How to Get the Last Character of a String in Java
-
Get the Last Character of a String in Java Using
substring()
-
Get the Last Character of a String in Java Using
charAt()
- Get the Last Character of a String in Java by Converting the String to a Char Array
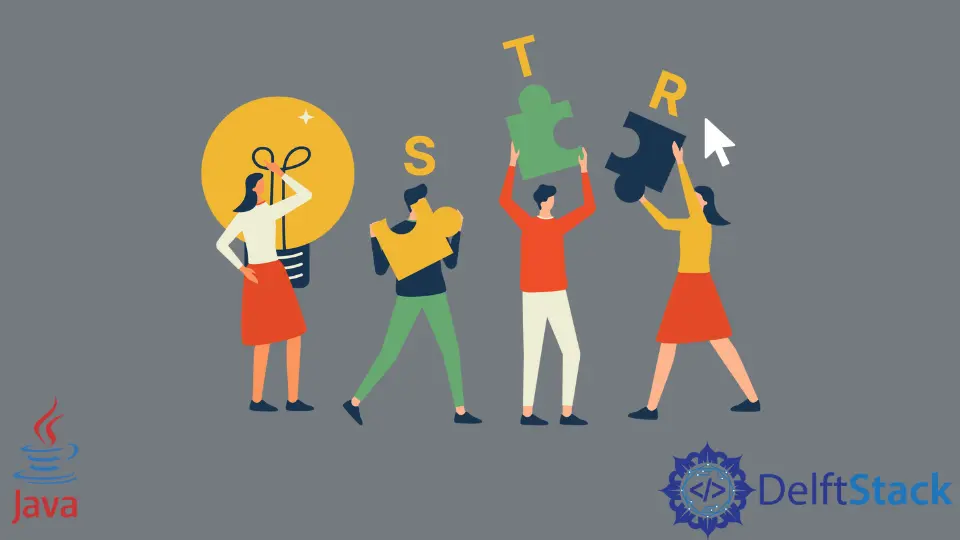
This tutorial will introduce how we can get the last character of the string in Java.
Get the Last Character of a String in Java Using substring()
In the below example code, the last character of exampleString
is g
. We will use a method of the String
class called substring()
that takes out a substring as its name suggests. The substring(startingIndex)
method takes an argument which should be the index or position of the part of the string that we need. We only need the last character, so we pass exampleString.length() - 1
as the starting index.
Now we have the last character in the lastCharacter
variable as a string. We need to have our character as a char
so that we can use toCharArray()
to convert the lastCharacter
to an array of char
and then get the only single character in that array.
public class LastCharString {
public static void main(String[] args) {
String exampleString = "This is a String";
String lastCharacter = exampleString.substring(exampleString.length() - 1);
char[] lastChar = lastCharacter.toCharArray();
System.out.println("Last char: " + lastChar[lastChar.length - 1]);
}
}
Output:
Last char: g
Get the Last Character of a String in Java Using charAt()
Instead of getting the last character as a string and then convert it to a char[]
, we can directly get the last character in a string by using the chartAt()
method of the String
class. This method lets us get a specific single character at the specified index number.
exampleString.charAt(exampleString.length() - 1)
is where we get the character located at the last position of exampleString
.
public class LastCharString {
public static void main(String[] args) {
String exampleString = "This is a String";
char lastChar = exampleString.charAt(exampleString.length() - 1);
System.out.println("Last char: " + lastChar);
}
}
Output:
Last char: g
Get the Last Character of a String in Java by Converting the String to a Char Array
Another short method that we can use is to directly convert the exampleString
into an array of char
. It makes the code more concise and faster. We can use the toCharArray()
method, which we used in this article’s first example, to convert the whole string to a char array. Then we get the last character by accessing the array with the last character’s index - lastCharArray.length - 1
.
public class LastCharString {
public static void main(String[] args) {
String exampleString = "This is a String";
char[] lastCharArray = exampleString.toCharArray();
char lastChar = lastCharArray[lastCharArray.length - 1];
System.out.println("Last char: " + lastChar);
}
}
Output:
Last char: g
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Char
- How to Convert Int to Char in Java
- Char vs String in Java
- How to Initialize Char in Java
- How to Represent Empty Char in Java
- How to Convert Char to Uppercase/Lowercase in Java
- How to Check if a Character Is Alphanumeric in Java