How to Calculate Median of an Array in Java
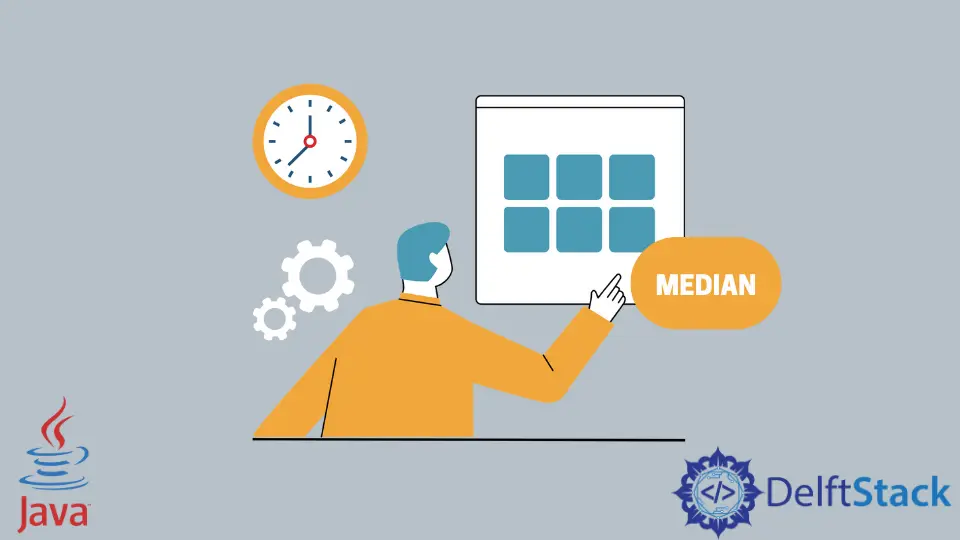
This tutorial will exemplify how to calculate the median and the difference between mean and median. Also, we will show you how to solve the median problem statement.
Then, we will run a Java program, which, in a nutshell, makes good use of Arrays.sort()
, length()
, and the standard median formula to determine the middle element.
Solve the Median Problem Statement
The median is the middle value in a sorted series of numbers, a list, or data collection. The series can be in ascending or descending order, in which the median is the number in the middle or center of a series of numbers.
It is found by sorting all data points and selecting the one in the middle. If there are two central values, take the mean of those two numbers.
For example, the median of (6, 1, 9)
is 6
because the number 6
is in the middle. Here is how we determine the median.
We get (1, 6, 9)
when you order the set of numbers. The number 6
is the middle element.
Difference Between Median and Mean
We determine a data set’s mean value (average) by calculating the sum of all its elements. After that, we divide it by the number of values in the respective set.
The median value remains in the middle when a data set is ordered from least to greatest.
Calculate Median of an Array in Java
We will run a simple Java program for you that calculates the central element of an array. Also, check the time complexity to find the median: O(n log n)
.
Code:
package delftstack.com.util;
// This program will show you the simplest method to calculate the median of an array.
// It is also called the central element of an array.
import java.util.Arrays;
// Main class
public class Example {
// This is our main function. It will find out the median.
public static double findMedian(int x[], int n)
// x = ordered list of data set values
// n = values in the data set
{
// Our array needs to be sorted before we find out its middle element (median)
Arrays.sort(x);
// Lets find out the even numbers
if (n % 2 != 0)
return (double) x[n / 2];
return (double) (x[(n - 1) / 2] + x[n / 2]) / 2.0;
}
// The following code will run our java application
public static void main(String args[]) {
int numseries[] = {1, 5, 6, 2, 9, 3, 11, 16};
// Determine the length of array (we are using length function)
int determine = numseries.length;
// Let us call the function here
System.out.println("The median of the given array is = " + findMedian(numseries, determine));
}
}
// main class ends here
// This article is authored by the team at delfstack.com
Output:
The median of the given array is = 5.5
You can also try the following method, similar to the previous one but cleaner.
Code:
Arrays.sort(arrayname);
double middle;
if (a.length % 2 == 0)
median = ((double) a[a.length / 2] + (double) a[a.length / 2 - 1]) / 2;
else
median = (double) a[a.length / 2];
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn