How to Calculate the Euclidean Distance in Java
- Understanding Euclidean Distance
- Calculate Euclidean Distance in Java With Predefined Values
- Calculate Euclidean Distance in Java With User-Input Values
- An Alternative Way to Calculate Euclidean Distance in Java With Predefined Values
- Conclusion
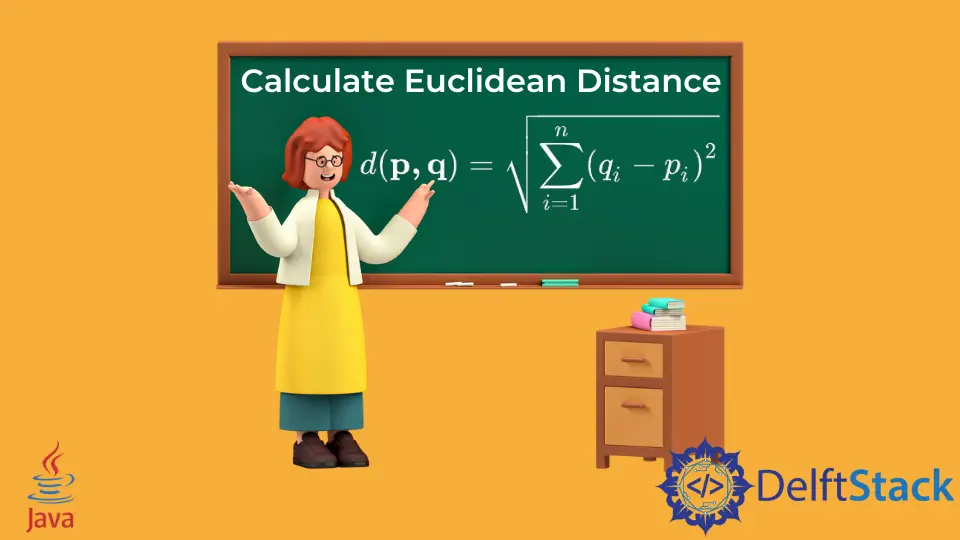
In this tutorial, we will explore the computation of the distance between two points using Java programming. Specifically, we will delve into the concept of Euclidean distance, also known as the Pythagorean distance, and provide examples of calculating it with both predefined and user-input values.
Understanding Euclidean Distance
In Mathematics, Euclidean distance represents the length of a line segment between two locations in Euclidean space. This distance is determined from the Cartesian coordinates of the locations using the Pythagorean theorem.
The formula for calculating Euclidean distance between two points (q_1, p_1)
and (q_2, p_2)
is given as follows:
In this formula, q
and p
represent the coordinates of the two points. The square root ensures that the Euclidean distance is a positive value, representing the length of the shortest path between the two points in the geometric space.
Calculate Euclidean Distance in Java With Predefined Values
Let’s start by examining a Java program that calculates the Euclidean distance between two points with predefined coordinates:
import java.lang.Math.*;
public class DistPoint {
public static void main(String arg[]) {
int q1, q2, p1, p2;
double distance;
q1 = 2;
p1 = 3;
q2 = 4;
p2 = 5;
distance = Math.sqrt((q2 - q1) * (q2 - q1) + (p2 - p1) * (p2 - p1));
System.out.println("Distance between two points: "
+ "(" + q1 + "," + p1 + "), "
+ "(" + q2 + "," + p2 + ") ===> " + distance);
}
}
In this Java program, we have a class named DistPoint
that contains a main
method. The goal of this program is to calculate and display the Euclidean distance between two points in a two-dimensional space.
The variables q1
, q2
, p1
, and p2
are declared to represent the coordinates of two points: (q1, p1)
and (q2, p2)
. These variables are then assigned specific values, which correspond to the coordinates of the two points in Euclidean space.
Here, we use the Math.sqrt
function to compute the square root and use basic arithmetic operations to find the squared differences between the coordinates. The result is stored in the variable distance
, which is of type double
to accommodate potential decimal values in the distance calculation.
distance = Math.sqrt((q2 - q1) * (q2 - q1) + (p2 - p1) * (p2 - p1));
Finally, the program prints the calculated Euclidean distance to the console using the System.out.println
statement.
