How to Handle JSON Arrays in Java
- Handling JSON Arrays in Java
- JSON Objects and JSON Arrays in Java
- Use Java’s Built-in Libraries to Read a JSON Array in Java
- Conclusion
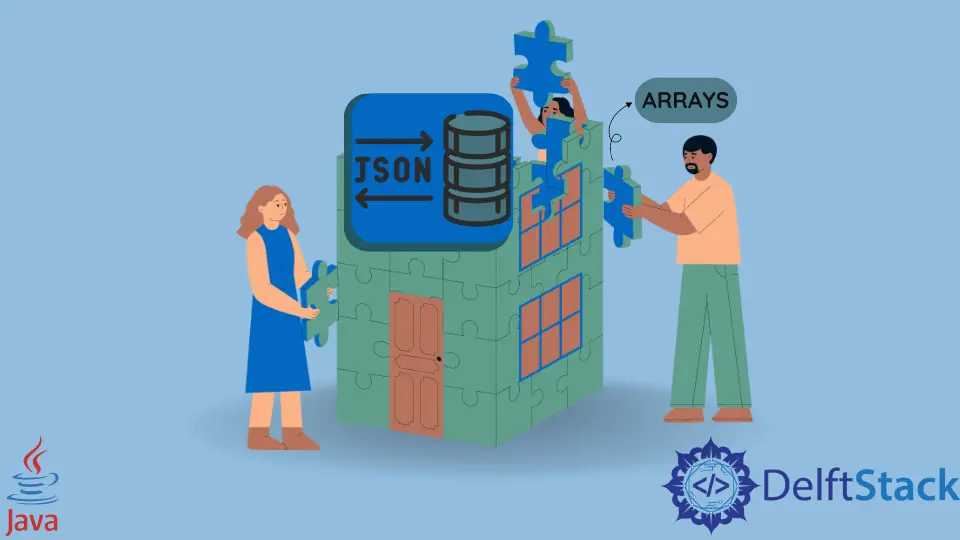
JSON is a popular and widely used data-interchange format among developers and organizations. The term JSON stands for JavaScript Object Notation.
It is a lightweight, readable, and text-based framework specially designed to facilitate data exchange in the human-readable format. JSON is an open standard format that means it can be used anywhere without special requirements.
Moreover, JSON is supported by most programming languages. You might have come across JSON if you ever worked as a project developer that requires data interchange.
For example, we often use JSON Objects to transfer data from a server to a mobile application and vice versa. This article will understand JSON basics, such as the structure of JSON, data types, JSON Objects, JSON Arrays, etc.
We will also understand how to work with JSON in Java. In the end, this article provides an example code to handle JSON in Java.
Handling JSON Arrays in Java
JSON is a very flexible and lightweight information-sharing framework where we use objects and datatypes to create and share information.
In JSON, data is represented as key-value pairs where the key is specifically a string, while the value can be any data type supported by JSON.
JSON supports the following data types.
- String
- Number
- Boolean
- Null
In addition to these basic JSON data types, we can also use JSON Arrays and JSON Objects as the value in a key-value pair.
Further, in the article, we will study more about arrays and objects. But before that, let’s look at the features of JSON.
Some of the outstanding features of JSON are as follows.
- JSON is very simple. You don’t have to put any extra effort to understand and use JSON.
- JSON is easily understandable and self-describing. By looking at a JSON object, you can easily comprehend what it is intended to convey.
- JSON is language independent. This feature has proved to be a major factor in the wide acceptance of JSON. We can work with JSON in almost every language with the help of libraries and simple codes.
- JSON is faster than XML. It is yet another reason for using it widely.
JSON Objects and JSON Arrays in Java
Before we learn to use JSON objects and arrays in Java, we shall understand the JSON objects and JSON arrays.
JSON objects are very similar to the dictionary data type, where the data is stored as key-value pairs. The key in this key-value pair should be a string, while the value can be an object, an array, or any other supported datatype.
We must separate a key and a value by a colon :
and each key-value pair by a comma ,
. A JSON object is represented by using curly brackets {}
.
We can make arbitrarily complex JSON objects by nesting objects and arrays into our JSON objects. It is called a hierarchical JSON object.
Here’s an example of a JSON object representing a student’s details.
{"name": "Roger", "Roll": 22, "Class": "XI"}
Note that we have quoted the string values with double-converted commas, while numbers do not need to be quoted.
We have just discussed JSON objects that are similar to a dictionary. On the other hand, JSON arrays are similar to lists.
We enclose objects in square brackets []
in a list. A JSON array can contain any supported data type, including another array or a JSON object.
Here is an example of a JSON array.
[1, "abc", {"name":"xyz"}]
Note that the JSON array above contains a number, a string, and an object. It means we can store different data types in an array.
Use Java’s Built-in Libraries to Read a JSON Array in Java
Java is a powerful language with a large number of libraries. Luckily, we have built-in libraries to read JSON objects and arrays in Java.
Such libraries facilitate our work and make it simple to parse and read JSON objects and arrays. Further in this article, we will learn to use such libraries in code to read the JSON object and JSON array in Java.
Before proceeding to the code, we create a JSON object and store it in a file. Note that a JSON file has the extension .json
.
So if you want to test and execute the code, copy and paste the following JSON code and save the file with the .json
extension at any desired location. The JSON code is given below.
[{
"name":"MMN",
"roll": 24,
"marks": [91, 95, 97, 97, 94],
"parents": {
"name": "IMT",
"mobile":"1234567890"
},
"hobbies": ["sports", "reading", "music"],
"others": ["A", 1]
}]
You should note that this JSON file contains a JSON Array with a single JSON Object. This inner JSON Object then contains several key-value pairs with different data types.
For example, name
is a string, roll
is an integer (note that JSON stores integers as long integers), marks
is a JSON Array, parents
is another JSON Object, and so on. Therefore, this is an example of arbitrarily complex JSON data.
Let us understand how we can read such a JSON Object using Java.
Before writing the code, you must make sure that you have downloaded the JSON jar file and added the classpath
variable pointing to that JSON file. If you do not do this, JSON will not be compiled.
We can open a JSON file the same way as we open any other file in Java, essentially by using the File()
constructor.
We must surround the file opening statement with a try-catch
block to handle the necessary exception that the constructor throws.
Once the file is open, its object is passed to the FileReader()
constructor.
We use a JSON parser that returns a Java Object to parse the JSON content. We should use an explicit cast to cast a JSON Array.
Our JSON Array contains a single object, but in some cases, we might have multiple JSON Objects in a JSON Array, so we should traverse the parsed array using a loop. We extract each JSON Object from the parsed JSON Array and further process it.
Note that we should know the JSON Object structure that we are iterating because we need to extract the values in a JSON Object using the key.
Further, we extract the data from the JSON Object by passing it to a function that uses named keys. Since JSON Object contains different data types, each can be handled accordingly.
For example, we displayed the integer, string, and array but iterated the nested JSON Object parents
. The following code implements the logic explained.
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.*;
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.JSONParser;
import org.json.simple.parser.ParseException;
public class JSONReader {
public static void main(String[] args) {
JSONParser parser = new JSONParser();
FileReader fileReader;
try {
File file = new File("/home/stark/eclipse-workspace-java/Aditya/src/data.json");
fileReader = new FileReader(file);
// Parser returns an object, we need an explicit cast to covert it into a JSONArray
JSONArray array = (JSONArray) parser.parse(fileReader);
// Traverse the list
for (int i = 0; i < array.size(); i++) {
JSONObject obj = (JSONObject) array.get(i);
parseObject(obj);
}
}
catch (FileNotFoundException e) {
System.out.println(e.getStackTrace() + " :File Not Found");
} catch (ParseException e) {
System.out.println(e.getStackTrace() + " :Could not parse");
} catch (IOException e) {
System.out.println(e.getStackTrace() + " :IOException");
}
}
private static void parseObject(JSONObject obj) {
String name = (String) obj.get("name");
long roll = (long) obj.get("roll");
JSONArray marks = (JSONArray) obj.get("marks");
JSONArray hobbies = (JSONArray) obj.get("hobbies");
JSONObject parents = (JSONObject) obj.get("parents");
JSONArray others = (JSONArray) obj.get("others");
System.out.println("Name: " + name);
System.out.println("Roll: " + roll);
System.out.println("Marks: " + marks);
System.out.println("Hobbies: " + hobbies);
System.out.println(
"Parent's Name: " + parents.get("name") + ", Mobile: " + parents.get("mobile"));
System.out.println("Others: " + others);
}
}
You should specify the correct path of the file in the File()
constructor. The output of the code above is below.
Name: MMN
Roll: 24
Marks: [91,95,97,97,94]
Hobbies: ["sports","reading","music"]
Parent's Name: IMT, Mobile: 1234567890
Others: ["A",1]
Conclusion
Java is a popular programming language, and often we come across situations where we need data interchange. Although other data interchange formats exist, JSON is one of the most popular, easy, and understandable formats.
We have learned JSON format, its datatypes, and parsed JSON files in Java. JSON can have an arbitrarily complex structure that enables us to interchange any JSON data.
On the other hand, Java provides a simple, unique yet powerful method to handle the JSON.