How to Convert Char to Int in Java
- Method 1: Using Type Casting
- Method 2: Using Character.getNumericValue()
- Method 3: Using Integer.parseInt() with String Conversion
- Conclusion
- FAQ
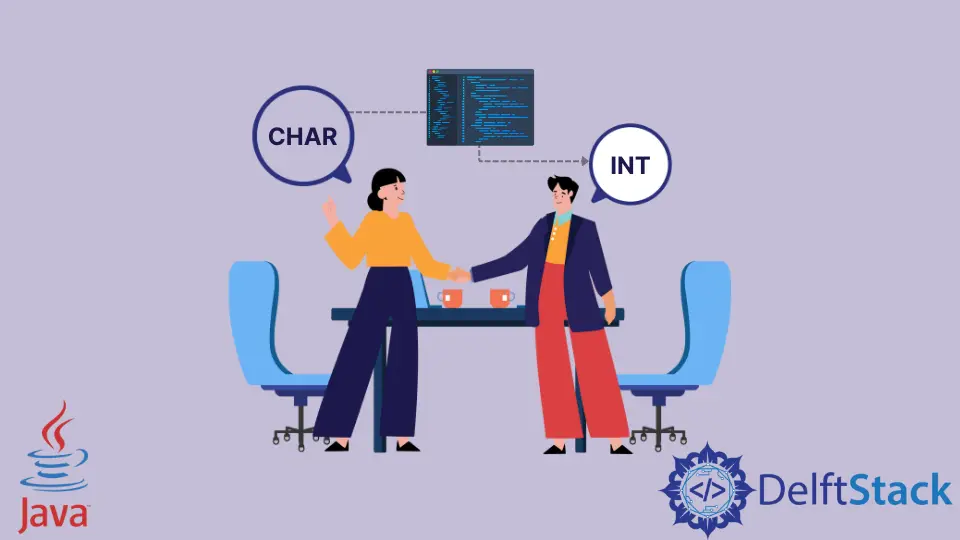
Converting a character to an integer in Java can seem daunting at first, especially for beginners. However, it’s a straightforward process that can be accomplished using several methods. Whether you are working on a small project or a larger application, understanding how to perform this conversion is essential for effective programming.
In this article, we will explore various methods to convert a char to an int in Java, providing clear examples and explanations to help you grasp the concept. By the end of this article, you will have a solid understanding of how to perform this conversion efficiently and effectively.
Method 1: Using Type Casting
One of the simplest ways to convert a char to an int in Java is through type casting. In Java, characters are represented using the Unicode standard, which assigns a unique integer value to each character. By directly casting a char to an int, you can obtain its corresponding integer value.
Here’s a quick example to illustrate this method:
public class CharToInt {
public static void main(String[] args) {
char character = 'A';
int integerValue = (int) character;
System.out.println("The integer value of '" + character + "' is: " + integerValue);
}
}
Output:
The integer value of 'A' is: 65
In this code snippet, we define a character variable character
and assign it the value ‘A’. By casting this character to an integer using (int)
, we retrieve its Unicode value, which is 65. This method is efficient and straightforward, making it a popular choice among developers.
Method 2: Using Character.getNumericValue()
Another method to convert a char to an int in Java is by utilizing the Character.getNumericValue()
method. This method is particularly useful when you are dealing with numeric characters, as it converts the character representation of a digit into its corresponding integer value.
Here’s how you can use this method:
public class CharToInt {
public static void main(String[] args) {
char character = '5';
int integerValue = Character.getNumericValue(character);
System.out.println("The integer value of '" + character + "' is: " + integerValue);
}
}
Output:
The integer value of '5' is: 5
In this example, we define a character variable character
with the value ‘5’. By calling Character.getNumericValue(character)
, we convert the character ‘5’ to its integer representation, which is 5. This method is particularly useful when dealing with characters that represent digits, as it handles the conversion seamlessly.
Method 3: Using Integer.parseInt() with String Conversion
If you are working with characters that represent digits, another approach is to convert the character to a String and then use Integer.parseInt()
to obtain the integer value. This method is a bit more indirect but can be useful in certain scenarios.
Here’s an example:
public class CharToInt {
public static void main(String[] args) {
char character = '7';
int integerValue = Integer.parseInt(String.valueOf(character));
System.out.println("The integer value of '" + character + "' is: " + integerValue);
}
}
Output:
The integer value of '7' is: 7
In this code, we first convert the character ‘7’ to a String using String.valueOf(character)
. Then, we pass that String to Integer.parseInt()
to obtain its integer value. While this method may seem a bit roundabout, it can be particularly useful when you are already working with Strings or need to perform additional string manipulations.
Conclusion
Converting a char to an int in Java is a fundamental skill that every Java developer should master. Whether you choose to use type casting, the Character.getNumericValue()
method, or Integer.parseInt()
, each method has its own advantages depending on the context. By understanding these methods, you can enhance your programming skills and handle character-to-integer conversions with ease. Remember, practice makes perfect, so don’t hesitate to try these methods in your own projects.
FAQ
- How do I convert a character to an integer in Java?
You can convert a character to an integer in Java using type casting,Character.getNumericValue()
, orInteger.parseInt()
.
-
What is the Unicode value of the character ‘A’?
The Unicode value of the character ‘A’ is 65. -
Can I convert non-numeric characters to integers?
Yes, you can convert any character to its Unicode integer value using type casting, but non-numeric characters won’t have meaningful integer representations in the context of numbers. -
Is there a method to convert a character string to an integer?
Yes, you can convert a character string to an integer usingInteger.parseInt()
after converting the character to a string. -
Are there any performance differences between the methods?
Type casting is generally the fastest method, while methods involving string conversion may be slower due to additional processing.
Related Article - Java Int
- How to Convert Int to Char in Java
- How to Convert Int to Double in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java
- How to Convert Int to Byte in Java