How to Print Objects in Java
-
Using
System.out.println()
to Print Objects in Java -
Using
toString()
to Print Objects in Java -
Using
PrintWriter
Class to Print Objects in Java -
Using
String.format()
Library to Print Objects in Java -
Using
Apache Commons Lang
Library to Print Objects in Java - Conclusion
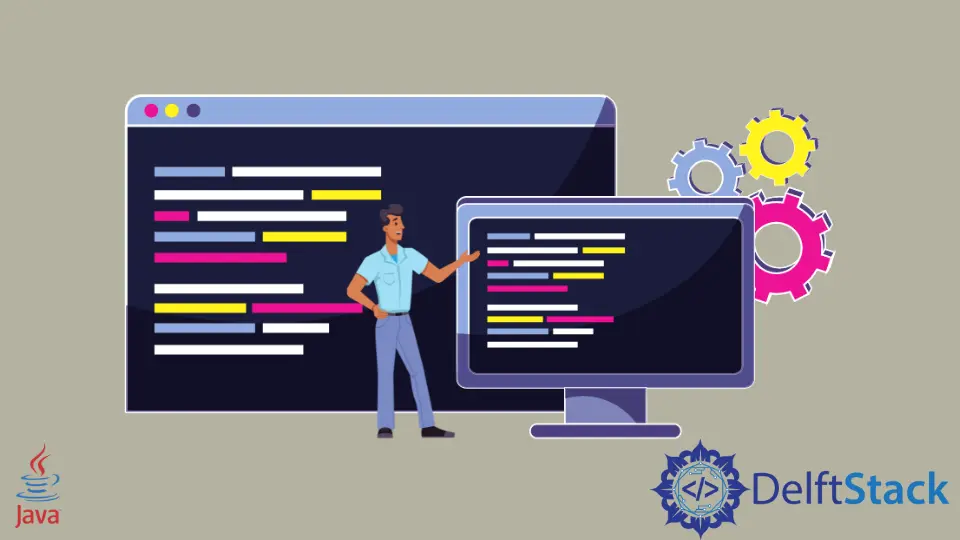
This tutorial introduces how to print an object in Java and lists some example codes to understand the topic.
Printing objects in Java is crucial for debugging and providing meaningful information to developers and users. It aids in understanding the state and behavior of the program during development, helping identify and fix issues.
Additionally, object printing is essential for logging and creating user-friendly outputs, enhancing the overall usability and transparency of Java applications.
Using System.out.println()
to Print Objects in Java
While the System.out.println()
method is a staple for output in Java, understanding how to effectively print different types of objects without relying on the toString()
method is crucial. In this article, we’ll explore a comprehensive example of using System.out.println()
to print a string, an object, and a collection of objects.
The System.out.println()
method is a simple and widely used way to display output in Java.
However, when it comes to printing objects, the default behavior often relies on the object’s toString()
method. In this example, we’ll focus on printing objects without using toString()
, demonstrating the versatility of System.out.println()
.
Let’s dive into a working code example that showcases how to use System.out.println()
to print various types of objects.
import java.util.ArrayList;
import java.util.List;
public class PrintObjectsExample {
public static void main(String[] args) {
// Creating instances for demonstration
String myString = "Hello, Java!";
MyClass myObject = new MyClass("Object1");
List<MyClass> myObjectList = new ArrayList<>();
myObjectList.add(new MyClass("Obj1"));
myObjectList.add(new MyClass("Obj2"));
// Printing different objects using System.out.println()
System.out.println("Printing a String:");
System.out.println(myString);
System.out.println("\nPrinting an Object:");
printObject(myObject);
System.out.println("\nPrinting an Object Collection:");
printObjectCollection(myObjectList);
}
static class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
}
// Method to print an object without using toString()
static void printObject(MyClass obj) {
System.out.println("MyClass{name='" + obj.name + "'}");
}
// Method to print a collection of objects without using toString()
static void printObjectCollection(List<MyClass> objList) {
for (MyClass obj : objList) {
printObject(obj);
}
}
}
In this code example, we start by creating instances for demonstration, including a string (myString
), an object (myObject
), and a list of objects (myObjectList
).
The main focus is on printing these objects using System.out.println()
without relying on the toString()
method.
In the code, printing a string using the System.out.println()
method is a straightforward process. The method simply outputs the content of the myString
variable.
When it comes to printing an object (myObject
), a separate method named printObject
is created. This method takes a MyClass
object and manually constructs the desired string representation for printing.
For printing a collection of objects (myObjectList
), a loop within the printObjectCollection
method is utilized. This method calls the printObject
method for each object in the collection, offering a concise and effective way to print objects without relying on the default toString()
behavior.
When you run the program, the output will be:
Printing a String:
Hello, Java!
Printing an Object:
MyClass{name='Object1'}
Printing an Object Collection:
MyClass{name='Obj1'}
MyClass{name='Obj2'}
This example demonstrates that by carefully crafting the string representation within the System.out.println()
method, we can effectively print objects without relying on the default toString()
behavior. This approach provides developers with more control over how objects are displayed and is particularly useful when customization is required for specific output scenarios in Java applications.
Using toString()
to Print Objects in Java
In Java, the toString()
method plays a significant role in determining the string representation of an object. By overriding this method in your class, you can customize how your objects are printed.
This article explores the concept of printing objects in Java using the toString()
method, covering various types such as strings, arrays, multi-dimensional arrays, objects, and object collections.
The toString()
method is part of the Object
class in Java, and every class inherits it. By default, the toString()
method returns a string containing a textual representation of the object.
However, this representation may not always be meaningful. To address this, Java developers can override the toString()
method in their classes to provide a more informative and customized string representation.
Let’s walk through a comprehensive example demonstrating how to use the toString()
method to print different types of objects in Java.
import java.util.Arrays;
import java.util.List;
public class PrintObjectsExample {
public static void main(String[] args) {
// Creating instances for demonstration
String myString = "Hello, Java!";
int[] myArray = {1, 2, 3, 4, 5};
int[][] myMultiArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
MyClass myObject = new MyClass("Object1");
List<MyClass> myObjectList = Arrays.asList(new MyClass("Obj1"), new MyClass("Obj2"));
// Printing different objects using the overridden toString() method
System.out.println("Printing a String:");
System.out.println(myString);
System.out.println("\nPrinting an Array:");
System.out.println(Arrays.toString(myArray));
System.out.println("\nPrinting a Multi-dimensional Array:");
for (int[] row : myMultiArray) {
System.out.println(Arrays.toString(row));
}
System.out.println("\nPrinting an Object:");
System.out.println(myObject);
System.out.println("\nPrinting an Object Collection:");
for (MyClass obj : myObjectList) {
System.out.println(obj);
}
}
static class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
@Override
public String toString() {
return "MyClass{name='" + name + "'}";
}
}
}
In the code, necessary packages are imported, including java.util.Arrays
for array-related operations. Instances of various types, such as a string, an array, a multi-dimensional array, an object, and a list of objects, are created.
Object printing is done using the overridden toString()
method, automatically called by the System.out.println()
method. The toString()
method is overridden in the MyClass
class to offer a personalized string representation for objects.
Finally, a loop is employed to iterate through the list of objects, and each object is printed using the overridden toString()
method.
When you run the program, the output will be:
Printing a String:
Hello, Java!
Printing an Array:
[1, 2, 3, 4, 5]
Printing a Multi-dimensional Array:
[1, 2, 3]
[4, 5, 6]
[7, 8, 9]
Printing an Object:
MyClass{name='Object1'}
Printing an Object Collection:
MyClass{name='Obj1'}
MyClass{name='Obj2'}
The toString()
method in Java provides a powerful mechanism for customizing the string representation of objects. By overriding this method, developers can control how their objects are printed, enhancing the readability and usability of their code.
This approach not only facilitates debugging and logging but also improves the overall user experience when interacting with Java applications.
Using PrintWriter
Class to Print Objects in Java
In Java, the PrintWriter
class is a versatile tool for outputting data. In this article, we’ll explore how to use the PrintWriter
class to print various types of objects, including strings, arrays, multi-dimensional arrays, objects, and collections.
The PrintWriter
class is part of the java.io
package and is commonly used to write formatted text to a file or another output stream. It provides methods for printing various data types, making it a valuable resource for developers needing to display information in a readable format.
Let’s dive into a comprehensive example that demonstrates how to use the PrintWriter
class to print various objects in Java.
