在 Java 中列印物件
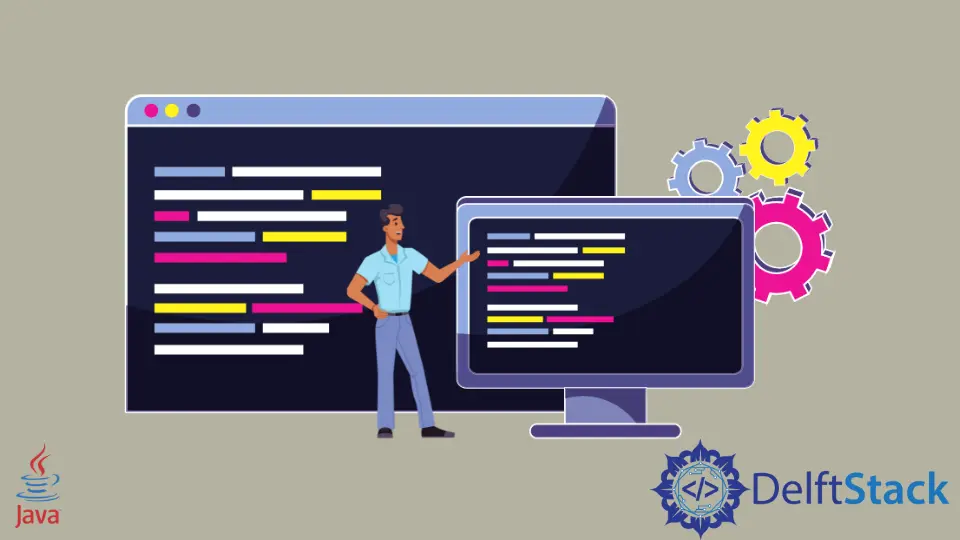
本教程介紹瞭如何在 Java 中列印物件並列出了一些示例程式碼來理解該主題。
物件是類的例項,我們可以使用它來訪問類的屬性和方法。但是如果我們嘗試使用 System.out.println()
方法列印一個物件,我們可能不會得到預期的輸出。我們經常列印物件屬性以進行除錯並確保一切正常。在本教程中,我們將學習如何在 Java 中列印物件屬性。
瞭解物件輸出
關於物件
- 讓我們試著理解當我們列印一個物件時會發生什麼。當我們呼叫 System.out.print() 方法時,就會呼叫 Object 類的
toString()
方法。 - 正如我們所知,Java 中的所有類都擴充套件了
Object
類。因此,toString()
方法可以應用於任何類的任何例項。 - 該方法返回一個由類名和物件的雜湊碼組成的字串。這兩個由@符號連線。
Java 中的列印物件
讓我們建立一個新類並嘗試列印其物件。
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
}
public class Demo {
public static void main(String[] args) {
Student s1 = new Student("Justin", 101, 8.81);
Student s2 = new Student("Jessica", 102, 9.11);
System.out.println(s1);
System.out.println(s2);
}
}
輸出:
Student@7a81197d
Student@5ca881b5
輸出的第一部分顯示類名(在本例中為 Student
),第二部分顯示物件的唯一雜湊碼。每次執行上述程式碼時,我們都會得到不同的雜湊碼。
陣列物件
陣列在 Java 中也是一個物件,當我們嘗試將陣列列印到控制檯時,我們沒有得到它的元素。讓我們執行以下程式碼並檢視其輸出。
public class Demo {
public static void main(String[] args) {
int[] integerArr = {5, 10, 15};
double[] doubleArr = {5.0, 10.0, 15.0};
char[] charArr = {'A', 'B', 'C'};
String[] stringArr = {"Justin", "Jessica"};
int[][] twoDimArray = {{1, 2, 3}, {4, 5, 6}};
System.out.println("Integer Array:" + integerArr);
System.out.println("Double Array:" + doubleArr);
System.out.println("Char Array:" + charArr);
System.out.println("String Array:" + stringArr);
System.out.println("2D Array:" + twoDimArray);
}
}
輸出:
Integer Array:[I@36baf30c
Double Array:[D@7a81197d
Char Array:[C@5ca881b5
String Array:[Ljava.lang.String;@24d46ca6
2D Array:[[I@4517d9a3
- 方括號表示陣列的維度。對於一維陣列,將列印單個左方括號。對於二維陣列,我們有兩個括號。
- 括號後的下一個字元表示陣列中儲存的內容。對於整數陣列,列印一個 I。對於 char 陣列,列印字母 C。
- 字串陣列的 L 表示該陣列包含一個類的物件。在這種情況下,接下來列印類的名稱(在我們的例子中是 java.lang.String)。
- 在@ 符號之後,列印物件的雜湊碼。
如何列印物件
如果我們想以不同的格式列印物件及其屬性,那麼我們需要覆蓋我們類中的 toString()
方法。此方法應返回一個字串。讓我們在我們的 Student 類中覆蓋這個方法並理解這個過程。
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
// overriding the toString() method
@Override
public String toString() {
return this.studentName + " " + this.regNo + " " + this.gpa;
}
}
現在,當我們列印這個類的物件時,我們可以檢視學生的姓名、註冊號和 GPA。
public class Demo {
public static void main(String[] args) {
Student s1 = new Student("Justin", 101, 8.81);
System.out.print(s1);
}
}
輸出:
Justin 101 8.81
列印陣列物件
我們需要使用 Arrays.toString()
方法來檢視陣列中的元素。請注意,如果我們有一個使用者定義的類物件陣列,那麼使用者定義的類也應該有一個重寫的 toString()
方法。這將確保正確列印類屬性。
import java.util.Arrays;
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
// overriding the toString() method
@Override
public String toString() {
return this.studentName + " " + this.regNo + " " + this.gpa;
}
}
public class Demo {
public static void main(String[] args) {
Student s1 = new Student("Justin", 101, 8.81);
Student s2 = new Student("Jessica", 102, 9.11);
Student s3 = new Student("Simon", 103, 7.02);
// Creating Arrays
Student[] studentArr = {s1, s2, s3};
int[] intArr = {5, 10, 15};
double[] doubleArr = {5.0, 10.0, 15.0};
String[] stringArr = {"Justin", "Jessica"};
System.out.println("Student Array: " + Arrays.toString(studentArr));
System.out.println("Intger Array: " + Arrays.toString(intArr));
System.out.println("Double Array: " + Arrays.toString(doubleArr));
System.out.println("String Array: " + Arrays.toString(stringArr));
}
}
輸出:
Student Array: [Justin 101 8.81, Jessica 102 9.11, Simon 103 7.02]
Intger Array: [5, 10, 15]
Double Array: [5.0, 10.0, 15.0]
String Array: [Justin, Jessica]
列印多維陣列物件
對於多維陣列,使用 deepToString()
方法而不是 toString()
方法並將所需的輸出傳送到控制檯。
import java.util.Arrays;
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
// overriding the toString() method
@Override
public String toString() {
return this.studentName + " " + this.regNo + " " + this.gpa;
}
}
public class Demo {
public static void main(String[] args) {
Student s1 = new Student("Justin", 101, 8.81);
Student s2 = new Student("Jessica", 102, 9.11);
Student s3 = new Student("Simon", 103, 7.02);
Student s4 = new Student("Harry", 104, 8.0);
Student[][] twoDimStudentArr = {{s1, s2}, {s3, s4}};
System.out.println("Using toString(): " + Arrays.toString(twoDimStudentArr));
System.out.println("Using deepToString(): " + Arrays.deepToString(twoDimStudentArr));
}
}
輸出:
Using toString(): [[LStudent;@7a81197d, [LStudent;@5ca881b5]
Using deepToString(): [[Justin 101 8.81, Jessica 102 9.11], [Simon 103 7.02, Harry 104 8.0]]
在 Java 中列印集合物件
像 Lists
、Sets
和 Maps
這樣的集合不需要像 Arrays.toString()
這樣的任何新增方法。如果我們正確地覆蓋了我們類的 toString()
方法,那麼簡單地列印集合就會給我們所需的輸出。
import java.util.ArrayList;
import java.util.HashSet;
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
// overriding the toString() method
@Override
public String toString() {
return this.studentName + " " + this.regNo + " " + this.gpa;
}
}
public class Demo {
public static void main(String[] args) {
Student s1 = new Student("Justin", 101, 8.81);
Student s2 = new Student("Jessica", 102, 9.11);
Student s3 = new Student("Simon", 103, 7.02);
// Creating an ArrayList
ArrayList<Student> studentList = new ArrayList<>();
studentList.add(s1);
studentList.add(s2);
studentList.add(s3);
// Creating a Set
HashSet<Student> studentSet = new HashSet<>();
studentSet.add(s1);
studentSet.add(s2);
studentSet.add(s3);
System.out.println("Student List: " + studentList);
System.out.println("Student Set: " + studentSet);
}
}
輸出:
Student List: [Justin 101 8.81, Jessica 102 9.11, Simon 103 7.02]
Student Set: [Simon 103 7.02, Justin 101 8.81, Jessica 102 9.11]
使用 Apache 公共庫
如果你正在使用 Apache 公共庫,那麼使用 Apache 公共庫的 ToStringBuilder
類以不同的方式格式化我們的物件。我們可以使用這個類的 reflectionToString()
方法。
我們可以使用此方法簡單地列印物件類和雜湊碼以及為屬性設定的值。
import org.apache.commons.lang3.builder.ToStringBuilder;
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
}
public class Demo {
public static void main(String[] args) {
Student s = new Student("Justin", 101, 8.81);
System.out.print(s);
}
}
輸出:
Student@25f38edc[gpa=8.81,regNo=101,studentName=Justin]
如果我們想省略雜湊碼,那麼我們可以使用 SHORT_PREFIX_STYLE 常量。請參考下面的示例。
import org.apache.commons.lang3.builder.ToStringBuilder;
import org.apache.commons.lang3.builder.ToStringStyle;
class Student {
private String studentName;
private int regNo;
private Double gpa;
Student(String s, int i, Double d) {
this.studentName = s;
this.regNo = i;
this.gpa = d;
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, ToStringStyle.SHORT_PREFIX_STYLE);
}
}
public class Demo {
public static void main(String[] args) {
Student s = new Student("Justin", 101, 8.81);
System.out.print(s);
}
}
輸出:
Student[gpa=8.81,regNo=101,studentName=Justin]
如果我們的類包含巢狀物件,我們可以使用 RecursiveToStringStyle()
方法來列印物件。
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this, new RecursiveToStringStyle());
}
總結
Object
類是 Java 中所有類的超類。在列印物件時呼叫的 toString()
方法在 Object
類中實現。但是這個實現沒有提供關於使用者定義的類屬性的任何資訊。為了正確檢視這些屬性,我們需要覆蓋我們類的 toString()
方法。對於陣列,我們可以直接使用 toString()
方法或 deepToString()
。