How to Calculate Probability in Java
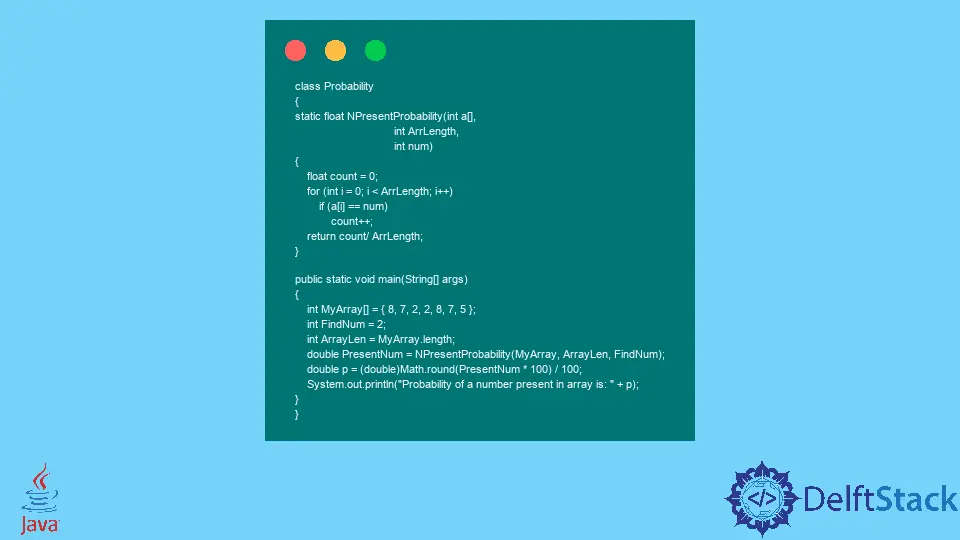
By using probability, you can find the chance of occurring a specific event. It is mainly a future prediction of an event.
If you are working with a program related to Artificial Intelligence, then you may need to apply some calculations in your program to find the probability. Probability is mainly used in these cases where the outcome is uncertain for a trial.
In this article, we will see how we can calculate the probability using Java. Also, we will discuss the topic by using necessary examples and explanations to make the topic easier.
An Example of Finding the Probability in Java
In the example below, we will find the probability of a number present in an array. The code for our example is shown below:
class Probability {
static float NPresentProbability(int a[], int ArrLength, int num) {
float count = 0;
for (int i = 0; i < ArrLength; i++)
if (a[i] == num)
count++;
return count / ArrLength;
}
public static void main(String[] args) {
int MyArray[] = {8, 7, 2, 2, 8, 7, 5};
int FindNum = 2;
int ArrayLen = MyArray.length;
double PresentNum = NPresentProbability(MyArray, ArrayLen, FindNum);
double p = (double) Math.round(PresentNum * 100) / 100;
System.out.println("Probability of a number present in array is: " + p);
}
}
Let’s explain the code part by part. We first take an integer array with some numbers in the code through the line int MyArray[] = { 8, 7, 2, 2, 8, 7, 5 };
.
On our second line, we declared an integer variable that holds the number we need to find in the array. After that, we took another variable that holds the array’s length.
Then we declared another double variable with the output value of the function NPresentProbability()
. We passed three parameters here; these are the array, array length, and the number we want to find.
Now we are going to discuss the function NPresentProbability()
. The purpose of this function is to find the probability.
Inside the function, we first count the number of matches with the provided number. Then we divided the total count by the length of the array.
Now when running the above code, you will get the following output:
Probability of a number present in array is: 0.29
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn