How to Parse XML to Java Object
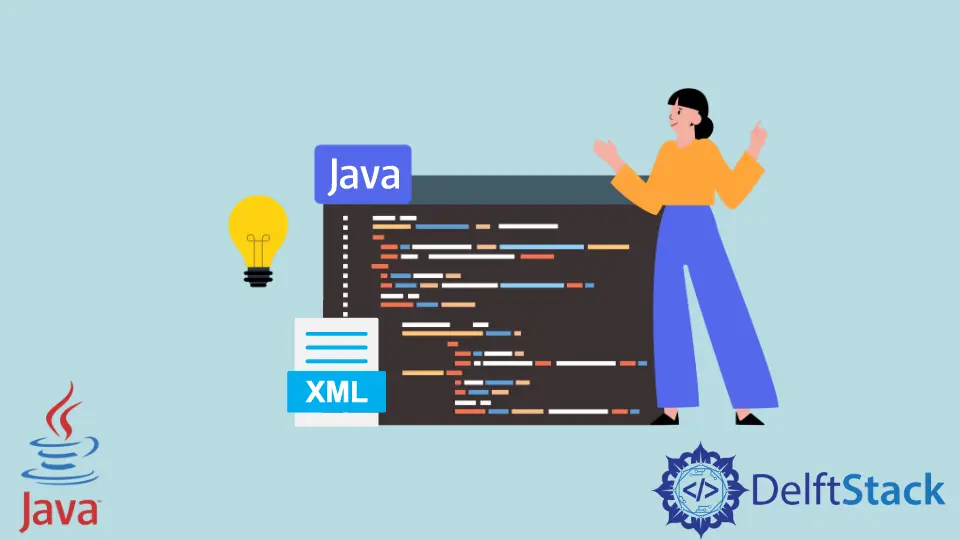
XML is the most widely used technology to store, modify and share data for any software environment. When an XML file arrives, our first job is to parse it to work on it.
This article will teach us about parsing XML in Java and converting it to an object.
Parse XML to Java Object
When talking about parsing XML files in Java, some common and most used parsers are available in Java. The DOM Parser
, SAX Parser
, and StAX Parser
are the most popular.
We are going to see an example for each of these XML parsers. But, before starting, suppose we have an XML file like the one below.
<?xml version = "1.0"?>
<class>
<person ID = "293">
<firstname>Alen</firstname>
<lastname>Walker</lastname>
<email>alen@email.com</email>
</person>
<person ID = "393">
<firstname>Stefen</firstname>
<lastname>Dev</lastname>
<email>stefen@email.com</email>
</person>
<person ID = "493">
<firstname>John</firstname>
<lastname>Doe</lastname>
<email>john@email.com</email>
</person>
</class>
Method 1: Use DOM Parser
to Parse XML to Java Object
Example Code:
// importing necessary files
import java.io.File;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
public class JavaXMLParsing {
public static void main(String[] args) {
try {
// Locating the file
File MyFile = new File("G:\\Order 011\\BATCH\\Myxml.xml");
// Creating a document object document builder.
DocumentBuilderFactory DBF = DocumentBuilderFactory.newInstance();
DocumentBuilder DB = DBF.newDocumentBuilder();
Document DOC = DB.parse(MyFile);
DOC.getDocumentElement().normalize();
// Printing the root element
System.out.println("Root element: " + DOC.getDocumentElement().getNodeName());
// Find the primary node
NodeList NodeList = DOC.getElementsByTagName("person");
// Extracting data from child node
for (int itr = 0; itr < NodeList.getLength(); itr++) {
Node MyNode = NodeList.item(itr);
System.out.println("\nNode Name:" + MyNode.getNodeName());
if (MyNode.getNodeType() == Node.ELEMENT_NODE) {
Element element = (Element) MyNode;
System.out.println(
"First Name: " + element.getElementsByTagName("firstname").item(0).getTextContent());
System.out.println(
"Last Name: " + element.getElementsByTagName("lastname").item(0).getTextContent());
System.out.println(
"Email: " + element.getElementsByTagName("email").item(0).getTextContent());
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Root element: class
Node Name: person
First Name: Alen
Last Name: Walker
Email: alen@email.com
Node Name: person
First Name: Stefen
Last Name: Dev
Email: stefen@email.com
Node Name: person
First Name: John
Last Name: Doe
Email: john@email.com
Remember you must download the DOM Parser
jar file before working with it. You can get it from here.
