How to Sort an Array of Objects in Java
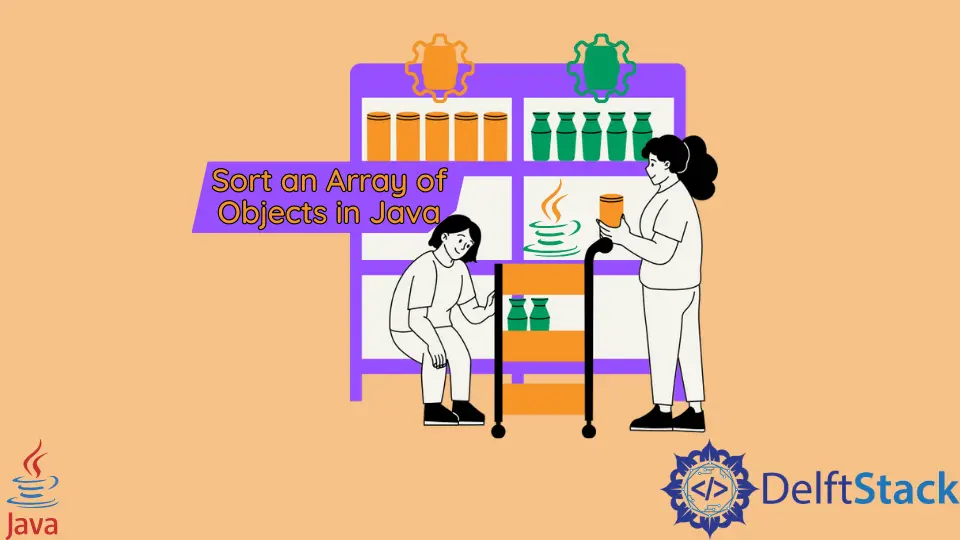
In this tutorial, we will learn how to sort an array of objects using the Comparable
interface and Lambda
functions in Java.
Using Comparable
to Sort Array of Objects in Java
A Comparable
interface in Java is used to order user-defined objects. Here, the Student
class implements Comparable
so that we can use Arrays.sort()
to sort an array of Student
class objects.
The Student
class has three member variables name
, age
, and gender
. We sort elements based on these data members only.
The Comparable interface has one method, compareTo()
. As we can see in the example, the Student
class has implemented this method.
The compareTo()
method is used to sort the current object with the specified object and returns a positive integer in case the current object is greater than the current object. When the current object is less than the specified object, it returns a negative integer; if zero is returned, the present object is equivalent to the specified object.
We created an array of the Student
class objects. We passed name
, age
, and gender
as arguments to initialize them using the class’s default constructor.
The array is first ordered by age
then name
, as we can see in the compareTo()
method of the Student
class. We pass students
array to Arrays.sort()
.
To print the sorted array in our format, we override the toString()
method in the Student
class.
import java.util.Arrays;
class Student implements Comparable<Student> {
private String name;
private int age;
private String gender;
public Student(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
@Override
public String toString() {
return "{"
+ "name='" + name + '\'' + ", gender: " + gender + ", "
+ "age=" + age + '}';
}
public int getAge() {
return age;
}
public String getGender() {
return gender;
}
public String getName() {
return name;
}
@Override
public int compareTo(Student o) {
if (this.age != o.getAge()) {
return this.age - o.getAge();
}
return this.name.compareTo(o.getName());
}
}
public class TestExample {
public static void main(String[] args) {
Student[] students = {new Student("Jack", 25, "male"), new Student("Sandy", 20, "female"),
new Student("Danny", 22, "male"), new Student("Zoey", 10, "female")};
Arrays.sort(students);
System.out.println(Arrays.toString(students));
}
}
Output:
[{name='Zoey', gender: female, age=10}, {name='Sandy', gender: female, age=20}, {name='Danny', gender: male, age=22}, {name='Jack', gender: male, age=25}]
Using Lambda to Sort Array of Objects in Java
Sorting an array of objects becomes easy with the Lambda
functions in Java 8 without implementing the Comparable
interface.
Here, we have a Books
user class with member variables, bookName
and author
. The default constructor initializes these data members.
The getter and setter methods to access these members are also defined in the Book
class. The List
interface supports sort()
method.
Here, bookList
is the instance of list containing the Books
class objects. The lambda expression to sort the list by author is (Books a , Books b) -> a.getAuthor().compareTo(b.getAuthor())
.
The method sort()
returns the sorted list, and we loop over that list and print each element. The sorted list is printed in the output.
import java.util.ArrayList;
import java.util.List;
class Books {
String bookName;
String author;
public Books(String bookName, String author) {
this.bookName = bookName;
this.author = author;
}
@Override
public String toString() {
return "{"
+ "Book ='" + bookName + '\'' + ", author = '" + author + '\'' + "}";
}
public String getAuthor() {
return author;
}
public String getBookName() {
return bookName;
}
}
public class ExampleObjectSort {
public static void main(String[] args) {
List<Books> bookList = new ArrayList<>();
bookList.add(new Books("Barney's Version", "Mordecai Richler"));
bookList.add(new Books("The Unsettlers", "Mark Sundeen"));
bookList.add(new Books("The Debt to Pleasure", "John Lanchester"));
bookList.add(new Books("How to Do Nothing", "Jenny Odell"));
System.out.println("Before Sorting");
bookList.forEach((s) -> System.out.println(s));
System.out.println("");
System.out.println("Sorted Books by author Using Lambda.");
bookList.sort((Books a, Books b) -> a.getAuthor().compareTo(b.getAuthor()));
bookList.forEach((s) -> System.out.println(s));
}
}
Output:
Before Sorting
{Book ='Barney's Version', author = 'Mordecai Richler'}
{Book ='The Unsettlers', author = 'Mark Sundeen'}
{Book ='The Debt to Pleasure', author = 'John Lanchester'}
{Book ='How to Do Nothing', author = 'Jenny Odell'}
Sorted Books by author Using Lambda.
{Book ='How to Do Nothing', author = 'Jenny Odell'}
{Book ='The Debt to Pleasure', author = 'John Lanchester'}
{Book ='The Unsettlers', author = 'Mark Sundeen'}
{Book ='Barney's Version', author = 'Mordecai Richler'}
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java