Java で配列の中央値を計算する
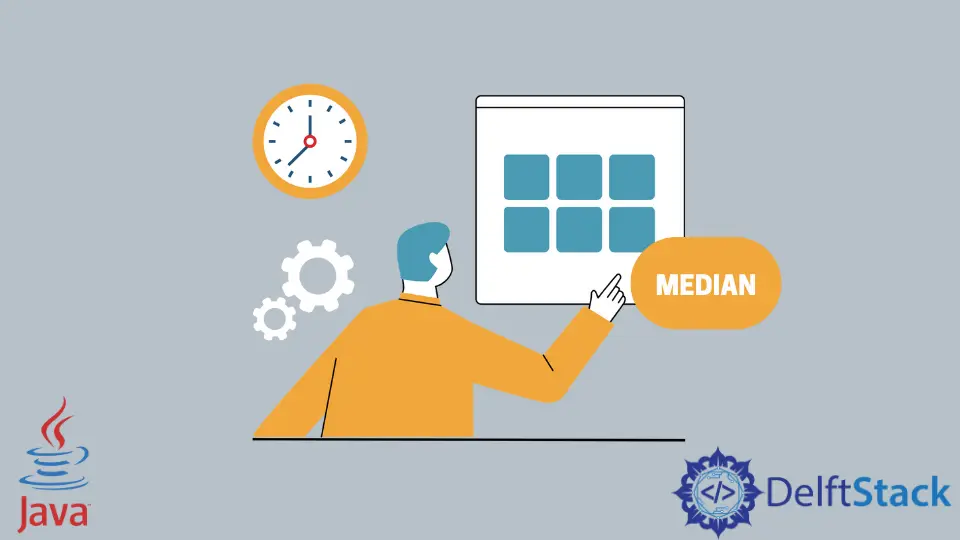
このチュートリアルでは、中央値と平均値と中央値の差を計算する方法を例示します。 また、中央値問題文の解き方も紹介します。
次に、Java プログラムを実行します。これは、簡単に言えば、Arrays.sort()
、length()
、および標準の中央値式を利用して中央の要素を決定します。
問題文の中央値を解く
中央値は、並べ替えられた一連の数値、リスト、またはデータ コレクションの中間値です。 系列は昇順または降順である場合があり、中央値は一連の数値の中央または中央にある数値です。
これは、すべてのデータ ポイントを並べ替えて、中央のデータ ポイントを選択することで見つかります。 中心値が 2つある場合は、それら 2つの数値の平均をとります。
たとえば、(6, 1, 9)
の中央値は 6
です。数字 6
は真ん中にあるからです。 ここでは、中央値を決定する方法について説明します。
数字のセットを注文すると (1, 6, 9)
が得られます。 数字 6
は真ん中の要素です。
中央値と平均値の違い
すべての要素の合計を計算することにより、データ セットの平均値 (平均) を決定します。 その後、それぞれのセットの値の数で割ります。
データセットを最小から最大の順に並べると、中央値は中間のままです。
Java で配列の中央値を計算する
配列の中心要素を計算する簡単な Java プログラムを実行します。 また、時間計算量をチェックして中央値を見つけます: O(n log n)
。
コード:
package delftstack.com.util;
// This program will show you the simplest method to calculate the median of an array.
// It is also called the central element of an array.
import java.util.Arrays;
// Main class
public class Example {
// This is our main function. It will find out the median.
public static double findMedian(int x[], int n)
// x = ordered list of data set values
// n = values in the data set
{
// Our array needs to be sorted before we find out its middle element (median)
Arrays.sort(x);
// Lets find out the even numbers
if (n % 2 != 0)
return (double) x[n / 2];
return (double) (x[(n - 1) / 2] + x[n / 2]) / 2.0;
}
// The following code will run our java application
public static void main(String args[]) {
int numseries[] = {1, 5, 6, 2, 9, 3, 11, 16};
// Determine the length of array (we are using length function)
int determine = numseries.length;
// Let us call the function here
System.out.println("The median of the given array is = " + findMedian(numseries, determine));
}
}
// main class ends here
// This article is authored by the team at delfstack.com
出力:
The median of the given array is = 5.5
前の方法に似ていますが、よりクリーンな次の方法を試すこともできます。
コード:
Arrays.sort(arrayname);
double middle;
if (a.length % 2 == 0)
median = ((double) a[a.length / 2] + (double) a[a.length / 2 - 1]) / 2;
else
median = (double) a[a.length / 2];
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn