How to Convert JSON to XML in Java
- Convert JSON to XML using org.json Library in Java
- Convert JSON to XML using underscore Library in Java
- Convert JSON to XML using Jackson Library in Java
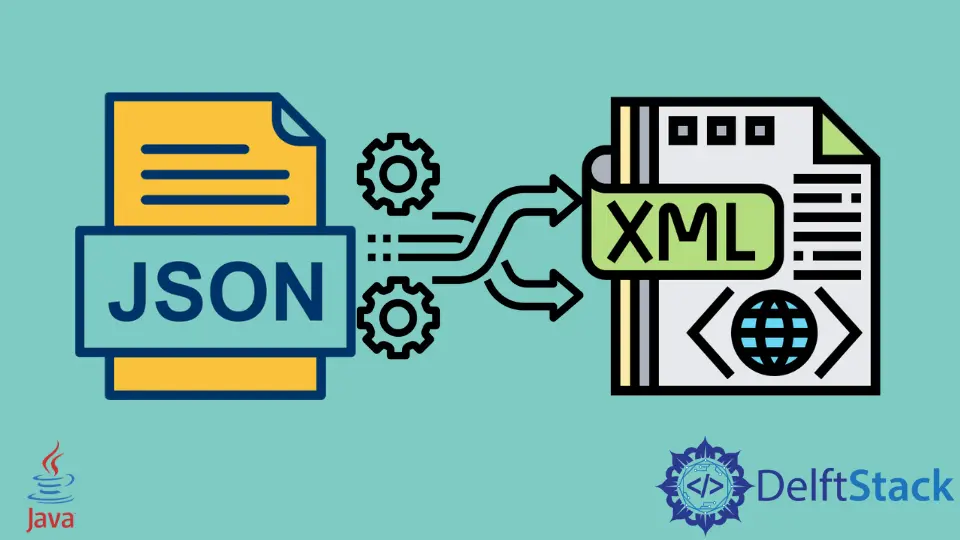
This tutorial introduces how to convert JSON to XML in Java.
To convert JSON to XML, we can use several libraries such as org.json, underscore, and the Jackson. In this article, we will learn to use these libraries and see the conversion process of JSON to XML.
JSON is a data format used by the application for data interchange. It is used by the applications because of lightweight and simple whereas XML is a markup language and is also used to transfer data.
Let’s start with some examples to convert the JSON to XML in Java.
Convert JSON to XML using org.json Library in Java
In this example, we used the org.json library that provides JSONObject
and an XML class. The JSONObject class is used to make a JSON string to a JSON object, and then by using the XML class, we converted the JSON to XML.
The XML class provides a static toString() method that returns the result as a String. See the example below.
import java.io.IOException;
import org.json.*;
public class SimpleTesting {
public static void main(String[] args) throws IOException, JSONException {
String jsonStr = "{student : { age:30, name : Kumar, technology : Java } }";
JSONObject json = new JSONObject(jsonStr);
String xml = XML.toString(json);
System.out.println(xml);
}
}
Output:
<student>
<name>Kumar</name>
<technology>Java</technology>
<age>30</age>
</student>
Convert JSON to XML using underscore Library in Java
Here, we used the underscore
library to convert JSON
to XML
. We used U
class and its static method jsonToXml()
that returns XML
as a string. See the example below.
import com.github.underscore.lodash.U;
import java.io.IOException;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
String jsonStr =
"{\"name\":\"JSON\",\"integer\":1,\"double\":2.0,\"boolean\":true,\"nested\":{\"id\":42},\"array\":[1,2,3]}";
System.out.println(jsonStr);
String xml = U.jsonToXml(jsonStr);
System.out.println(xml);
}
}
Output:
{"name":"JSON","integer":1,"double":2.0,"boolean":true,"nested":{"id":42},"array":[1,2,3]}
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>JSON</name>
<integer number="true">1</integer>
<double number="true">2.0</double>
<boolean boolean="true">true</boolean>
<nested>
<id number="true">42</id>
</nested>
<array number="true">1</array>
<array number="true">2</array>
<array number="true">3</array>
</root>
Convert JSON to XML using Jackson Library in Java
Here, we used the ObjectMapper
class to read JSON and then the XmlMapper
class to get XML format data. Here, we used two major packages, jackson.databind
and jackson.dataformat
; the first contains classes related to JSON, and the second includes classes related to XML. See the example below.
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.ser.ToXmlGenerator;
import java.io.IOException;
import java.io.StringWriter;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
final String jsonStr =
"{\"name\":\"JSON\",\"integer\":1,\"double\":2.0,\"boolean\":true,\"nested\":{\"id\":42},\"array\":[1,2,3]}";
ObjectMapper jsonMapper = new ObjectMapper();
JsonNode node = jsonMapper.readValue(jsonStr, JsonNode.class);
XmlMapper xmlMapper = new XmlMapper();
xmlMapper.configure(SerializationFeature.INDENT_OUTPUT, true);
xmlMapper.configure(ToXmlGenerator.Feature.WRITE_XML_DECLARATION, true);
xmlMapper.configure(ToXmlGenerator.Feature.WRITE_XML_1_1, true);
StringWriter sw = new StringWriter();
xmlMapper.writeValue(sw, node);
System.out.println(sw.toString());
}
}
Output:
{"name":"JSON","integer":1,"double":2.0,"boolean":true,"nested":{"id":42},"array":[1,2,3]}
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>JSON</name>
<integer number="true">1</integer>
<double number="true">2.0</double>
<boolean boolean="true">true</boolean>
<nested>
<id number="true">42</id>
</nested>
<array number="true">1</array>
<array number="true">2</array>
<array number="true">3</array>
</root>
Related Article - Java JSON
- How to Pretty-Print JSON Data in Java
- How to Serialize Object to JSON in Java
- How to Convert JSON Data to String in Java
- How to Deserialize JSON in Java
- How to Handle JSON Arrays in Java
- How to Convert JSON to Java object