import java.io.PrintWriter;
import java.util.Arrays;
import java.util.List;
public class PrintObjectsExample {
public static void main(String[] args) {
// Creating instances for demonstration
String myString = "Hello, Java!";
int[] myArray = {1, 2, 3, 4, 5};
int[][] myMultiArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
MyClass myObject = new MyClass("Object1");
List<MyClass> myObjectList = Arrays.asList(new MyClass("Obj1"), new MyClass("Obj2"));
// Using PrintWriter to print different objects
try (PrintWriter writer = new PrintWriter(System.out)) {
writer.println("Printing a String:");
writer.println(myString);
writer.println("\nPrinting an Array:");
writer.println(Arrays.toString(myArray));
writer.println("\nPrinting a Multi-dimensional Array:");
for (int[] row : myMultiArray) {
writer.println(Arrays.toString(row));
}
writer.println("\nPrinting an Object:");
writer.println(myObject);
writer.println("\nPrinting an Object Collection:");
for (MyClass obj : myObjectList) {
writer.println(obj);
}
}
}
static class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
@Override
public String toString() {
return "MyClass{name='" + name + "'}";
}
}
}
In the code, necessary packages are imported, such as java.io.PrintWriter
and java.util.Arrays
. Instances of different types are created, including a string, an array, a multi-dimensional array, an object, and a list of objects.
Object printing is accomplished using the PrintWriter
class, employing different print methods for strings, arrays, and objects. Arrays are printed using Arrays.toString()
, while nested loops handle the printing of multi-dimensional arrays.
The toString()
method is overridden in the MyClass
to tailor the string representation of objects. Lastly, a loop is used to iterate through the list of objects, and each object is printed using the PrintWriter
instance.
When you run the program, the output will be:
Printing a String:
Hello, Java!
Printing an Array:
[1, 2, 3, 4, 5]
Printing a Multi-dimensional Array:
[1, 2, 3]
[4, 5, 6]
[7, 8, 9]
Printing an Object:
MyClass{name='Object1'}
Printing an Object Collection:
MyClass{name='Obj1'}
MyClass{name='Obj2'}
This output demonstrates the flexibility of the PrintWriter
class in handling various types of objects, providing a clear and readable representation on the console.
Understanding how to use the PrintWriter
class for printing different objects in Java is a valuable skill for developers. It enhances the debugging and presentation capabilities of Java programs, making the code more accessible and informative.
Using String.format()
Library to Print Objects in Java
While the standard System.out.println()
method is commonly used, the String.format()
method provides a more versatile and controlled way to format and print strings in Java.
In this article, we’ll explore how to use the String.format()
method to print various types of objects, including strings, arrays, multi-dimensional arrays, objects, and object collections.
The String.format()
method in Java is a powerful tool for creating formatted strings by combining fixed text with dynamically generated content. It allows developers to specify placeholders in a format string and substitute them with values, providing a clean and readable way to construct complex strings.
This method is particularly useful when printing objects with specific formatting requirements, offering more control than the standard printing methods.
Let’s dive into a comprehensive example that demonstrates how to use the String.format()
method to print different types of objects in Java.
import java.util.Arrays;
import java.util.List;
public class PrintObjectsExample {
public static void main(String[] args) {
// Creating instances for demonstration
String myString = "Hello, Java!";
int[] myArray = {1, 2, 3, 4, 5};
int[][] myMultiArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
MyClass myObject = new MyClass("Object1");
List<MyClass> myObjectList = Arrays.asList(new MyClass("Obj1"), new MyClass("Obj2"));
// Printing different objects using String.format()
System.out.println("Printing a String:");
System.out.println(String.format("String: %s", myString));
System.out.println("Printing an Array:");
System.out.println(String.format("Array: %s", Arrays.toString(myArray)));
System.out.println("Printing a Multi-dimensional Array:");
for (int[] row : myMultiArray) {
System.out.println(String.format("Array Row: %s", Arrays.toString(row)));
}
System.out.println("Printing an Object:");
System.out.println(String.format("Object: %s", myObject));
System.out.println("Printing an Object Collection:");
for (MyClass obj : myObjectList) {
System.out.println(String.format("Object in Collection: %s", obj));
}
}
static class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
@Override
public String toString() {
return "MyClass{name='" + name + "'}";
}
}
}
Instances of various types, including a string, an array, a multi-dimensional array, an object, and a list of objects, are created for demonstration purposes. The String.format()
method is employed to print each type of object.
This method allows developers to specify placeholders in the format string, and corresponding values are provided to replace these placeholders, creating a clean and readable output. The format string is customized for each type of object, utilizing placeholders like %s
for strings.
In the case of multi-dimensional arrays, %s
within the loop dynamically substitutes array rows, providing a structured representation. Furthermore, the String.format()
method seamlessly integrates with object collections, ensuring a consistent and formatted display of each object in the collection.
This approach enhances the clarity and readability of the printed output, making it a valuable tool for developers when working with diverse data types in Java applications.
When you run the program, the output will be:
Printing a String:
String: Hello, Java!
Printing an Array:
Array: [1, 2, 3, 4, 5]
Printing a Multi-dimensional Array:
Array Row: [1, 2, 3]
Array Row: [4, 5, 6]
Array Row: [7, 8, 9]
Printing an Object:
Object: MyClass{name='Object1'}
Printing an Object Collection:
Object in Collection: MyClass{name='Obj1'}
Object in Collection: MyClass{name='Obj2'}
The String.format()
method in Java offers a flexible and readable way to format and print strings, making it particularly valuable when dealing with complex object representations. By combining fixed text with dynamic values, developers can achieve precise formatting for different types of objects, enhancing the clarity and usability of printed output in Java applications. Understanding and leveraging this method provides developers with a powerful tool for creating well-formatted and informative output in their programs.
Using Apache Commons Lang
Library to Print Objects in Java
While Java’s standard libraries offer effective methods like toString()
and System.out.println()
, there are third-party libraries that can streamline and enhance this process.
In this article, we’ll explore how to print objects in Java using the Apache Commons Lang library’s ToStringBuilder
class. This library provides additional features and flexibility in creating string representations of objects.
The Apache Commons Lang library is a popular utility library that provides reusable, general-purpose classes with additional functionality beyond what is available in the standard Java libraries. One of its components, the ToStringBuilder
class, simplifies the process of creating string representations for objects.
By using this class, developers can easily customize the output format and include specific fields in the printed representation.
Let’s delve into a comprehensive example that demonstrates how to use the ToStringBuilder
class from the Apache Commons Lang library to print various objects in Java.
import java.util.Arrays;
import java.util.List;
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
public class PrintObjectsExample {
public static void main(String[] args) {
// Creating instances for demonstration
String myString = "Hello, Java!";
int[] myArray = {1, 2, 3, 4, 5};
int[][] myMultiArray = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
MyClass myObject = new MyClass("Object1");
List<MyClass> myObjectList = Arrays.asList(new MyClass("Obj1"), new MyClass("Obj2"));
// Printing different objects using ToStringBuilder
System.out.println("Printing a String:");
System.out.println(ToStringBuilder.reflectionToString(myString, ToStringStyle.DEFAULT_STYLE));
System.out.println("\nPrinting an Array:");
System.out.println(ToStringBuilder.reflectionToString(myArray, ToStringStyle.DEFAULT_STYLE));
System.out.println("\nPrinting a Multi-dimensional Array:");
System.out.println(
ToStringBuilder.reflectionToString(myMultiArray, ToStringStyle.DEFAULT_STYLE));
System.out.println("\nPrinting an Object:");
System.out.println(ToStringBuilder.reflectionToString(myObject, ToStringStyle.DEFAULT_STYLE));
System.out.println("\nPrinting an Object Collection:");
System.out.println(
ToStringBuilder.reflectionToString(myObjectList, ToStringStyle.DEFAULT_STYLE));
}
static class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
}
}
In this code snippet, essential packages are imported from the Apache Commons Lang library, specifically ToStringBuilder
and ToStringStyle
. These packages facilitate the use of the ToStringBuilder
class, a valuable tool for creating string representations of objects.
Instances of various types, such as a string, an array, a multi-dimensional array, an object, and a list of objects, are then created for demonstration purposes.
The ToStringBuilder
class’s reflectionToString
method is employed to print each type of object, with the ToStringStyle.DEFAULT_STYLE
argument specifying the default formatting style. This method intelligently builds the string representation by inspecting the fields of the object.
For instance, in the case of the MyClass
object, it includes the name
field in the printed output, providing a comprehensive and customizable view of the object’s state.
When you run the program, the output will be:
Printing a String:
Hello, Java!
Printing an Array:
{1,2,3,4,5}
Printing a Multi-dimensional Array:
{{1,2,3},{4,5,6},{7,8,9}}
Printing an Object:
MyClass@hashcode[name=Object1]
Printing an Object Collection:
[MyClass@hashcode[name=Obj1], MyClass@hashcode[name=Obj2]]
The Apache Commons Lang library’s ToStringBuilder
class provides a convenient way to generate string representations of objects in a flexible and customizable manner. By incorporating this library into your Java projects, you can enhance the debugging and logging capabilities of your applications.
The reflectionToString
method, combined with various ToStringStyle
options, empowers developers to control the format of the printed output.
Conclusion
The Object
class is the superclass of all classes in Java. The toString()
method, which is invoked when printing an object, is implemented in the Object
class. But this implementation doesn’t give any information about the user-defined class properties. To properly view these properties, we need to override our class’s toString()
method. For arrays, we can directly use the toString()
method or the deepToString()
.