Java에서 배열의 중앙값 계산
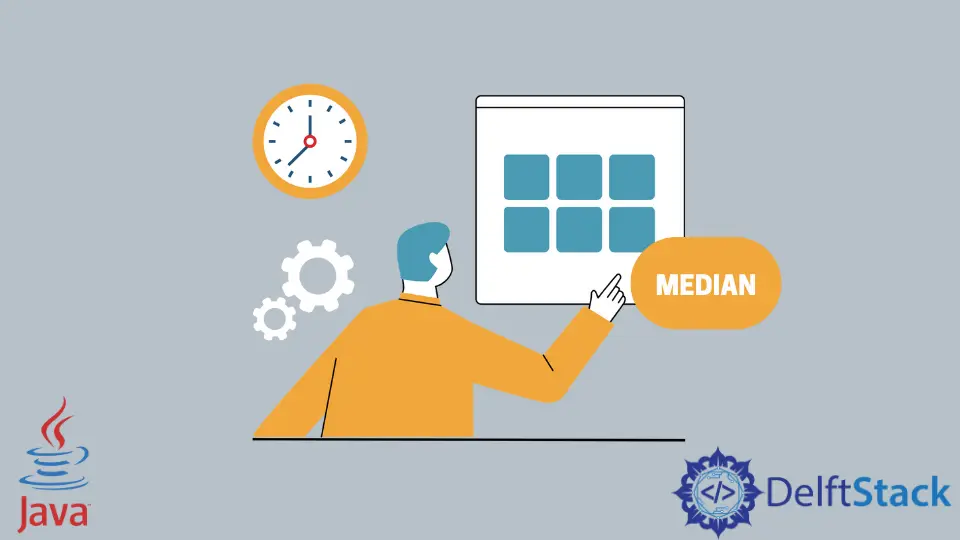
이 자습서에서는 중앙값과 평균과 중앙값의 차이를 계산하는 방법을 예시합니다. 또한 중앙값 문제 진술을 해결하는 방법을 보여드리겠습니다.
그런 다음 간단히 말해서 Arrays.sort()
, length()
및 중간 요소를 결정하는 표준 중간 수식을 잘 활용하는 Java 프로그램을 실행합니다.
중앙값 문제 설명 풀기
중앙값은 정렬된 일련의 숫자, 목록 또는 데이터 컬렉션의 중간 값입니다. 계열은 오름차순 또는 내림차순일 수 있으며 중앙값은 일련의 숫자 가운데 또는 중앙에 있는 숫자입니다.
모든 데이터 포인트를 정렬하고 중간에 있는 것을 선택하면 찾을 수 있습니다. 두 개의 중심 값이 있는 경우 해당 두 숫자의 평균을 취하십시오.
예를 들어 (6, 1, 9)
의 중앙값은 숫자 6
이 중간에 있기 때문에 6
입니다. 중앙값을 결정하는 방법은 다음과 같습니다.
숫자 집합을 주문하면 (1, 6, 9)
가 표시됩니다. 숫자 6
은 중간 요소입니다.
중앙값과 평균의 차이점
모든 요소의 합계를 계산하여 데이터 세트의 평균값(average)을 결정합니다. 그런 다음 각 세트의 값 수로 나눕니다.
데이터 세트가 최소에서 최대로 정렬될 때 중앙값은 중간에 유지됩니다.
Java에서 배열의 중앙값 계산
배열의 중심 요소를 계산하는 간단한 Java 프로그램을 실행할 것입니다. 또한 중앙값을 찾기 위해 시간 복잡도를 확인하십시오: O(n log n)
.
암호:
package delftstack.com.util;
// This program will show you the simplest method to calculate the median of an array.
// It is also called the central element of an array.
import java.util.Arrays;
// Main class
public class Example {
// This is our main function. It will find out the median.
public static double findMedian(int x[], int n)
// x = ordered list of data set values
// n = values in the data set
{
// Our array needs to be sorted before we find out its middle element (median)
Arrays.sort(x);
// Lets find out the even numbers
if (n % 2 != 0)
return (double) x[n / 2];
return (double) (x[(n - 1) / 2] + x[n / 2]) / 2.0;
}
// The following code will run our java application
public static void main(String args[]) {
int numseries[] = {1, 5, 6, 2, 9, 3, 11, 16};
// Determine the length of array (we are using length function)
int determine = numseries.length;
// Let us call the function here
System.out.println("The median of the given array is = " + findMedian(numseries, determine));
}
}
// main class ends here
// This article is authored by the team at delfstack.com
출력:
The median of the given array is = 5.5
이전 방법과 비슷하지만 더 깔끔한 다음 방법을 시도해 볼 수도 있습니다.
암호:
Arrays.sort(arrayname);
double middle;
if (a.length % 2 == 0)
median = ((double) a[a.length / 2] + (double) a[a.length / 2 - 1]) / 2;
else
median = (double) a[a.length / 2];
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn