在 C# 中將 List<string>轉換為字串
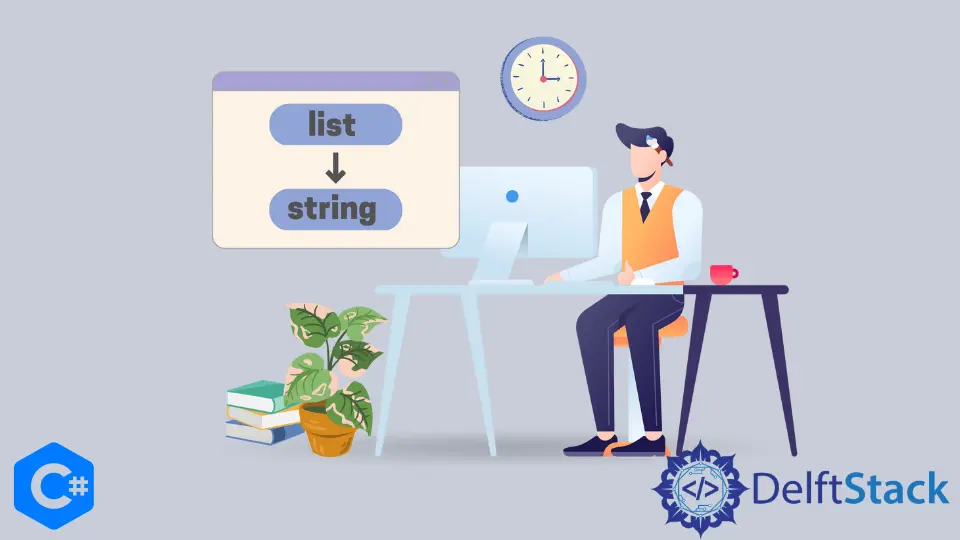
在本教程中,我們將討論在 C# 中把 List<string>
轉換為字串變數的方法。
使用 C# 中的 Linq 方法將 List<string>
轉換為字串
Linq 或語言整合查詢可以在 C# 中執行健壯的文字操作。Linq 具有 Aggregate()
函式,可以將字串列表轉換為字串變數。以下程式碼示例向我們展示瞭如何使用 C# 中的 Linq 方法將 List<string>
轉換為字串。
using System;
using System.Collections.Generic;
using System.Linq;
namespace list_to_string {
class Program {
static void Main(string[] args) {
List<string> names = new List<string>() { "Ross", "Joey", "Chandler" };
string joinedNames = names.Aggregate((x, y) => x + ", " + y);
Console.WriteLine(joinedNames);
}
}
}
輸出:
Ross, Joey, Chandler
我們建立字串列表 names
,並在 names
中插入值 { "Ross", "Joey", "Chandler" }
。然後,使用 Aggregate()
函式將 names
列表中的字串用 ,
作為它們之間的分隔符連線起來。
此方法非常慢,不建議使用。這與執行 foreach
迴圈並連線每個元素相同。
使用 C# 中的 String.Join()
函式將 List<string>
轉換為字串
String.Join(separator, Strings)
函式可以在 C# 中用指定的 separator
連線 Strings
。String.Join()
函式返回通過將 Strings
引數與指定的 separator
連線而形成的字串。
下面的程式碼示例向我們展示瞭如何使用 C# 中的 String.Join()
函式將 List<string>
轉換為字串。
using System;
using System.Collections.Generic;
namespace list_to_string {
class Program {
static void Main(string[] args) {
List<string> names = new List<string>() { "Ross", "Joey", "Chandler" };
string joinedNames = String.Join(", ", names.ToArray());
Console.WriteLine(joinedNames);
}
}
}
輸出:
Ross, Joey, Chandler
我們建立字串列表 names
,並在 names
中插入值 { "Ross", "Joey", "Chandler" }
。然後,使用 C# 中的 String.Join()
函式將 names
列表中的字串用 ,
作為它們之間的分隔符連線起來。
此方法快得多,並且比以前的方法更好。
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn