How to Create an Array of Objects in Java
- Create an Array of Objects and Initialize Objects Using a Constructor in Java
-
Create an Array of Objects and Initialize the Objects Calling the Constructor Using the
{}
Array Notation in Java - Declare an Array of Objects With Initial Values in Java
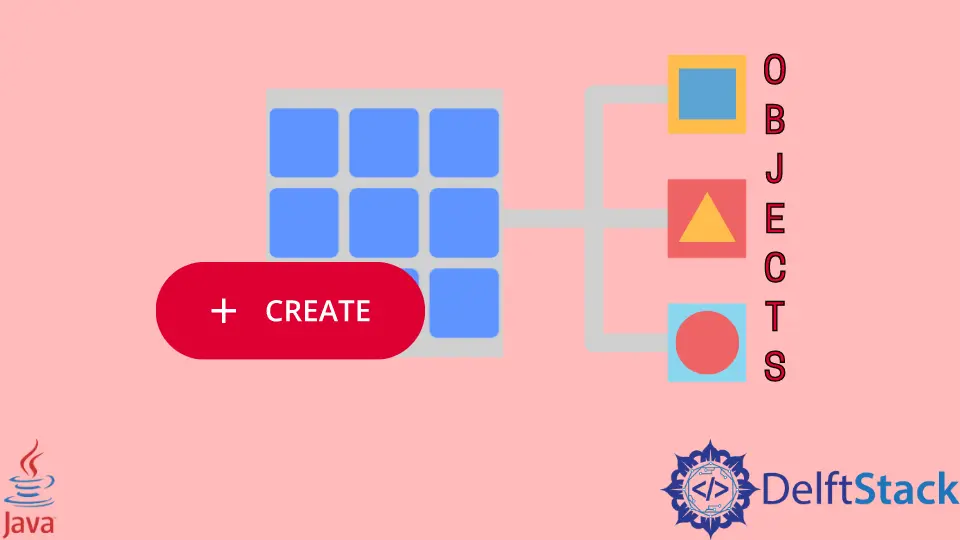
This article will introduce methods to create an array of objects in Java. The article will also demonstrate the instantiation of objects and their implementation.
Create an Array of Objects and Initialize Objects Using a Constructor in Java
Java is an object-oriented programming language, and it consists of classes and objects. We can create an array of an object using the []
array notation in Java. We can use the constructor to initialize the objects by passing the values to it. The syntax of the expression is shown below.
Type[] objectName = new ClassName[];
The Type
denotes the type of the object. It may be of a specific data type or a class type. The []
symbol after the type resembles that we are creating an array. The option objectName
refers to the name of the object. The new
operator creates an instance. The ClassName
refers to the name of the class whose object is made. We can specify the size of the array in the []
after the class. We can use the index in the array to instantiate each object.
For example, we have a class named Customer
:
- Create a class
Store
to write the main method to it. Inside the main method, create an arrayarr
of theCustomer
type and allocate the memory for twoCustomer
classes’ objects. - Create two objects of the
Customer
class from the indexed arrayarr
. - Supply the values
709270
andRobert
for the first object and709219
andNeal
for the second object while creating the object.
These values are the parameters for the constructor of the Customer
class. Then call the display()
function with the created objects.
The Customer
class contains the public properties id
and name
. The constructor of the class sets the values for these properties. The public function display()
displays the property of the class. In the example below, the objects are created from an array, and the constructor is invoked during the creation of the object. Then, the objects call the display()
function, and the output is displayed. So far, we have learned how to create an array of objects and use it with the methods.
Example Code:
public class Store {
public static void main(String args[]) {
Customer[] arr = new Customer[2];
arr[0] = new Customer(709270, "Robert");
arr[1] = new Customer(709219, "Neal");
arr[0].display();
arr[1].display();
}
}
class Customer {
public int id;
public String name;
Customer(int id, String name) {
this.id = id;
this.name = name;
}
public void display() {
System.out.println("Customer id is: " + id + " "
+ "and Customer name is: " + name);
}
}
Output:
Customer id is: 709270 and Customer name is: Robert
Customer id is: 709219 and Customer name is: Neal
Create an Array of Objects and Initialize the Objects Calling the Constructor Using the {}
Array Notation in Java
In the second method, we will create an array of objects as we did in the first method. That is, we will be using the constructor to instantiate the objects. But we will use a single-line approach to instantiate the objects. We will call the constructor the time we create the array to hold the objects. We can write the constructor call in a single line inside the {}
array notation. We will create the objects of the Customer
class in the Store
class.
For example, create an array arr
as in the first method. But instead of allocating the memory for the objects, create the objects in the same line. Write an array {}
notation after the new Customer[]
. Next, create two objects of the Customer
class with the new
keyword. Supply the respective id
and name
as the parameters to the constructor. Use a comma to separate each constructor call.
Example code:
public class Store {
public static void main(String args[]) {
Customer[] arr = new Customer[] {new Customer(709270, "Robert"), new Customer(709219, "Neal")};
arr[0].display();
arr[1].display();
}
}
class Customer {
public int id;
public String name;
Customer(int id, String name) {
this.id = id;
this.name = name;
}
public void display() {
System.out.println("Customer id is: " + id + " "
+ "and Customer name is: " + name);
}
}
Output:
Customer id is: 703270 and Customer name is: Sushant
Customer id is: 703219 and Customer name is: Simanta
Declare an Array of Objects With Initial Values in Java
In the third method of creating an array of objects in Java, we will declare an array of objects providing the initial values. We will not create another class object in this approach. So, there will be no use of the constructor in this method. We will use the array {}
notation to write the array of objects. We will use the Object
type to create the array of objects.
For example, create a class and write the main method. Then, create an array arr
of the Object
type. Write the objects inside the {}
notation. The objects are CRF
, a string value, an instance of the Integer
class with the value of 2020
, Husky
another string, and another instance of the Integer
class with value 2017
. Finally, print each of the objects using the array indexing method.
Example Code:
class Motorcycle {
public static void main(String args[]) {
Object[] arr = {"CRF", new Integer(2020), "Husky", new Integer(2017)};
System.out.println(arr[0]);
System.out.println(arr[1]);
System.out.println(arr[2]);
System.out.println(arr[3]);
}
}
Output:
CRF
2020
Husky
2017
Related Article - Java Object
- How to Parse XML to Java Object
- How to Serialize Object to JSON in Java
- How to Serialize Object to String in Java
- How to Implement Data Access Object in Java
- How to Sort an Array of Objects in Java
- How to Print Objects in Java