How to Add Char to String in Java
-
Java Add Char to String Using
+
Operator -
Java Add Char to String Using
StringBuilder.append()
-
Java Add Char to a String Using
String.format()
-
Java Add Char to String Using
Arrays.copyOf()
-
Java Add Char to String using
StringBuffer.insert()
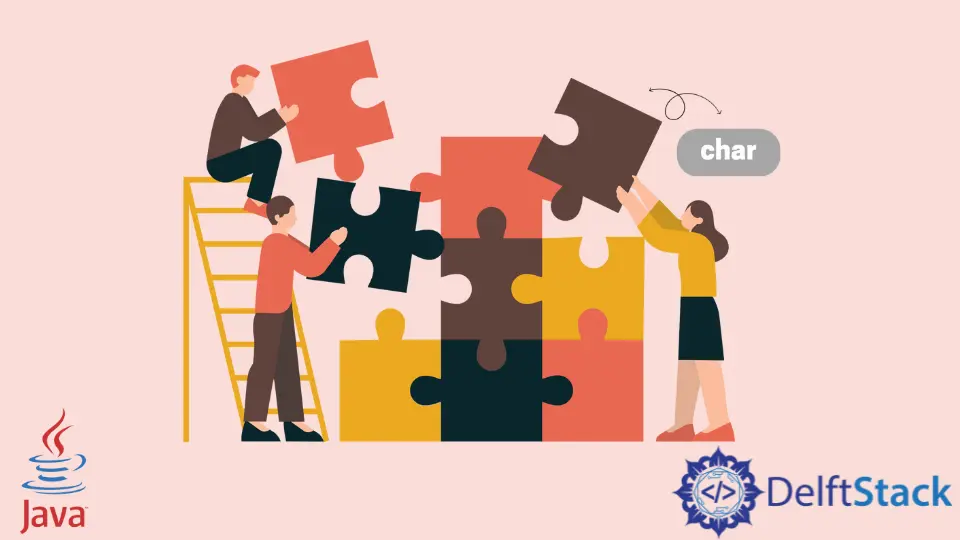
This article will introduce how we can add a character to a string in Java. A character in Java is represented by the data type char
, and it holds only a single value. We will use several methods to add char to string Java at different positions.
Java Add Char to String Using +
Operator
This is the easiest and most straightforward way to add a character to a string in Java. We concatenate a char to the string using the +
operator. In the program below, we have two char
values - charToAdd1
and charToAdd2
which we will concatenate with strings - alex
and bob
.
In the first variable - alex
, we have added charToAdd1
at the last position, while charToAdd2
is added in the middle. One thing to notice is that when we use the +
concatenation, any data type like char
will be converted to a string.
public class AddCharToString {
public static void main(String[] args) {
char charToAdd1 = 'A';
char charToAdd2 = 'C';
String alex = "Alex got Grade " + charToAdd1;
String bob = "While Bob got " + charToAdd2 + " Grade";
System.out.println(alex);
System.out.println(bob);
}
}
Output:
Alex got Grade A
While Bob got C Grade
Java Add Char to String Using StringBuilder.append()
StringBuffer
is a class in Java that provides a mutable sequence of characters. It allows you to efficiently manipulate strings by appending, inserting, or modifying characters without creating new objects for each operation.
This can significantly improve performance when dealing with frequent string modifications.
Here are some key characteristics of StringBuffer
:
- Mutability: Unlike regular strings,
StringBuffer
allows you to modify its content. - Thread-Safe:
StringBuffer
is synchronized, making it safe for use in multi-threaded environments. - Efficiency:
StringBuffer
performs string manipulation more efficiently compared to repeated string concatenation using the+
operator.
In this method, we add char to string using the append()
function of the StringBuilder
class in Java. This function appends two or more strings just like the +
operator.
In the below example, we create two StringBuilder
objects and then first append the charToAdd1
to alex
and then join charToAdd2
to bob
.
public class AddChartToString {
public static void main(String[] args) {
char charToAdd1 = 'A';
char charToAdd2 = 'C';
StringBuilder stringBuilder1 = new StringBuilder();
StringBuilder stringBuilder2 = new StringBuilder();
String alex = "Alex got Grade ";
String bob = "While Bob got Grade ";
stringBuilder1.append(alex).append(charToAdd1);
stringBuilder2.append(bob).append(charToAdd2);
System.out.println(stringBuilder1);
System.out.println(stringBuilder2);
}
}
Output:
Alex got Grade A
While Bob got Grade C
Java Add Char to a String Using String.format()
The String.format()
method in Java is a versatile tool for creating formatted strings. It uses a format string with placeholders that are replaced by the specified values. While commonly used for formatting numbers and strings, it can also be used to add characters to a string.
To add a character to a string using String.format()
, follow these steps:
- Create a Format String: Use a format string with a placeholder for the character. The
%c
specifier is used for characters. - Provide the Character: Pass the character that you want to add as an argument to the
String.format()
method. - Use the
String.format()
Method: Call theString.format()
method, passing the format string and the character as parameters.
Here’s an example:
public class CharToStringFormat {
public static void main(String[] args) {
char character = 'X';
String formattedString = String.format("Character: %c", character);
System.out.println(formattedString);
}
}
Output:
Character: X
Java Add Char to String Using Arrays.copyOf()
The Arrays.copyOf()
method in Java is part of the java.util.Arrays
class. It provides an efficient way to create a new array with a specified length and copy elements from an existing array. This method can be leveraged to add characters to a string.
To add a character to a string using Arrays.copyOf()
, follow these steps:
- Convert String to Character Array: Convert the string to a character array using the
toCharArray()
method. - Create a New Character Array: Use
Arrays.copyOf()
to create a new character array with additional space for the new character. - Add the Character: Assign the new character to the last index of the new character array.
- Convert Back to String: Convert the modified character array back to a string using the
String
constructor.
Here’s an example:
import java.util.Arrays;
public class CharToStringArrayCopy {
public static void main(String[] args) {
String originalString = "Hello";
char newChar = '!';
char[] charArray = originalString.toCharArray();
char[] newCharArray = Arrays.copyOf(charArray, charArray.length + 1);
newCharArray[newCharArray.length - 1] = newChar;
String modifiedString = new String(newCharArray);
System.out.println(modifiedString);
}
}
Output:
Hello!
Java Add Char to String using StringBuffer.insert()
The StringBuffer.insert()
method is part of the java.lang.StringBuffer
class in Java. It allows you to insert characters at any specified index within a string.
By carefully selecting the index, you can effectively add a character to the end of a string. This method provides flexibility and precision, making it a suitable choice for various scenarios.
To add a character to the end of a string using the StringBuffer.insert()
method, follow these steps:
- Create a
StringBuffer
Object: Initialize aStringBuffer
instance with the original content of the string. - Determine the Index: Since we want to add the character to the end of the string, the index will be the length of the current string.
- Use the
insert()
Method: Call theinsert()
method, passing the determined index and the character to add.
Here’s an example:
public class AddCharToEndOfString {
public static void main(String[] args) {
StringBuffer buffer = new StringBuffer("Hello");
char newChar = '!';
int index = buffer.length(); // Index is at the end
buffer.insert(index, newChar);
System.out.println(buffer);
}
}
Output:
Hello!
In this example, the insert()
method adds the character !
to the end of the string Hello
, resulting in the string Hello!
.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Char
- How to Convert Int to Char in Java
- Char vs String in Java
- How to Initialize Char in Java
- How to Represent Empty Char in Java
- How to Convert Char to Uppercase/Lowercase in Java
- How to Check if a Character Is Alphanumeric in Java