在 Java 中將 JSON 轉換為 XML
Mohammad Irfan
2023年10月12日
Java
Java JSON
Java XML
- 使用 Java 中的 org.json 庫將 JSON 轉換為 XML
-
使用 Java 中的
underscore
庫將 JSON 轉換為 XML - 使用 Java 中的 Jackson 庫將 JSON 轉換為 XML
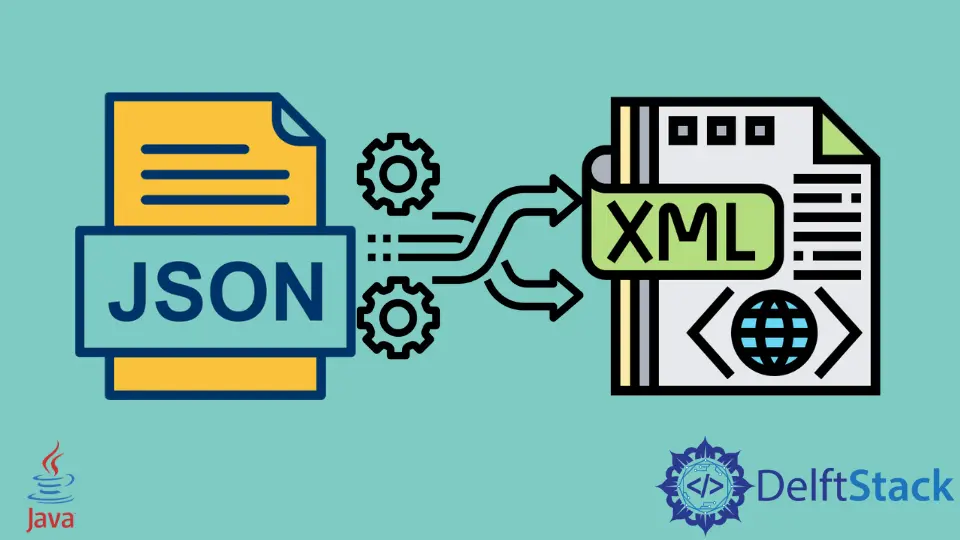
本教程介紹如何在 Java 中將 JSON 轉換為 XML。
要將 JSON 轉換為 XML,我們可以使用多個庫,例如 org.json、下劃線和 Jackson。在本文中,我們將學習使用這些庫並檢視 JSON 到 XML 的轉換過程。
JSON 是應用程式用於資料交換的資料格式。由於輕量級和簡單,它被應用程式使用,而 XML 是一種標記語言,也用於傳輸資料。
讓我們從一些在 Java 中將 JSON 轉換為 XML 的示例開始。
使用 Java 中的 org.json 庫將 JSON 轉換為 XML
在這個例子中,我們使用了 org.json 庫,它提供了 JSONObject
和一個 XML 類。JSONObject 類用於將 JSON 字串轉換為 JSON 物件,然後通過使用 XML 類將 JSON 轉換為 XML。
XML 類提供了一個靜態的 toString() 方法,該方法將結果作為字串返回。請參閱下面的示例。
import java.io.IOException;
import org.json.*;
public class SimpleTesting {
public static void main(String[] args) throws IOException, JSONException {
String jsonStr = "{student : { age:30, name : Kumar, technology : Java } }";
JSONObject json = new JSONObject(jsonStr);
String xml = XML.toString(json);
System.out.println(xml);
}
}
輸出:
<student>
<name>Kumar</name>
<technology>Java</technology>
<age>30</age>
</student>
使用 Java 中的 underscore
庫將 JSON 轉換為 XML
在這裡,我們使用 underscore
庫將 JSON
轉換為 XML
。我們使用了 U
類及其靜態方法 jsonToXml()
,它以字串形式返回 XML
。請參閱下面的示例。
import com.github.underscore.lodash.U;
import java.io.IOException;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
String jsonStr =
"{\"name\":\"JSON\",\"integer\":1,\"double\":2.0,\"boolean\":true,\"nested\":{\"id\":42},\"array\":[1,2,3]}";
System.out.println(jsonStr);
String xml = U.jsonToXml(jsonStr);
System.out.println(xml);
}
}
輸出:
{"name":"JSON","integer":1,"double":2.0,"boolean":true,"nested":{"id":42},"array":[1,2,3]}
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>JSON</name>
<integer number="true">1</integer>
<double number="true">2.0</double>
<boolean boolean="true">true</boolean>
<nested>
<id number="true">42</id>
</nested>
<array number="true">1</array>
<array number="true">2</array>
<array number="true">3</array>
</root>
使用 Java 中的 Jackson 庫將 JSON 轉換為 XML
在這裡,我們使用 ObjectMapper
類讀取 JSON,然後使用 XmlMapper
類獲取 XML 格式的資料。在這裡,我們使用了兩個主要的包,jackson.databind
和 jackson.dataformat
;第一個包含與 JSON 相關的類,第二個包含與 XML 相關的類。請參閱下面的示例。
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.fasterxml.jackson.dataformat.xml.XmlMapper;
import com.fasterxml.jackson.dataformat.xml.ser.ToXmlGenerator;
import java.io.IOException;
import java.io.StringWriter;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
final String jsonStr =
"{\"name\":\"JSON\",\"integer\":1,\"double\":2.0,\"boolean\":true,\"nested\":{\"id\":42},\"array\":[1,2,3]}";
ObjectMapper jsonMapper = new ObjectMapper();
JsonNode node = jsonMapper.readValue(jsonStr, JsonNode.class);
XmlMapper xmlMapper = new XmlMapper();
xmlMapper.configure(SerializationFeature.INDENT_OUTPUT, true);
xmlMapper.configure(ToXmlGenerator.Feature.WRITE_XML_DECLARATION, true);
xmlMapper.configure(ToXmlGenerator.Feature.WRITE_XML_1_1, true);
StringWriter sw = new StringWriter();
xmlMapper.writeValue(sw, node);
System.out.println(sw.toString());
}
}
輸出:
{"name":"JSON","integer":1,"double":2.0,"boolean":true,"nested":{"id":42},"array":[1,2,3]}
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>JSON</name>
<integer number="true">1</integer>
<double number="true">2.0</double>
<boolean boolean="true">true</boolean>
<nested>
<id number="true">42</id>
</nested>
<array number="true">1</array>
<array number="true">2</array>
<array number="true">3</array>
</root>
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe