Java で 2 点間の距離を計算する
Sheeraz Gul
2023年10月12日
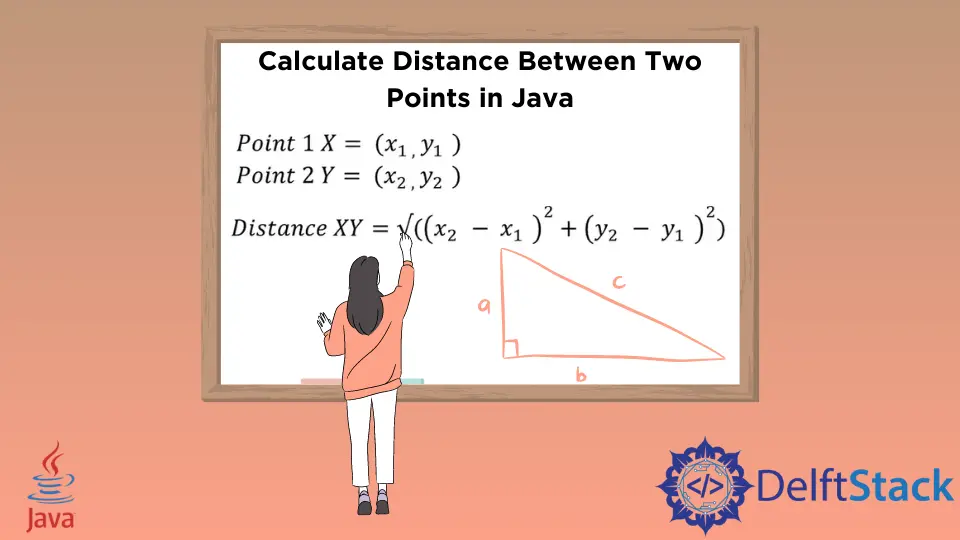
ピタゴリアンの定理を使用して、Java の 2 点間の距離を見つけることができます。このチュートリアルでは、Java で 2 点間の距離を計算する方法を示します。
Java で 2 点間の距離を計算する
たとえば、2つのポイント X
と Y
の座標は (x1, y1)
と (x2, y2)
であり、これら 2つのポイント間の距離は XY
と表すことができ、ピタゴリアンの定理距離を計算するために Java で実装できます。
下の写真の式は、これら 2つのポイントのピタゴリアンの定理を表しています。
ピタゴリアンの定理を Java で実装してみましょう。
package delftstack;
import java.util.Scanner;
public class Distance_Two_Points {
public static void main(String[] args) {
Scanner Temp = new Scanner(System.in);
// declare the variables
int x1;
int x2;
int y1;
int y2;
int x;
int y;
double Distance_Result;
// get the input coordinates
System.out.print("Enter the values of first point coordinates : ");
x1 = Temp.nextInt();
y1 = Temp.nextInt();
System.out.print("Enter the values of second point coordinates : ");
x2 = Temp.nextInt();
y2 = Temp.nextInt();
// Implement pythagorean theorem
x = x2 - x1;
y = y2 - y1;
Distance_Result = Math.sqrt(x * x + y * y);
System.out.println("Distance between the two points is : " + Distance_Result);
}
}
上記のコードは、2 点の座標を取り、ピタゴリアンの定理によって距離を計算します。出力を参照してください:
Enter the values of first point coordinates : 12
21
Enter the values of second point coordinates : 13
34
Distance between the two points is : 13.038404810405298
著者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook