How to Convert JSON Data to String in Java
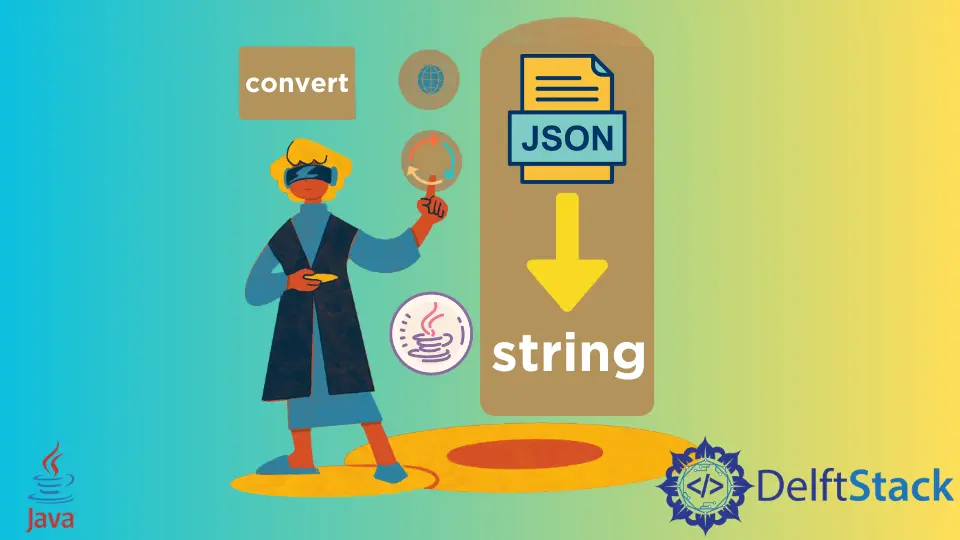
JSON, fully known as JavaScript Object Notation, is a text format for transporting and storing data. It is easy to understand and self-describing.
JSON is a lightweight format for data interchange. It uses plain text with a combination of JavaScript object notation.
Besides, JSON is language-independent, so you can easily create, modify, or store data using any programming language.
Sometimes, we need to convert the JSON data into the string for performing various operations like extracting specific data. This article will show how we can convert JSON data or files into strings.
Also, we will discuss the topic with necessary examples and explanations to make the topic easier.
Create JSON Data From String in Java
First, we will see how we can convert JSON data into a string.
We will convert a JSON object into a string in the example below. The code for our example will be the following:
import org.json.CDL;
import org.json.JSONArray;
import org.json.JSONTokener;
public class JavaArticles {
public static void main(String[] args) {
String JsonData = "CANADA, UK, USA"; // Taking the JSON data as string
JSONArray JsonArray =
CDL.rowToJSONArray(new JSONTokener(JsonData)); // Converting to the JSON array
System.out.println(JsonArray); // Printing the JSON array
System.out.println(CDL.rowToString(JsonArray));
JsonArray = new JSONArray(); // Creating a JSON array object.
JsonArray.put("ID"); // Put a field to JSON array name `ID`
JsonArray.put("Name"); // Put a field to JSON array name `Name`
JsonArray.put("Age"); // Put a field to JSON array name `Age`
JsonData = "1, Alex, 25 \n"
+ "2, Robert, 30 \n"
+ "3, Micle, 27"; // Taking the JSON data as string
System.out.println(CDL.toJSONArray(JsonArray, JsonData)); // Printing the JSON data
}
}
The example above illustrates how to generate a JSON file from the string. Also, we already provided the purpose of each line in the code.
After you execute the program above, you will get an output like the one below.
["CANADA","UK","USA"]
CANADA,UK,USA
[{"Age":"25","ID":"1","Name":"Alex"},{"Age":"30","ID":"2","Name":"Robert"},{"Age":"27","ID":"3","Name":"Micle"}]
Read JSON File as String in Java
Now we will see how we can read the JSON file as a string.
In our next example, we will extract the content of a JSON file and then convert it to a string. For the example, suppose we have a JSON file like the following:
{
"name":"Thomas",
"age":22,
"hobbies":["Gardening","Swimming"],
"languages":{"English":"Advanced"}
}
The code for our example code is shown below:
import java.nio.file.Files;
import java.nio.file.Paths;
public class ReadJsonFileAsString {
public static void main(String[] args) throws Exception {
String Myfile = "test/resources/myFile.json";
String JsonData = readFileAsString(Myfile);
System.out.println(JsonData);
}
public static String readFileAsString(String Myfile) throws Exception {
return new String(Files.readAllBytes(Paths.get(Myfile)));
}
}
In the example above, we first take the file’s location in a string variable. After that, we read the file using the readFileAsString()
method defined at the end of the code.
This method will return a string. We print that string through the line System.out.println(JsonData);
.
Now for the function, we read the file using the readAllBytes()
method and returned the data. After executing the program, you will get an output like the one below.
{
"name":"Thomas",
"age":22,
"hobbies":["Gardening","Swimming"],
"languages":{"English":"Advanced"}
}
Please include the necessary .jar
file on your project when working with JSON. Otherwise, you may get an error.
Note that the example codes shared in this article are written in Java. You must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - Java JSON
- How to Pretty-Print JSON Data in Java
- How to Serialize Object to JSON in Java
- How to Deserialize JSON in Java
- How to Handle JSON Arrays in Java
- How to Convert JSON to Java object