How to Serialize Object to JSON in Java
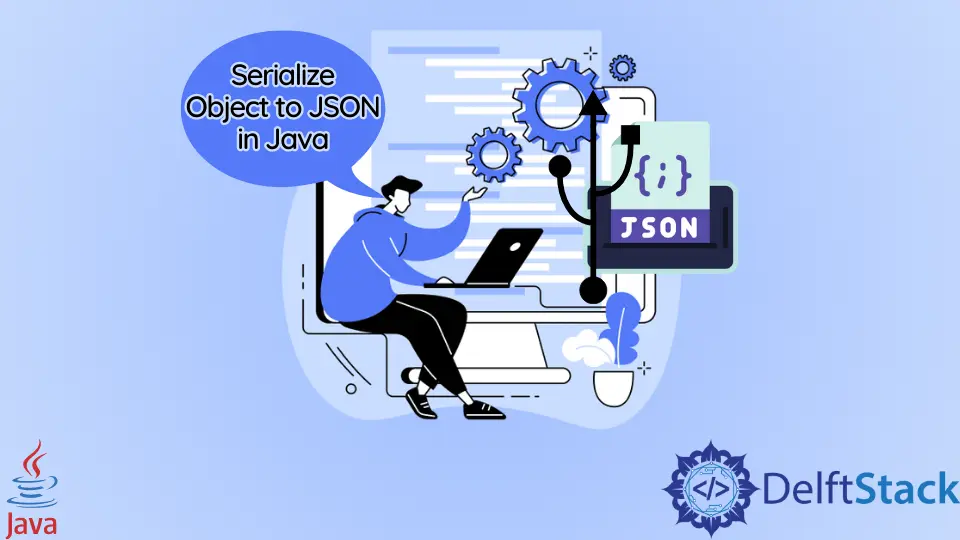
This tutorial demonstrates the use of Java-JSON
and Jackson
API to serialize an object to JSON in Java.
Serialize Object to JSON in Java
There are different libraries provided out there which are used for JSON operations. These libraries can also serialize Java objects to JSON using Java programming.
Use Java-JSON
API to Serialize Java Object to JSON
The Java-JSON
is a third-party API to perform JSON operations in Java, as Java doesn’t support JSON in the built-in mode. This library can serialize Java objects to JSON.
The Java-JSON
comes with different features and is better than many similar libraries. The main features are:
- Backward Compatibility.
- Consistent and Reliable Results.
- It has fast execution and a low memory footprint.
- There are no external dependencies.
- It provides adherence to the JSON specification.
Before using this API, you must add it to your build path. It can be easily done using the Gradle project dependency:
implementation 'org.json:json:20210307'
You can also add it to your general Java project by downloading the jar
file of this API from here and add it to your project’s build path.
The Java-JSON
provides a class JSONWriter
which we can use to serialize Java data into a JSON object. Let’s try an example:
package delftstack;
import java.io.StringWriter;
import java.io.Writer;
import org.json.JSONException;
import org.json.JSONWriter;
public class Example {
public static void main(String[] args) throws JSONException {
Writer DemoUser = new StringWriter();
var JSON_Writer = new JSONWriter(DemoUser);
JSON_Writer.object();
JSON_Writer.key("Name").value("Sheeraz");
JSON_Writer.key("Occupation").value("Software Engineer");
JSON_Writer.key("Age").value(28);
JSON_Writer.key("Married").value(false);
JSON_Writer.key("Skills");
JSON_Writer.array();
JSON_Writer.value("Java");
JSON_Writer.value("Python");
JSON_Writer.value("PHP");
JSON_Writer.value("Matlab");
JSON_Writer.value("R");
JSON_Writer.endArray();
JSON_Writer.endObject();
System.out.println(DemoUser);
}
}
The code above will serialize the data into a JSON object using the JSONWriter
class of the API. See output:
{"Name":"Sheeraz","Occupation":"Software Engineer","Age":28,"Married":false,"Skills":["Java","Python","PHP","Matlab","R"]}
Use Jackson
API to Serialize Java Object to JSON
Jackson
is an open-source library for JSON operations in Java. The library can serialize and deserialize JSON objects in Java.
This library has the method writeValue(...)
, which is used to serialize Java objects to JSON objects. This API can be added as a maven dependency:
<dependency>
<groupId>org.codehaus.jackson</groupId>
<artifactId>jackson-mapper-asl</artifactId>
<version>1.9.13</version>
</dependency>
Another way is to add jars
for Jackson API to your general project build path. Download the jars
from the links below:
Once the API is added to the build path now, we can try an example to serialize Java object to JSON:
package delftstack;
import com.fasterxml.jackson.core.JsonGenerationException;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.File;
import java.io.IOException;
public class Example {
public static void main(String args[]) {
Example JSON_Tester = new Example();
try {
Employee Employee1 = new Employee();
Employee1.setAge(28);
Employee1.setName("Sheeraz");
JSON_Tester.writeJSON(Employee1);
Employee Employee2 = JSON_Tester.readJSON();
System.out.println(Employee2);
} catch (JsonParseException e) {
e.printStackTrace();
} catch (JsonMappingException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
private void writeJSON(Employee employee)
throws JsonGenerationException, JsonMappingException, IOException {
ObjectMapper Object_Mapper = new ObjectMapper();
Object_Mapper.writeValue(new File("employee.json"), employee);
}
private Employee readJSON() throws JsonParseException, JsonMappingException, IOException {
ObjectMapper Object_Mapper = new ObjectMapper();
Employee employee = Object_Mapper.readValue(new File("employee.json"), Employee.class);
return employee;
}
}
class Employee {
private String Employee_Name;
private int Employee_Age;
public Employee() {}
public String getName() {
return Employee_Name;
}
public void setName(String Employee_Name) {
this.Employee_Name = Employee_Name;
}
public int getAge() {
return Employee_Age;
}
public void setAge(int Employee_Age) {
this.Employee_Age = Employee_Age;
}
public String toString() {
return "Employee [Employee Name: "+Employee_Name+",
Employee Age: "+Employee_Age+ " ]";
}
}
The code above will convert the data from a Java class into a JSON. See output:
Employee [Employee Name: Sheeraz, Employee Age: 28 ]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Object
- How to Parse XML to Java Object
- How to Serialize Object to String in Java
- How to Implement Data Access Object in Java
- How to Sort an Array of Objects in Java
- How to Print Objects in Java