Output:
Distance between two points: (2,3), (4,5) ===> 2.8284271247461903
Calculate Euclidean Distance in Java With User-Input Values
Now, let’s enhance the program to accept user-input values for the coordinates of the two points:
import java.util.Scanner;
public class Distance {
public static void main(String arg[]) {
int q1, q2, p1, p2;
double distance;
Scanner newnum = new Scanner(System.in);
System.out.println("Enter q1 point:");
q1 = newnum.nextInt();
System.out.println("Enter p1 point:");
p1 = newnum.nextInt();
System.out.println("Enter q2 point:");
q2 = newnum.nextInt();
System.out.println("Enter p2 point:");
p2 = newnum.nextInt();
distance = Math.sqrt((q2 - q1) * (q2 - q1) + (p2 - p1) * (p2 - p1));
System.out.println("Distance between two points: "
+ "(" + q1 + "," + p1 + "), "
+ "(" + q2 + "," + p2 + ") ===> " + distance);
}
}
As you can see, this program is an extension of the previous one but with a notable enhancement – it allows the user to input their values for the coordinates of two points, making the calculation more interactive and versatile.
A Scanner
object named newnum
is created to facilitate user input. This object is initialized with System.in
, indicating that it will read input from the standard input stream.
The program then prompts the user to enter the coordinates of the first point (q1, p1)
by displaying the message Enter q1 point:
. The user’s input is obtained using the newnum.nextInt()
method, and the entered value is assigned to the variable q1
.
Similar prompts and input procedures are repeated for p1
, q2
, and p2
, allowing the user to input the coordinates of the second point (q2, p2)
.
Following the user input, the program proceeds to calculate the Euclidean distance using the same formula as in the previous example. The calculated distance is then stored in the variable distance
.
Finally, the program prints the result to the console using the System.out.println
statement.
Output:
Enter q1 point:
2
Enter p1 point:
3
Enter q2 point:
4
Enter p2 point:
5
Distance between two points: (2,3), (4,5) ===> 2.8284271247461903
An Alternative Way to Calculate Euclidean Distance in Java With Predefined Values
The formula for calculating the Euclidean distance can also be directly implemented in the code using the Math.sqrt
and Math.pow
functions.
Take a look at how it works:
import java.lang.Math;
public class EuclideanDistance {
public static void main(String[] args) {
double x1 = 2;
double y1 = 3;
double x2 = 4;
double y2 = 5;
double distance = Math.sqrt(Math.pow((x2 - x1), 2) + Math.pow((y2 - y1), 2));
System.out.println("Euclidean Distance: " + distance);
}
}
After declaring and initializing the four variables (x1
, y1
, x2
, and y2
) to represent the coordinates of two points: (x1, y1)
and (x2, y2)
, the Euclidean Distance Formula is directly implemented using the Math.sqrt
function for the square root and the Math.pow
function for raising each difference to the power of 2.
The differences in x-coordinates and y-coordinates are squared, summed, and then square-rooted to obtain the Euclidean distance. The result is stored in the variable distance
.
Finally, the program prints the calculated Euclidean distance to the console using the System.out.println
statement.
Output:
Euclidean Distance: 2.8284271247461903
This program provides a more concise and efficient way to calculate Euclidean distance in Java.
Consider another example:
import java.lang.Math;
public class EuclideanDistance {
public static void main(String[] args) {
double x1 = 2;
double y1 = 3;
double x2 = 4;
double y2 = 5;
double distance = calculateEuclideanDistance(x1, y1, x2, y2);
System.out.println("Euclidean Distance: " + distance);
}
public static double calculateEuclideanDistance(double x1, double y1, double x2, double y2) {
return Math.sqrt(Math.pow((x2 - x1), 2) + Math.pow((y2 - y1), 2));
}
}
Here, the main method initializes the coordinates of two points. Instead of directly applying the Euclidean Distance Formula, a function call calculateEuclideanDistance(x1, y1, x2, y2)
is used to perform the calculation.
The calculateEuclideanDistance
function receives the coordinates as parameters and directly applies the Euclidean Distance Formula within its body. It returns the calculated distance as a double
value and stores it in the variable distance
.
Here’s the output:
Euclidean Distance calculated in a separate function: 2.8284271247461903
Using a separate function for Euclidean distance calculation in Java enhances code organization and readability, promoting a modular and reusable approach. This method becomes particularly beneficial when multiple instances of distance calculations are required throughout a program, allowing for efficient and clean code maintenance.
Conclusion
Understanding how to calculate Euclidean distance between two points is essential for various applications, including spatial analysis, geometry, and distance-based algorithms.
In this article, we explored different approaches to computing Euclidean distance in Java, ranging from simple programs with predefined values to more interactive versions that accept user input.
We also introduced a concise implementation using the direct application of the Euclidean Distance Formula and demonstrated the benefits of encapsulating the calculation in a separate function for improved code organization and reusability.
Whether you are working on geometric algorithms, data science applications, or any other field that involves spatial analysis, having a solid understanding of Euclidean distance calculations in Java will empower you to tackle a wide range of problems with confidence.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn