How to Iterate Over Characters of String in Java
-
Use
String.chars()
in Java 8 to Iterate Over All Characters in a String -
Use
String.codePoints()
Java 8 to Loop Over All Characters in a String in Java -
Use
String.toCharArray()
to Loop Over All Characters in a String in Java -
Use
StringCharacterIterator
to Iterate Over All Characters in a String in Java -
Use
String.split()
to Loop Over All Characters in a String in Java
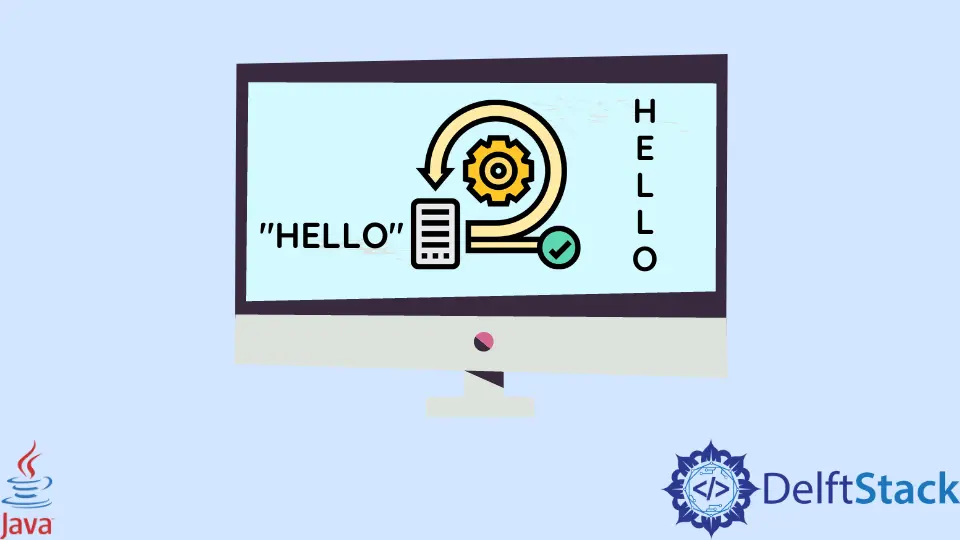
This article will introduce various methods to iterate over every character in a string in Java.
Use String.chars()
in Java 8 to Iterate Over All Characters in a String
Java 8 provides us with a new method String.chars()
which returns an IntStream
. The returned IntStream
contains an integer representation of the characters in the string. Here we get stream1
from myString.chars()
.
We map the returned IntStream
into an object. The stream1.mapToObj()
converts the integer values into their respective character equivalent. However, to display and read the characters, we need to convert them into a user-friendly character form.
import java.util.stream.IntStream;
public class ForEachChar {
public static void main(String[] args) {
String myString = "Hello There!";
String myString2 = "My Friends";
String myString3 = "Happy Coding";
IntStream stream1 = myString.chars();
IntStream stream2 = myString2.chars();
IntStream stream3 = myString3.chars();
stream1.mapToObj(Character::toChars).forEach(System.out::print);
System.out.println("");
stream2.mapToObj(i -> new Character((char) i)).forEach(System.out::print);
System.out.println("");
stream3.forEach(i -> System.out.print(Character.toChars(i)));
}
}
Output:
Hello There!
My Friends
Happy Coding
Use String.codePoints()
Java 8 to Loop Over All Characters in a String in Java
Java 8 String.codePoints()
returns an IntStream
of Unicode code points from this sequence. It returns the ASCII
values of the character passed.
We can map the returned IntStream
to an object using stream.mapToObj
so that it will be automatically converted into a Stream<Character>
. We use the method reference and print each character in the specified string.
import java.util.stream.IntStream;
public class ForEachChar {
public static void main(String[] args) {
String myString = "Happy Coding";
IntStream stream = myString.codePoints();
stream.mapToObj(Character::toChars).forEach(System.out::print);
}
}
Output:
Happy Coding
Use String.toCharArray()
to Loop Over All Characters in a String in Java
The String.toCharArray()
method converts the given string into a sequence of characters. It returns a Character
array whose length is similar to the length of the string.
To iterate over every character in a string, we can use toCharArray()
and display each character.
public class ForEachChar {
public static void main(String[] args) {
String myString = "Hello Friends";
char[] chars = myString.toCharArray();
int len = chars.length;
System.out.println("length of the char array: " + len);
for (char ch : chars) {
System.out.println(ch);
}
}
}
Output:
length of the char array: 13
H
e
l
l
o
F
r
i
e
n
d
s
Use StringCharacterIterator
to Iterate Over All Characters in a String in Java
The StringCharacterIterator
class implements a bidirectional iteration of the string. It takes a string as the parameter, which constructs an iterator with an initial index of 0.
In the while
loop, we call current()
on the iterator it
, which returns the character at the current position or returns DONE
if the current position is the end of the text.
The next()
method on it
returns the character at the new position or DONE
if the new position is the end.
import java.text.CharacterIterator;
import java.text.StringCharacterIterator;
public class ForEachChar {
public static void main(String[] args) {
String myString = "Hello Friends";
CharacterIterator it = new StringCharacterIterator(myString);
while (it.current() != CharacterIterator.DONE) {
System.out.print(it.current());
it.next();
}
}
}
Output:
Hello Friends
Use String.split()
to Loop Over All Characters in a String in Java
The String.split()
method splits the string against the given regular expression and returns a new array.
In the code below, we use myString.split("")
to split the string between each character. We can iterate every character in the str_arr
and display it.
public class ForEachChar {
public static void main(String[] args) {
String myString = "Hello Friends";
String[] str_arr = myString.split("");
for (String ch : str_arr) {
System.out.print(ch);
}
}
}
Output:
Hello Friends
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java