Method 2: Use SAX Parser
to Parse XML to Java Object
Example Code:
// importing necessary files
import javax.xml.parsers.SAXParser;
import javax.xml.parsers.SAXParserFactory;
import org.xml.sax.Attributes;
import org.xml.sax.SAXException;
import org.xml.sax.helpers.DefaultHandler;
public class JavaSAXParser {
public static void main(String argv[]) {
try {
// Organizing a SAXParser
SAXParserFactory MyFactory = SAXParserFactory.newInstance();
SAXParser SaxParser = MyFactory.newSAXParser();
// Creating a default handler
DefaultHandler MyHandler = new DefaultHandler() {
boolean firstname = false;
boolean lastname = false;
boolean email = false;
// Extracting data from the XML
public void startElement(String uri, String localName, String qName, Attributes attributes)
throws SAXException {
if (qName.equalsIgnoreCase("firstname")) {
firstname = true;
}
if (qName.equalsIgnoreCase("lastname")) {
lastname = true;
}
if (qName.equalsIgnoreCase("email")) {
email = true;
}
}
public void characters(char ch[], int start, int length) throws SAXException {
if (firstname) {
System.out.println("First Name: " + new String(ch, start, length));
firstname = false;
}
if (lastname) {
System.out.println("Last Name: " + new String(ch, start, length));
lastname = false;
}
if (email) {
System.out.println("Email: " + new String(ch, start, length));
email = false;
}
}
};
SaxParser.parse("G:\\Order 011\\BATCH\\Myxml.xml", MyHandler);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
First Name: Alen
Last Name: Walker
Email: alen@email.com
First Name: Stefen
Last Name: Dev
Email: stefen@email.com
First Name: John
Last Name: Doe
Email: john@email.com
Method 3: Use StAX Parser
to Parse XML to Java Object
Example Code:
// importing necessary files
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.util.Iterator;
import javax.xml.namespace.QName;
import javax.xml.stream.XMLEventReader;
import javax.xml.stream.XMLInputFactory;
import javax.xml.stream.XMLStreamException;
import javax.xml.stream.events.*;
public class JavaSTAXparser {
private static boolean firstname, lastname, email;
public static void main(String[] args) throws FileNotFoundException, XMLStreamException {
// Locating the file
File file = new File("G:\\Order 011\\BATCH\\Myxml.xml");
// Parsing the file with the parse() method
parser(file);
}
// The parse() method
public static void parser(File file) throws FileNotFoundException, XMLStreamException {
firstname = lastname = email = false;
// Declaring a XMLInputFactory object
XMLInputFactory MyFactory = XMLInputFactory.newInstance();
// Declaring a XMLEventReader object
XMLEventReader EventReader = MyFactory.createXMLEventReader(new FileReader(file));
while (EventReader.hasNext()) {
// Creating a xml event
XMLEvent Event = EventReader.nextEvent();
if (Event.isStartElement()) {
// Extracting data from the xml file
StartElement Element = (StartElement) Event;
Iterator<Attribute> iterator = Element.getAttributes();
while (iterator.hasNext()) {
Attribute attribute = iterator.next();
QName name = attribute.getName();
String value = attribute.getValue();
System.out.println(name + " = " + value);
}
if (Element.getName().toString().equalsIgnoreCase("firstname")) {
firstname = true;
}
if (Element.getName().toString().equalsIgnoreCase("lastname")) {
lastname = true;
}
if (Element.getName().toString().equalsIgnoreCase("email")) {
email = true;
}
}
if (Event.isEndElement()) {
EndElement Element = (EndElement) Event;
if (Element.getName().toString().equalsIgnoreCase("firstname")) {
firstname = false;
}
if (Element.getName().toString().equalsIgnoreCase("lastname")) {
lastname = false;
}
if (Element.getName().toString().equalsIgnoreCase("email")) {
email = false;
}
}
if (Event.isCharacters()) {
Characters Element = (Characters) Event;
if (firstname) {
System.out.println(Element.getData());
}
if (lastname) {
System.out.println(Element.getData());
}
if (email) {
System.out.println(Element.getData());
}
}
}
}
}
Output:
ID = 293
Alen
Walker
alen@email.com
ID = 393
Stefen
Dev
stefen@email.com
ID = 493
John
Doe
john@email.com
Above, we discussed three different methods of parsing XML to Java objects and extracting data from it. You can select any of them based on your need.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Java XML
- How to Pretty Print XML in Java
- How to Write XML in Java
- How to Convert JSON to XML in Java
- How to Convert XML to JSON in Java