How to Convert List<string> to String in C#
-
Convert
List<string>
to String With the LINQ Method inC#
-
Convert
List<string>
to String With theString.Join()
Function inC#
-
Convert
List<string>
to String With theStringBuilder
Class inC#
- Conclusion
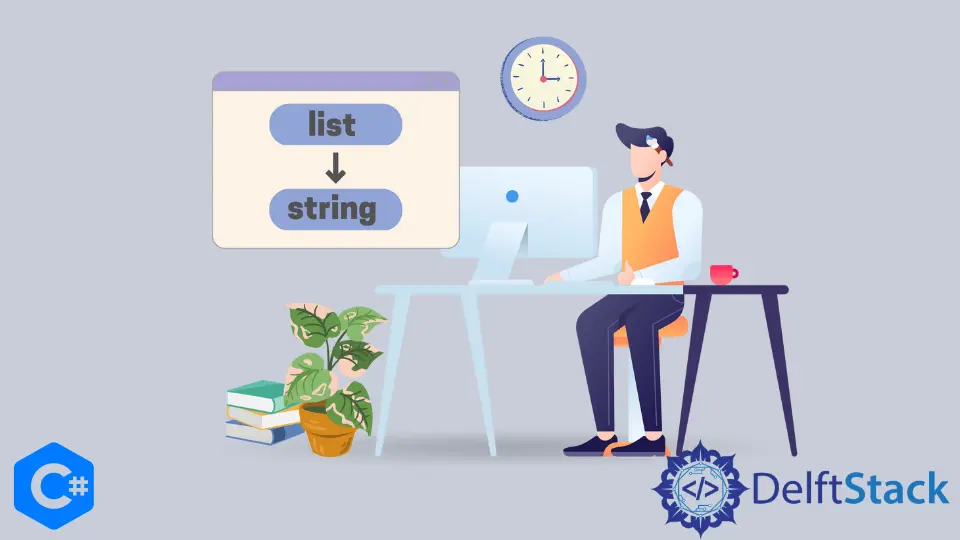
This guide explores three methods: LINQ’s Aggregate
for concise concatenation, String.Join()
for simplicity and readability, and StringBuilder
for optimal performance in memory-intensive operations.
Convert List<string>
to String With the LINQ Method in C#
The LINQ Aggregate
method concatenates elements of a list into a single string. This method is part of the LINQ (Language-Integrated Query) extension methods available in C#.
In this specific example, a list of names
is used, and the Aggregate
method is employed to join them together with commas in between.
using System;
using System.Collections.Generic;
using System.Linq;
namespace list_to_string {
class Program {
static void Main(string[] args) {
List<string> names = new List<string>() { "Ross", "Joey", "Chandler" };
string joinedNames = names.Aggregate((x, y) => x + ", " + y);
Console.WriteLine(joinedNames);
}
}
}
Output:
Ross, Joey, Chandler
The given C# code converts a list of names ("Ross," "Joey," "Chandler")
into a comma-separated string using the Aggregate()
function. It concatenates the elements, creating a string where each name is separated by a comma and space. The final result is displayed on the console, demonstrating a concise and readable approach to list-to-string conversion in C#.
This method is suitable when a concise approach to joining strings is desired, and the dataset size is relatively small. However, caution is advised for larger datasets as it may exhibit suboptimal performance.
Convert List<string>
to String With the String.Join()
Function in C#
The String.Join(separator, Strings)
function converts a list of strings into a single, comma-separated string. This method is part of the String
class in C# and provides a straightforward way to concatenate elements of a collection with a specified delimiter.
In this specific example, a list of names
is used, and the String.Join()
function is employed to join them together with commas in between.
using System;
using System.Collections.Generic;
namespace list_to_string {
class Program {
static void Main(string[] args) {
List<string> names = new List<string>() { "Ross", "Joey", "Chandler" };
string joinedNames = String.Join(", ", names.ToArray());
Console.WriteLine(joinedNames);
}
}
}
Output:
Ross, Joey, Chandler
The provided C# code utilizes the String.Join()
method to convert a list of names ("Ross," "Joey," "Chandler")
into a comma-separated string. By specifying the delimiter as ,
, the method efficiently concatenates the elements, forming a string where each name is separated by a comma and space. The resulting joined string is then printed to the console, showcasing a concise and readable approach to list-to-string conversion in C#.
This method is suitable when both performance and code readability are priorities. It is effective for moderate-sized datasets. Note that this method creates a new string, potentially leading to less memory-efficient operations, especially with very large strings.
Convert List<string>
to String With the StringBuilder
Class in C#
StringBuilder
in C# is a versatile tool for string manipulation, allowing efficient joining and modification of strings. It’s particularly useful for tasks involving dynamic changes or extensive concatenation operations.
In the given example, the ListToStringConverter
class demonstrates the straightforward use of StringBuilder
to convert a list of names into a comma-separated string.
using System;
using System.Collections.Generic;
using System.Text;
namespace list_to_string {
class Program {
static void Main() {
List<string> names = new List<string> { "Ross", "Joey", "Chandler" };
string joinedNames = ListToStringConverter.ConvertListToString(names);
Console.WriteLine(joinedNames);
}
}
public class ListToStringConverter {
public static string ConvertListToString(List<string> stringList) {
StringBuilder stringBuilder = new StringBuilder();
foreach (string element in stringList) {
stringBuilder.Append(element).Append(", ");
}
string joinedNames = stringBuilder.ToString().TrimEnd(',', ' ');
return joinedNames;
}
}
}
Output:
Ross, Joey, Chandler
In this C# code, a custom ListToStringConverter
class offers an alternative approach to convert a list of names ("Ross," "Joey," "Chandler")
into a comma-separated string. The class defines a static method, ConvertListToString
, which utilizes a StringBuilder
to iteratively concatenate the elements with a comma and space. The resulting string is then trimmed to remove the trailing comma and space. The Main
method in the Program
class showcases the application of this converter, underscoring a manual but controlled method for list-to-string conversion in C#.
This method is suitable when you need your code to be really fast, especially with a lot of data. It’s excellent at building strings on the go and using less memory, making it vital for handling substantial tasks efficiently.
Conclusion
The process of converting a List<string>
to a string in C# demands a thoughtful selection of methods tailored to specific application needs. The LINQ Aggregate
method, with its concise syntax, proves advantageous for smaller datasets despite potential performance considerations. Meanwhile, the String.Join()
function strikes a balance between simplicity and efficiency, making it suitable for moderate-sized data. On the other hand, the StringBuilder
class, with its manual yet controlled approach, emerges as the optimal choice for performance and memory-intensive operations, particularly in scenarios involving substantial string manipulations. By understanding the strengths and considerations of each method, C# developers can make informed decisions for efficient and effective list-to-string conversions in their applications.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedInRelated Article - Csharp List
- How to Convert an IEnumerable to a List in C#
- How to Remove Item From List in C#
- How to Join Two Lists Together in C#
- How to Get the First Object From List<Object> Using LINQ
- How to Find Duplicates in a List in C#
- The AddRange Function for List in C#