How to Remove a Character From String in Java
-
Use the
replace
Function to Remove a Character From String in Java -
Use the
deleteCharAt
Method to Remove a Character From String in Java -
Use the
substring
Method to Remove a Character From String in Java
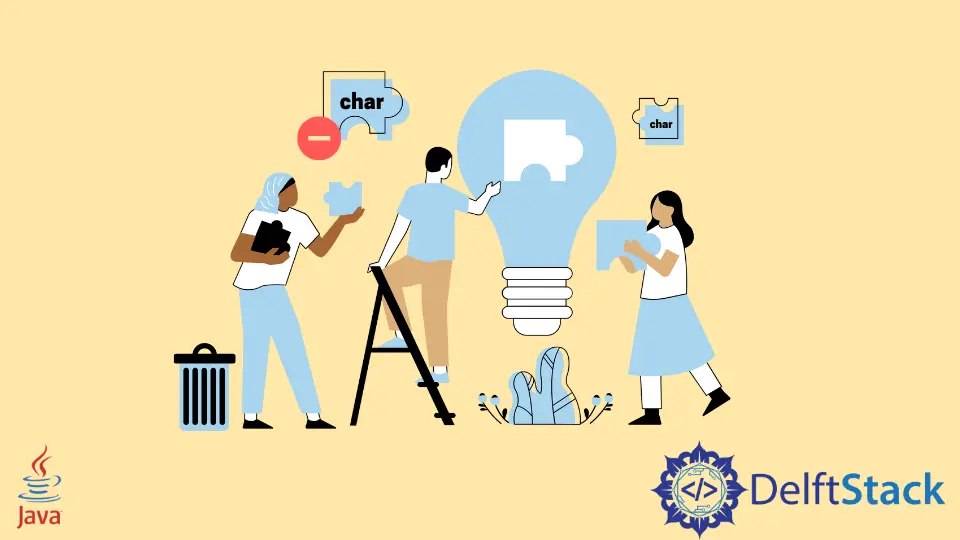
This tutorial article will describe how to remove a character from a string in Java.
There are several built-in functions to remove a particular character from a string that is as follows.
replace
FunctiondeleteCharAt
Functionsubstring
Function
Use the replace
Function to Remove a Character From String in Java
The replace
function can be used to remove a particular character from a string in Java.
The replace
function takes two parameters, the first parameter is the character to be removed, and the second parameter is the empty string.
The replace
function replaces the character with the empty string that results in removing a particular character that is passed along with an empty string.
The example code of using the replace
function to remove a character from a string in Java is as follows.
public class RemoveCharacter
{
public static void main(String[] args)
{
String MyString = "Hello World";
System.out.println("The string before removing character: " + MyString);
MyString = MyString.replace(" ", "");
System.out.println("The string after removing character: " + MyString);
}
}
In this above code, we remove the whitespace between the Hello
and World
. We pass a white space along with the empty string and the white space is replaced with the empty string, or in other words, removed from the Hello World
string.
The output of the code is as follows.
The string before removing character : Hello World The string after removing character : HelloWorld
Use the deleteCharAt
Method to Remove a Character From String in Java
The deleteCharAt()
method is a member method of the StringBuilder
class that can also be used to remove a character from a string in Java. To remove a particular character from a string, we have to know that character’s position if we want to remove that character using the deleteCharAt
method.
The deleteCharAt()
method takes that particular character’s position that we want to remove from the string. So we need to know the position of that particular character when using the deleteCharAt
method to remove the particular character from the string.
The example code of using the deleteCharAt
method to remove a character from a string in Java is as follows.
public class RemoveCharacter
{
public static void main(String[] args)
{
StringBuilder MyString = new StringBuilder("Hello World");
System.out.println("The string before removing character: " + MyString);
MyString = MyString.deleteCharAt(5);
System.out.println("The string after removing character: " + MyString);
}
}
In the above code, we remove white space between the Hello
and World
. We pass the position of the white space, that is 5, in the string Hello World
because the index starts from 0 in Java.
The output of the code is as follows.
The string before removing character: Hello World
The string after removing character: HelloWorld
Use the substring
Method to Remove a Character From String in Java
The substring
method can also be used to remove a character from a string in Java. To remove a particular character using the substring
method, we have to pass the starting position and the position before the removing character. After that, we concatenate the string from the position where our character is situated in the string.
The substring
method splits the string according to the starting and ending index and then concatenates the same string by overwriting the character we want to remove from the string.
The example code of using the substring
method to remove a character from a string in Java is as follows.
public class RemoveCharacter
{
public static void main(String[] args)
{
String MyString = "Hello World";
int Position = 5;
System.out.println("The string before removing character: " + MyString);
MyString = MyString.substring(0,Position) + MyString.substring(Position+1);
System.out.println("The string after removing character: " + MyString);
}
In this above code, we remove the white space between Hello
and World
. We know the position of the white space in a variable that is 5. We split the Hello World
from 0th to the 5th position using the substring
method and concatenate the other parts of the string from the 6th position. By this, we remove the white space from the Hello World
.
The output of the code is as follows.
The string before removing character: Hello World
The string after removing character: HelloWorld
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java