How to Evaluate a Mathematical Expression in Java
- Evaluate a Mathematical Expression in Java
-
Solve a String Mathematical Expression Using
ScriptEngine
in Java
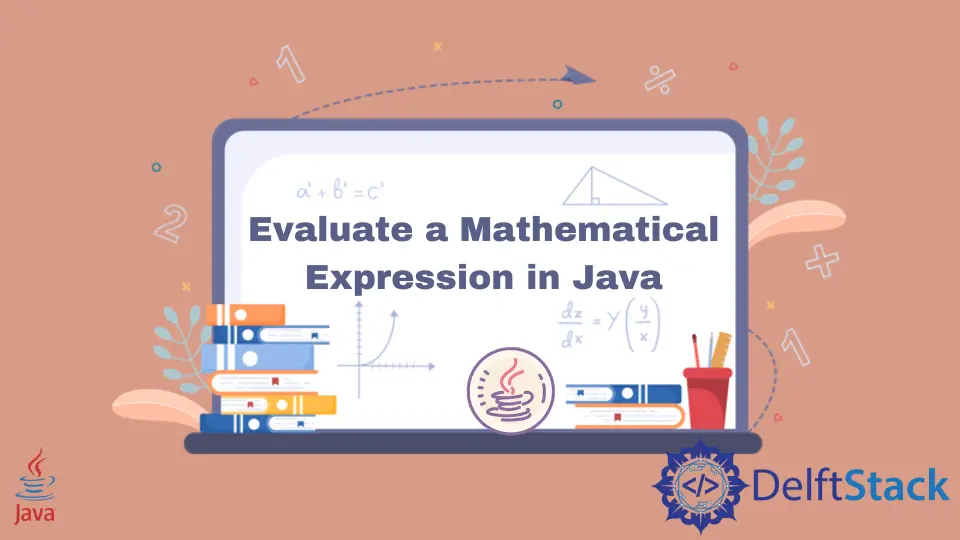
Evaluating a mathematical expression using a stack is one of the most common and useful options.
Stack has two standard methods, pop()
and push()
, used to put and get operands or operators from the stack. The pop()
method removes the top element of the expression, whereas the push()
method puts an element on the top of the stack.
Evaluate a Mathematical Expression in Java
Here is an example in Java to evaluate a mathematical expression. This code follows the proper DMAS rules with the following precedence: Division, Multiplication, Addition, and Subtraction.
You can give it any mathematical expression as input but ensure that the expression consists of the following four operations (Addition, Multiplication, Division, and Subtraction) only.
Example code:
package evaluateexpression;
import java.util.Scanner;
import java.util.Stack;
public class EvaluateExpression {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
// Creating stacks for operators and operands
Stack<Integer> operator = new Stack();
Stack<Double> value = new Stack();
// Let's create some temparory stacks for operands and operators
Stack<Integer> tmpOp = new Stack();
Stack<Double> tmpVal = new Stack();
// Enter an arthematic expression
System.out.println("Enter expression");
String input = scan.next();
System.out.println(
"The type of the expression is " + ((Object) input).getClass().getSimpleName());
input = "0" + input;
input = input.replaceAll("-", "+-");
// In the respective stacks store the operators and operands
String temp = "";
for (int i = 0; i < input.length(); i++) {
char ch = input.charAt(i);
if (ch == '-')
temp = "-" + temp;
else if (ch != '+' && ch != '*' && ch != '/')
temp = temp + ch;
else {
value.push(Double.parseDouble(temp));
operator.push((int) ch);
temp = "";
}
}
value.push(Double.parseDouble(temp));
// Create a character array for the operator precedence
char operators[] = {'/', '*', '+'};
/* Evaluation of expression */
for (int i = 0; i < 3; i++) {
boolean it = false;
while (!operator.isEmpty()) {
int optr = operator.pop();
double v1 = value.pop();
double v2 = value.pop();
if (optr == operators[i]) {
// if operator matches evaluate and store it in the temporary stack
if (i == 0) {
tmpVal.push(v2 / v1);
it = true;
break;
} else if (i == 1) {
tmpVal.push(v2 * v1);
it = true;
break;
} else if (i == 2) {
tmpVal.push(v2 + v1);
it = true;
break;
}
} else {
tmpVal.push(v1);
value.push(v2);
tmpOp.push(optr);
}
}
// pop all the elements from temporary stacks to main stacks
while (!tmpVal.isEmpty()) value.push(tmpVal.pop());
while (!tmpOp.isEmpty()) operator.push(tmpOp.pop());
// Iterate again for the same operator
if (it)
i--;
}
System.out.println("\nResult = " + value.pop());
}
}
Output:
Enter expression
2+7*5-3/2
The type of the expression is String
Result = 35.5
As you can see in the output of the above code, the expression 2+7*5-3/2
was given as input. And the program has calculated the result as 35.5
.
It first divided 3/2 = 1.5
because, in DMAS rules, the division has the highest precedence. Then the multiplication part is calculated as 7*5 = 35
.
Next, we have an addition of 2+35 = 37
, and the last part of the expression is a subtraction that is 37 -1.5 = 35.5
.
Solve a String Mathematical Expression Using ScriptEngine
in Java
In our example below, we illustrated how we could solve a mathematical expression in string format using the ScriptEngine
. Take a look at our below code:
import javax.script.ScriptEngine;
import javax.script.ScriptEngineManager;
import javax.script.ScriptException;
public class EvaluateExpLib {
public static void main(String[] args) throws ScriptException {
ScriptEngineManager MyMgr = new ScriptEngineManager(); // Declaring a "ScriptEngineManager"
ScriptEngine MathEng = MyMgr.getEngineByName("JavaScript"); // Declaring a "ScriptEngine"
String Exp = "300+2-3";
System.out.println("The result is: " + MathEng.eval(Exp));
}
}
We have commanded the purpose of each line. Now, after running the example code, you will see the below output:
The result is: 299
Remember, you may need JDK1.6 to run the above example.
Please note that the example codes shared in this article are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn