How to Simplify or Reduce Fractions in Java
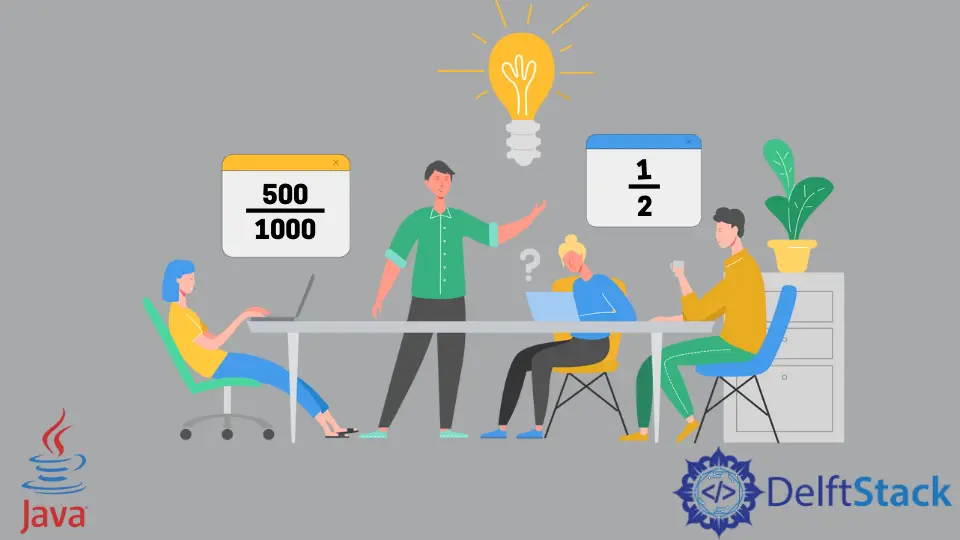
Fractions are a fundamental concept in mathematics, and sometimes, it’s essential to simplify or reduce them to their simplest form. In Java, simplifying fractions can be achieved through a systematic process, and
In this article, we’ll walk through the steps of implementation along with a detailed code example.
Simplify and Reduce Fractions in Java
Firstly, let’s create a Java class, Fraction
, to represent fractions. This class will have two private attributes, the numerator
and the denominator
, representing the parts of a fraction.
The constructor public Fraction(int numerator, int denominator)
initializes these attributes with the values passed as arguments when creating a Fraction
object.
public class Fraction {
private int numerator;
private int denominator;
public Fraction(int numerator, int denominator) {
this.numerator = numerator;
this.denominator = denominator;
}
}
Then, to simplify a fraction, we need to find the greatest common divisor (GCD) of the numerator and denominator. We can implement a method to calculate the GCD using the Euclidean algorithm.
So, we define a private method named findGCD
within the Fraction
class, which calculates the greatest common divisor (GCD) of two integers (a
and b
) using the Euclidean algorithm. The algorithm iteratively replaces a
with b
and b
with a % b
until b
becomes zero, at which point a
is the GCD.
private int findGCD(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
Now, let’s add a simplify
method to our Fraction
class that simplifies the fraction using the GCD we calculated earlier.
This uses the findGCD
method to calculate the GCD of the numerator and denominator. Then, it divides both the numerator and denominator by the GCD, effectively simplifying the fraction.
public void simplify() {
int gcd = findGCD(numerator, denominator);
numerator /= gcd;
denominator /= gcd;
}
We should also include a method to display the fraction. Here’s the complete Fraction
class:
public class Fraction {
private int numerator;
private int denominator;
public Fraction(int numerator, int denominator) {
this.numerator = numerator;
this.denominator = denominator;
}
private int findGCD(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
public void simplify() {
int gcd = findGCD(numerator, denominator);
numerator /= gcd;
denominator /= gcd;
}
public void display() {
System.out.println(numerator + "/" + denominator);
}
}
Now, let’s create a main class to test our Fraction
class and its simplification method.
Here’s the complete working code example:
public class Fraction {
private int numerator;
private int denominator;
public Fraction(int numerator, int denominator) {
this.numerator = numerator;
this.denominator = denominator;
}
private int findGCD(int a, int b) {
while (b != 0) {
int temp = b;
b = a % b;
a = temp;
}
return a;
}
public void simplify() {
int gcd = findGCD(numerator, denominator);
numerator /= gcd;
denominator /= gcd;
}
public void display() {
System.out.println(numerator + "/" + denominator);
}
public static void main(String[] args) {
Fraction fraction = new Fraction(4, 8);
System.out.println("Original Fraction:");
fraction.display();
fraction.simplify();
System.out.println("Simplified Fraction:");
fraction.display();
}
}
Output:
Original Fraction:
4/8
Simplified Fraction:
1/2
Here, we start by creating a Fraction
class with attributes for the numerator and denominator. The findGCD
method calculates the greatest common divisor using the Euclidean algorithm.
The simplify
method then uses this GCD to simplify the fraction by dividing both the numerator and denominator.
In the test example, we create a Fraction
object with the values 4
and 8
. We display the original fraction, then call the simplify
method and display the simplified fraction.
This approach allows for easy integration into larger projects where fraction simplification may be required. The Fraction
class can be expanded to include additional functionality as needed.
Conclusion
In summary, our Java program offers a practical and modular solution for simplifying and reducing fractions.
Through a well-structured Fraction
class, we demonstrated the steps involved in finding the greatest common divisor (GCD) and utilizing it to simplify fractions. The resulting code provides a foundation for handling fractions in larger Java projects, promoting modularity and ease of use.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn