How to Pretty-Print JSON Data in Java
-
Use
Gson
to Pretty-Print JSON Data in Java -
Use
JSON
to Pretty-Print JSON Data in Java -
Use
Jackson
to Pretty-Print JSON Data in Java
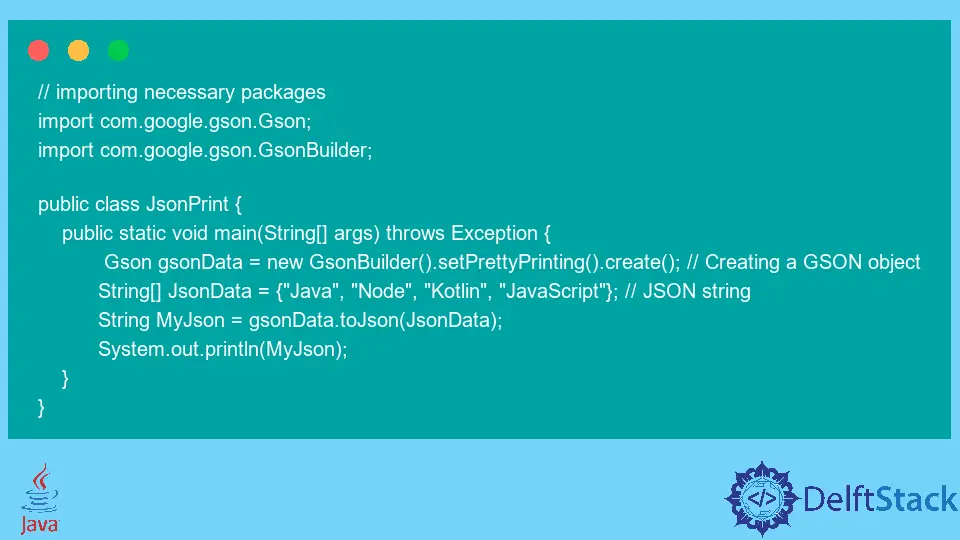
JSON is a mainly used medium to share information. Numerous tools are available to create, modify and parse JSON files; however, these files are mostly not human-readable, so it’s not easy for a human to understand the JSON data.
There is a way through which it is called the Pretty-Print
. This article will discuss how we can Pretty-Print
a JSON file in Java.
We will discuss the topic using necessary examples and explanations to make the matter easier. We are going to discuss the three most used methods in this article.
Use Gson
to Pretty-Print JSON Data in Java
In our example below, we will see how we can Pretty-Print
JSON data using Gson
. The code will be as follows:
// importing necessary packages
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class JsonPrint {
public static void main(String[] args) throws Exception {
Gson gsonData = new GsonBuilder().setPrettyPrinting().create(); // Creating a GSON object
String[] JsonData = {"Java", "Node", "Kotlin", "JavaScript"}; // JSON string
String MyJson = gsonData.toJson(JsonData);
System.out.println(MyJson);
}
}
We have commanded the purpose of each line. Now, after executing the example shared above, you will get an output like the one below:
[
"Java",
"Node",
"Kotlin",
"JavaScript"
]
Use JSON
to Pretty-Print JSON Data in Java
In our example below, we will see how we can Pretty-Print
JSON data using JSON
. The code will be as follows:
// importing necessary packages
import org.json.JSONObject;
public class JsonPrint {
public static void main(String[] args) throws Exception {
// Creating a JSON object
String JsonData = "{\"one\":\"AAA\", \"two\":\"BBB\", \"three\":\"CCC\", \"four\":\"DDD\",}";
// JSON string
System.out.println(new JSONObject(JsonData).toString(4));
}
}
We have already commanded the purpose of each line. Now, after executing the example shared above, you will get the following output:
{
"four": "DDD",
"one": "AAA",
"two": "BBB",
"three": "CCC"
}
Use Jackson
to Pretty-Print JSON Data in Java
In our example below, we will see how we can Pretty-Print
JSON data using Jackson
. The code will be as follows:
// importing necessary packages
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
public class JsonPrint {
public static void main(String[] args) throws IOException {
// Creating a ObjectMapper object
ObjectMapper MyMapper = new ObjectMapper().enable(SerializationFeature.INDENT_OUTPUT);
// JSON string
String inputJson = "{\"one\":\"AAA\", \"two\":\"BBB\", \"three\":\"CCC\", \"four\":\"DDD\"}";
System.out.println(MyMapper.writeValueAsString(MyMapper.readTree(inputJson)));
}
}
We have already commanded the purpose of each line. Now, after executing the example shared above, you will get the output below:
{
"one" : "AAA",
"two" : "BBB",
"three" : "CCC",
"four" : "DDD"
}
Please note that the example codes shared in this article are in Java. You must install Java on your environment if your system doesn’t have it.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn