How to Calculate Distance Between Two Points in Java
- Understanding the Distance Formula
- Method 1: Using the Math Class
- Method 2: Using a Custom Distance Class
- Method 3: Using Java Streams (Java 8 and Above)
- Conclusion
- FAQ
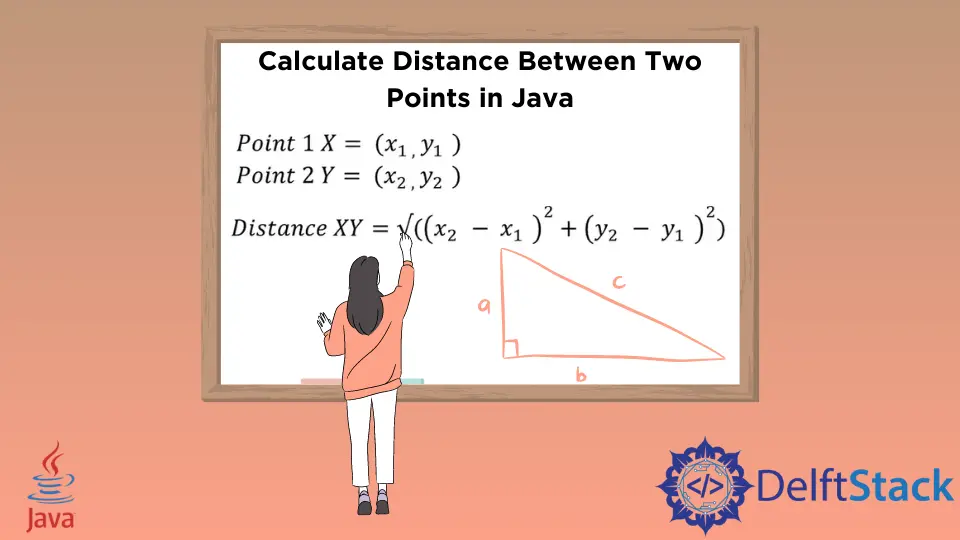
Calculating the distance between two points is a common task in programming, especially in applications involving mapping, navigation, or geometry. In Java, this can be achieved using various methods, but the most popular and straightforward approach is by utilizing the distance formula derived from the Pythagorean theorem.
This tutorial will guide you through the steps to calculate the distance between two points in Java, complete with code examples and explanations. Whether you’re a beginner or an experienced developer, this guide will enhance your understanding of geometric calculations in Java.
Understanding the Distance Formula
Before diving into the code, it’s essential to understand the distance formula itself. The distance (d) between two points ((x_1, y_1)) and ((x_2, y_2)) can be calculated using the formula:
This formula is derived from the Pythagorean theorem, where the distance represents the hypotenuse of a right triangle formed by the horizontal and vertical distances between the two points.
Method 1: Using the Math Class
Java’s built-in Math
class provides a convenient way to calculate the square root, making it easy to implement the distance formula.
public class DistanceCalculator {
public static void main(String[] args) {
double x1 = 1.0, y1 = 2.0;
double x2 = 4.0, y2 = 6.0;
double distance = calculateDistance(x1, y1, x2, y2);
System.out.println("Distance: " + distance);
}
public static double calculateDistance(double x1, double y1, double x2, double y2) {
return Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
}
}
Output:
Distance: 5.0
In this method, we define a class called DistanceCalculator
. Inside the main
method, we initialize two points with their respective coordinates. The calculateDistance
method uses the Math.sqrt
and Math.pow
functions to implement the distance formula. First, it calculates the squared differences in both the x and y coordinates, sums them up, and then takes the square root to find the distance. This approach is efficient and leverages Java’s built-in capabilities.
Method 2: Using a Custom Distance Class
For more complex applications, you might want to encapsulate the distance calculation logic within its own class. This approach enhances code organization and reusability.
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double distanceTo(Point other) {
return Math.sqrt(Math.pow(other.x - this.x, 2) + Math.pow(other.y - this.y, 2));
}
public static void main(String[] args) {
Point point1 = new Point(1.0, 2.0);
Point point2 = new Point(4.0, 6.0);
double distance = point1.distanceTo(point2);
System.out.println("Distance: " + distance);
}
}
Output:
Distance: 5.0
In this method, we create a Point
class that represents a point in a 2D space. The constructor initializes the x and y coordinates. The distanceTo
method takes another Point
object as a parameter and calculates the distance between the two points using the same formula as before. This encapsulation makes the code cleaner and allows for easy extension in the future, such as adding more geometric functionalities.
Method 3: Using Java Streams (Java 8 and Above)
If you’re using Java 8 or later, you can leverage the power of streams for a more functional programming approach to calculate distances.
import java.util.Arrays;
public class DistanceCalculator {
public static void main(String[] args) {
double[] point1 = {1.0, 2.0};
double[] point2 = {4.0, 6.0};
double distance = calculateDistance(point1, point2);
System.out.println("Distance: " + distance);
}
public static double calculateDistance(double[] p1, double[] p2) {
return Math.sqrt(Arrays.stream(p1)
.map(i -> Math.pow(p2[Arrays.asList(p1).indexOf(i)] - i, 2))
.sum());
}
}
Output:
Distance: 5.0
In this method, we use arrays to represent points and the Arrays.stream
method to create a stream from the first point’s coordinates. We then map each coordinate to its squared difference from the corresponding coordinate in the second point and sum them up. Finally, we take the square root of the summed values to get the distance. This method is elegant and showcases the power of Java’s functional programming capabilities.
Conclusion
Calculating the distance between two points in Java can be done in various ways, each with its own advantages. Whether you prefer using the built-in Math
class, creating a dedicated class for points, or utilizing Java Streams, the choice ultimately depends on your specific use case and coding style. By mastering these methods, you can enhance your Java programming skills and apply them to real-world applications, such as mapping and navigation systems.
FAQ
-
How accurate is the distance calculation in Java?
The distance calculation using the methods discussed is mathematically accurate, assuming the input coordinates are correct. -
Can I calculate distances in three-dimensional space using these methods?
Yes, you can extend the distance formula to three dimensions by adding the z-coordinate and modifying the calculations accordingly.
-
What libraries can help with distance calculations in Java?
Libraries like Apache Commons Math and JTS (Java Topology Suite) offer advanced geometric calculations, including distance measurements. -
Is there a built-in Java method for calculating distances?
Java does not have a built-in method specifically for distance calculations, but you can easily implement it using the provided examples. -
Can I use these methods for geographical coordinates?
The methods discussed are suitable for Cartesian coordinates. For geographical coordinates (latitude and longitude), you would need to use the Haversine formula or similar approaches.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook