LinkedList
, providing various methods for adding, removing, and accessing elements.
Lists
are fundamental for managing and organizing data in Java programs. The Java lists are index-based, where elements can be accessed using indices.
We can store objects, arrays, and any other complex data type in a list, and we should note that a list can even store a null element. Since the List
is an interface, we can not directly instantiate an object of type List
.
We can also create our list type by implementing the List
interface and defining its methods. We can define and instantiate an ArrayList
as given below.
List<datatype> nameList = new ArrayList<datatype>();
Note that we can not instantiate an interface, but we can define an object of an interface. Therefore, we need to instantiate a specific list type such as ArrayList
, LinkedList
, etc.
We can use the different syntax as given below. As the List
is the parent of the ArrayList
, the List type object can store an ArrayList
object.
ArrayList<datatype> nameList = new ArrayList<datatype>();
The syntax above defines an object of ArrayList
and instantiates the ArrayList
type. We can also define the List
and instantiate it later.
What Are Arrays
in Java
In Java, an array is a fixed-size, ordered collection of elements. All elements in an array must be of the same type.
Arrays provide a structured way to store and access multiple values using a single variable. The size of an array is determined at the time of its creation and cannot be changed during runtime.
Elements in an array are accessed by their index, starting from 0. Arrays are a fundamental data structure in Java, commonly used for tasks that involve handling sets of similar data.
Similar to lists, arrays are also not limited to primitive data types, and we can create arrays with any complex data types.
Arrays can be defined directly and can be instantiated dynamically in Java. Java arrays inherit the object class and implement cloneable and serializable interfaces.
The syntax to declare and instantiate an array in Java is given below.
datatype nameArr[] = new datatype[size];
We can also use a slightly different syntax, as given below.
datatype[] nameArr = new datatype[size];
Note that we can define an array and instantiate it later as well.
What Is a List
of Arrays
in Java
In Java, a List
of Arrays
is a dynamic collection where each element is an array. It combines the flexibility of a List
(dynamic size) with the structured nature of arrays.
This means you can have an evolving number of arrays in your list, and each array may contain elements of the same type. It’s useful when you need a collection that can grow or shrink, and each element needs to be an array.
Create a List
of Arrays
in Java
In the realm of Java programming, efficient data management is crucial. A concept that shines in this regard is the List
of Arrays.
This approach amalgamates the versatility of lists with the structured nature of arrays, presenting a dynamic and organized solution to handle data in various scenarios. Let’s delve into this concept, exploring its significance and unveiling its potential through a comprehensive code example.
Consider the following Java code snippet that demonstrates the use of a List
containing arrays:
import java.util.ArrayList;
import java.util.List;
public class ListOfArraysExample {
public static void main(String[] args) {
// Creating a List of Arrays
List<int[]> listOfArrays = new ArrayList<>();
// Adding arrays to the list
listOfArrays.add(new int[] {1, 2, 3});
listOfArrays.add(new int[] {4, 5, 6});
listOfArrays.add(new int[] {7, 8, 9});
// Accessing and printing elements from the list
for (int[] array : listOfArrays) {
for (int element : array) {
System.out.print(element + " ");
}
System.out.println();
}
}
}
In this code example, we start by importing essential Java utility classes and defining a class named ListOfArraysExample
. Inside the main
method, a List
named listOfArrays
is created to store arrays of integers.
Following this, we populate the list with three arrays, each representing a row of integer elements. The subsequent step involves using nested loops to iterate through the list and the arrays within it.
This iteration prints each element to the console, resulting in a structured and organized output.
The power of the List
of Arrays
becomes evident as the code effortlessly manages a dynamic collection of arrays. The output of this example showcases the organized presentation of the elements:
This structured approach offers flexibility in handling multidimensional data, making the List
of Arrays
a valuable tool in Java programming. Whether dealing with matrices, datasets, or any scenario requiring dynamic arrays, this concept proves to be a versatile and efficient choice.
Conclusion
In Java programming, a List
is a dynamic, ordered collection of elements, providing flexibility in managing data. Arrays, on the other hand, offer a fixed-size, ordered structure for storing elements of the same type.
Combining these concepts, a List
of Arrays
in Java represents a dynamic collection where each element is an array, allowing for both flexibility and structured data storage. Creating a List
of Arrays
involves initializing a List and populating it with arrays, offering a versatile solution for handling varying data structures within a dynamic context.
This approach is invaluable when dealing with scenarios requiring both adaptability and organized data management in Java.
Discover the differences between Lists
and Arrays
in Java by checking out this link: List
vs. Array
in Java.
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java