How to Check if a String Is an Integer in Java
-
Check if the String Is an Integer by
Character.digit()
in Java -
Check if the String Is an Integer by
string.matches(pattern)
in Java -
Check if the String Is an Integer by
Scanner.nextInt(radix)
in Java
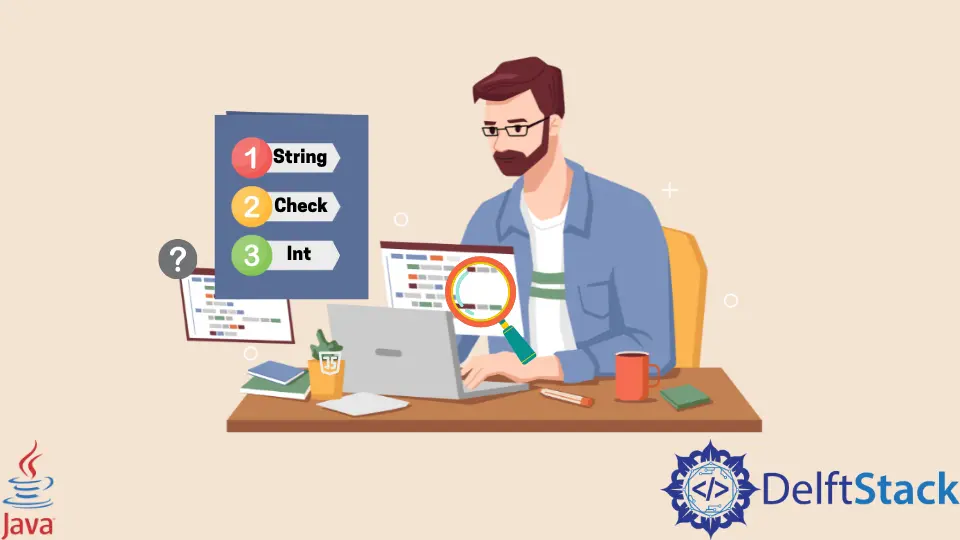
String and Integers in Java are often used for storing data, but sometimes we might want to check if one data type contains elements compatible with another data type or not.
As we know that a String can contain alphabets, symbols, and numbers, it is useful to determine the type of data our string is holding. We are going to see examples of checking the string with different methods.
Check if the String Is an Integer by Character.digit()
in Java
We can see that the following example has three strings that we can pass into the function isStringInteger(stringToCheck, radix)
. radix
tells the range of the number that we want to get, here we are using 10, which allows the range of 0 to 9.
Character.digit()
checks every character in the string and returns a number more than 0 if it is a digit. Additional conditional statements can also be added to make the result accurate.
public class Main {
public static void main(String[] args) {
String str1 = "ABC123";
String str2 = "3030";
String str3 = "-9";
boolean integerOrNot1 = isStringInteger(str1, 10);
System.out.println("Is " + str1 + " an Integer? -> " + integerOrNot1);
boolean integerOrNot2 = isStringInteger(str2, 10);
System.out.println("Is " + str2 + " an Integer? -> " + integerOrNot2);
boolean integerOrNot3 = isStringInteger(str3, 10);
System.out.println("Is " + str3 + " an Integer? -> " + integerOrNot3);
}
public static boolean isStringInteger(String stringToCheck, int radix) {
if (stringToCheck.isEmpty())
return false; // Check if the string is empty
for (int i = 0; i < stringToCheck.length(); i++) {
if (i == 0 && stringToCheck.charAt(i) == '-') { // Check for negative Integers
if (stringToCheck.length() == 1)
return false;
else
continue;
}
if (Character.digit(stringToCheck.charAt(i), radix) < 0)
return false;
}
return true;
}
}
Output:
Is ABC123 an Integer? -> false
Is 3030 an Integer? -> true
Is -9 an Integer? -> true
Check if the String Is an Integer by string.matches(pattern)
in Java
In the next method of identifying if the string contains Integer elements, we can use the Regular Expression, which can help match a specific pattern, i.e., numerical value. -?\\d+
is the expression that we can match against the string and get the result in a boolean type.
public class Main {
public static void main(String[] args) {
String str1 = "ABC123";
String str2 = "123";
String str3 = "000000009";
boolean integerOrNot1 = str1.matches("-?\\d+");
System.out.println("Is " + str1 + " an Integer? -> " + integerOrNot1);
boolean integerOrNot2 = str2.matches("-?\\d+");
System.out.println("Is " + str2 + " an Integer? -> " + integerOrNot2);
boolean integerOrNot3 = str3.matches("-?\\d+");
;
System.out.println("Is " + str3 + " an Integer? -> " + integerOrNot3);
}
}
Output:
Is ABC123 an Integer? -> false
Is 123 an Integer? -> true
Is 000000009 an Integer? -> true
Check if the String Is an Integer by Scanner.nextInt(radix)
in Java
We can also use the famous Scanner()
class of Java. Its nextInt()
method can check if the given string is of Int
type or not.
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
String str1 = "ABC123";
String str2 = "3030";
String str3 = "000000009";
System.out.println("Is " + str1 + " an Integer? -> " + isStringInteger(str1, 10));
System.out.println("Is " + str2 + " an Integer? -> " + isStringInteger(str2, 10));
System.out.println("Is " + str3 + " an Integer? -> " + isStringInteger(str3, 10));
}
public static boolean isStringInteger(String stringToCheck, int radix) {
Scanner sc = new Scanner(stringToCheck.trim());
if (!sc.hasNextInt(radix))
return false;
sc.nextInt(radix);
return !sc.hasNext();
}
}
Output:
Is ABC123 an Integer? -> false
Is 3030 an Integer? -> true
Is 000000009 an Integer? -> true
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java