How to Calculate Power of Integers in Java
-
Use the
for
Loop to Calculate the Power of an Integer in Java - Use Recursion to Calculate the Power of an Integer in Java
-
Use
Math.pow()
to Calculate the Power of an Integer in Java
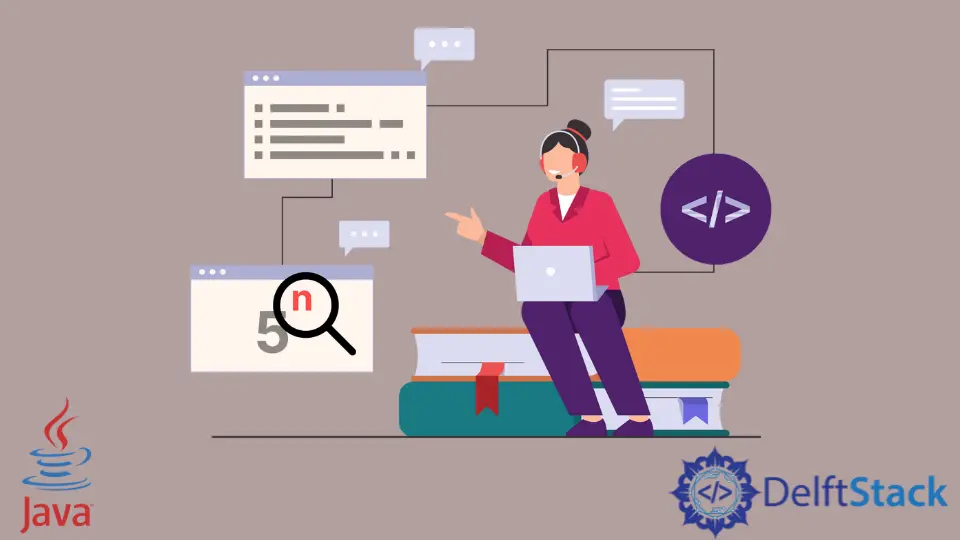
In this tutorial, we will look into various approaches to calculate the power of an integer. There are standard methods for this purpose. It can also be achieved using a loop as well as the Java library function.
Use the for
Loop to Calculate the Power of an Integer in Java
The power of a number is the number of times a number is to be multiplied by itself. We calculate the power of a number using a for
loop as given below. Here we have variables, num
and power
of data type int
.
The function calculatePower(int num, int power)
has three conditions. First, if the number is greater than 0 but the power is 0 it will return 1. If the power is not 0, but the number is 0, then 0 is returned.
In the other case, we run a loop that calculates the given number’s exponent raised to the given power.
public class Power {
public static void main(String args[]) {
int number = 5;
int power = 3;
int result = calculatePower(number, power);
System.out.println(number + "^" + power + "=" + result);
}
static int calculatePower(int num, int power) {
int answer = 1;
if (num > 0 && power == 0) {
return answer;
} else if (num == 0 && power >= 1) {
return 0;
} else {
for (int i = 1; i <= power; i++) {
answer = answer * num;
}
return answer;
}
}
}
Output:
5^3=125
Use Recursion to Calculate the Power of an Integer in Java
We can use the recursive function CalculatePower
to calculate the power of an integer. A Recursion is a mechanism that helps a function call itself.
Here the recursive call to the CalculatePower
function continues until the stop condition meets, which is if pow
we passed is equal to 0.
public class Power {
public static void main(String args[]) {
int number = 3;
int power = 3;
int result = CalculatePower(number, power);
System.out.println(number + "^" + power + "=" + result);
}
static int CalculatePower(int num, int pow) {
if (pow == 0)
return 1;
else
return num * CalculatePower(num, pow - 1);
}
}
Output:
3^3=27
Use Math.pow()
to Calculate the Power of an Integer in Java
We can easily calculate the power of an integer with the function Math.pow()
provided by the Java library. The number raised to the power of some other number can be calculated using this function.
This method takes two parameters; the first number is the base while the second is the exponent. If the pass exponent is 0, then the returned result will be 1, while the result will be the base value only if the exponent is 1. If the second parameter passed is NaN
, then the result will also be NaN
.
import java.lang.Math;
public class Power {
public static void main(String args[]) {
int number = 6;
int power = 3;
double result = CalculatePower(number, power);
System.out.println(number + "^" + power + "=" + result);
}
static double CalculatePower(int num, int pow) {
return Math.pow(num, pow);
}
}
Output:
6^3=216.0
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Math
- How to Calculate Probability in Java
- How to Find Factors of a Given Number in Java
- How to Evaluate a Mathematical Expression in Java
- How to Calculate the Euclidean Distance in Java
- How to Calculate Distance Between Two Points in Java
- How to Simplify or Reduce Fractions in Java