Bewerten einen mathematischen Ausdruck in Java
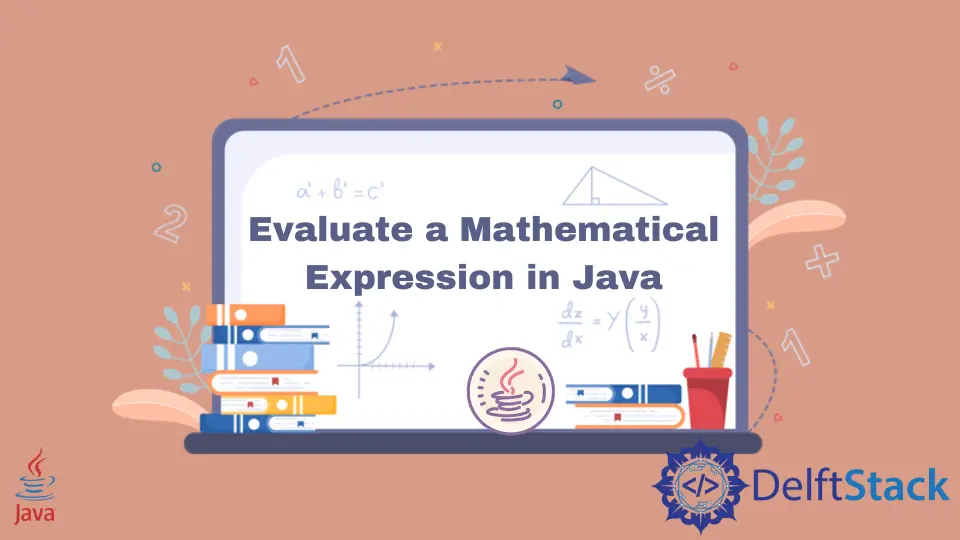
Das Auswerten eines mathematischen Ausdrucks mithilfe eines Stapels ist eine der häufigsten und nützlichsten Optionen.
Stack hat zwei Standardmethoden, pop()
und push()
, die verwendet werden, um Operanden oder Operatoren aus dem Stack zu legen und zu holen. Die Methode pop()
entfernt das oberste Element des Ausdrucks, während die Methode push()
ein Element ganz oben auf den Stapel legt.
Bewerten einen mathematischen Ausdruck in Java
Hier ist ein Beispiel in Java, um einen mathematischen Ausdruck auszuwerten. Dieser Code folgt den richtigen DMAS-Regeln mit der folgenden Priorität: Division, Multiplikation, Addition und Subtraktion.
Sie können ihm jeden mathematischen Ausdruck als Eingabe geben, aber stellen Sie sicher, dass der Ausdruck nur aus den folgenden vier Operationen (Addition, Multiplikation, Division und Subtraktion) besteht.
Beispielcode:
package evaluateexpression;
import java.util.Scanner;
import java.util.Stack;
public class EvaluateExpression {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
// Creating stacks for operators and operands
Stack<Integer> operator = new Stack();
Stack<Double> value = new Stack();
// Let's create some temparory stacks for operands and operators
Stack<Integer> tmpOp = new Stack();
Stack<Double> tmpVal = new Stack();
// Enter an arthematic expression
System.out.println("Enter expression");
String input = scan.next();
System.out.println(
"The type of the expression is " + ((Object) input).getClass().getSimpleName());
input = "0" + input;
input = input.replaceAll("-", "+-");
// In the respective stacks store the operators and operands
String temp = "";
for (int i = 0; i < input.length(); i++) {
char ch = input.charAt(i);
if (ch == '-')
temp = "-" + temp;
else if (ch != '+' && ch != '*' && ch != '/')
temp = temp + ch;
else {
value.push(Double.parseDouble(temp));
operator.push((int) ch);
temp = "";
}
}
value.push(Double.parseDouble(temp));
// Create a character array for the operator precedence
char operators[] = {'/', '*', '+'};
/* Evaluation of expression */
for (int i = 0; i < 3; i++) {
boolean it = false;
while (!operator.isEmpty()) {
int optr = operator.pop();
double v1 = value.pop();
double v2 = value.pop();
if (optr == operators[i]) {
// if operator matches evaluate and store it in the temporary stack
if (i == 0) {
tmpVal.push(v2 / v1);
it = true;
break;
} else if (i == 1) {
tmpVal.push(v2 * v1);
it = true;
break;
} else if (i == 2) {
tmpVal.push(v2 + v1);
it = true;
break;
}
} else {
tmpVal.push(v1);
value.push(v2);
tmpOp.push(optr);
}
}
// pop all the elements from temporary stacks to main stacks
while (!tmpVal.isEmpty()) value.push(tmpVal.pop());
while (!tmpOp.isEmpty()) operator.push(tmpOp.pop());
// Iterate again for the same operator
if (it)
i--;
}
System.out.println("\nResult = " + value.pop());
}
}
Ausgabe:
Enter expression
2+7*5-3/2
The type of the expression is String
Result = 35.5
Wie Sie in der Ausgabe des obigen Codes sehen können, wurde als Eingabe der Ausdruck 2+7*5-3/2
angegeben. Und das Programm hat das Ergebnis als 35.5
errechnet.
Es dividierte zunächst 3/2 = 1.5
, weil in den DMAS-Regeln die Division den höchsten Vorrang hat. Dann wird der Multiplikationsteil als 7*5 = 35
berechnet.
Als nächstes haben wir eine Addition von 2+35 = 37
, und der letzte Teil des Ausdrucks ist eine Subtraktion, die 37 -1.5 = 35.5
ist.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn