How to Get a Substring in C
-
Use the
memcpy()
Function to Get a Substring in C -
Use the
strncpy()
Function to Get a Substring in C - Use Pointer Arithmetic to Get a Substring in C
- Use Array Indexing and Null Termination to Get a Substring in C
- Conclusion
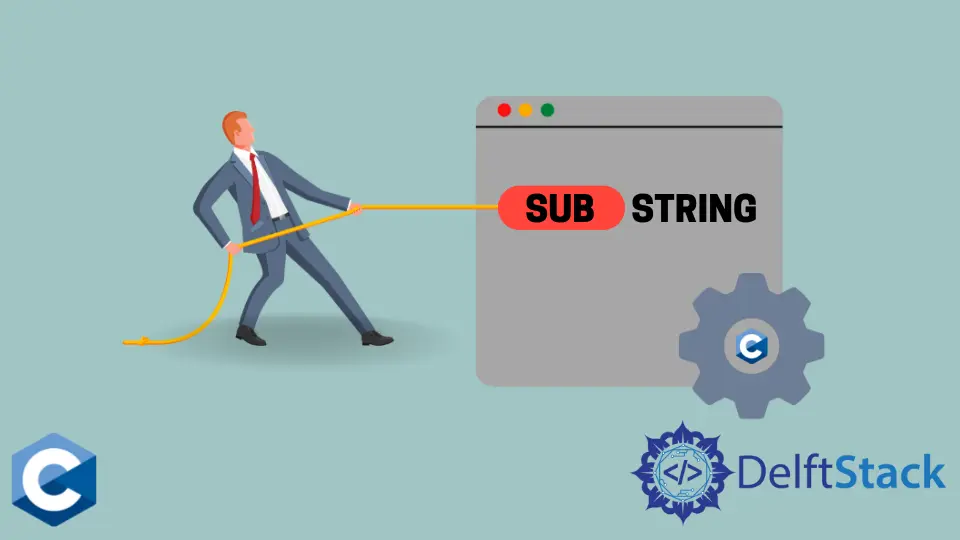
In this article, we will explore the use of the memcpy()
function, strncpy()
function, pointer arithmetic, and array indexing with null termination to extract substrings in C. We will discuss the syntax, usage, and steps involved in each approach, providing practical examples to demonstrate their application.
Use the memcpy()
Function to Get a Substring in C
The memcpy()
function in C is a fundamental tool used to copy a specified number of characters from a source memory area to a destination memory area. This function is part of the standard C library and is available in the <string.h>
header file.
One important consideration when using memcpy()
is that it does not perform checks for overlapping memory regions between the source and destination. This can lead to unexpected behavior if overlapping occurs.
Additionally, memcpy()
does not validate for null addresses or buffer overflow, making it essential for the programmer to ensure proper memory allocation and valid addresses.
The syntax of memcpy()
is straightforward:
void *memcpy(void *destination_string, const void *source_string, size_t number);
destination_string
: Pointer to the destination memory area where the content will be copied.source_string
: Pointer to the source memory area from which the data will be copied.number
: Number of characters (or bytes) to be copied.
This function returns a pointer to the destination string, allowing for potential chaining of operations.
To extract a substring using memcpy()
, follow these steps:
-
Determine the length of the substring.
-
Allocate memory for the destination buffer.
-
Use
memcpy()
to copy the substring from the source buffer to the destination buffer. -
Ensure the destination buffer is null-terminated to form a valid C string.
Let’s delve into a practical example to demonstrate the application of memcpy()
in extracting a substring from a source string:
#include <stdio.h>
#include <string.h>
int main(void)
{
char *text = "The test string is here";
char subtext[7];
// Using memcpy to extract a substring
memcpy(subtext, &text[9], 6);
subtext[6] = '\0'; // Null-terminate the substring
// Displaying the original string and the extracted substring
printf("The original string is: %s\n", text);
printf("Extracted substring is: %s", subtext);
return 0;
}
In this C program, we’re demonstrating how to use the memcpy()
function to extract a substring from a given text. First, we include the necessary header files: stdio.h
for input/output operations and string.h
for string-related functions like memcpy()
.
Next, we declare a pointer text
and assign it a string value, "The test string is here"
. We also create an array subtext
of size 7 to hold the extracted substring.
To extract the substring, we utilize the memcpy()
function. We pass the subtext
array as the destination, and we specify the starting point of the substring in the text
string (9th character or index 9) with a length of 6 characters.
After the memcpy()
operation, we null-terminate the subtext
array by placing a null character ('\0'
) at the end.
Lastly, we display the original string (text
) and the extracted substring (subtext
) using printf()
statements. The original string is printed as is, and the extracted substring is printed accordingly.
When we run the program, it will output:
The original string is: The test string is here
Extracted substring is: string
Use the strncpy()
Function to Get a Substring in C
The strncpy()
function in C serves a similar purpose to the well-known strcpy()
function, with a distinct feature: it allows the copying of a specified number of characters from the source to the destination. This function is a part of the standard C library and is included in the <string.h>
header file.
The strncpy()
function is primarily used to copy a given number of characters from a source string to a destination string.
Its syntax is as follows:
char *strncpy(char *destination_string, const char *source_string, size_t number);
destination_string
: Pointer to the destination string where the content will be copied.source_string
: Pointer to an array of originalchar
values (the source string).number
: Number of characters to be copied.
This function returns a pointer to the destination string after performing the copy operation.
An important aspect of strncpy()
is its behavior when encountering a null character ('\0'
) in the source_string
. If such a null character is encountered, the strncpy()
function will appropriately add null characters to the destination_string
to ensure the string is null-terminated.
To extract a substring using strncpy()
, follow these steps:
-
Determine the length of the substring you want to extract.
-
Allocate memory for the destination buffer to hold the substring.
-
Use
strncpy()
to copy the substring from the source buffer to the destination buffer. -
Ensure the destination buffer is null-terminated to form a valid C string.
Let’s have a practical example to show how strncpy()
can be used to extract a substring from a given string:
#include <stdio.h>
#include <string.h>
int main(void)
{
char *text = "The test string is here";
char subtext[7];
// Using strncpy to extract a substring
strncpy(subtext, &text[9], 6);
subtext[6] = '\0'; // Null-terminate the substring
// Displaying the original string and the extracted substring
printf("The original string is: %s\n", text);
printf("Extracted substring is: %s", subtext);
return 0;
}
In this C program, we are showcasing how to extract a substring from a given text using the strncpy()
function. We start by including the necessary header files: stdio.h
for input/output operations and string.h
for string-related functions like strncpy()
.
Next, we declare a pointer text
and assign it a string value, "The test string is here"
. Additionally, we create an array subtext
of size 7 to hold the extracted substring.
The extraction of the substring is performed using the strncpy()
function. We pass the subtext
array as the destination, and we specify the starting point of the substring in the text
string (9th character or index 9) with a length of 6 characters.
After the strncpy()
operation, we null-terminate the subtext
array by placing a null character ('\0'
) at the end.
Finally, we display the original string (text
) and the extracted substring (subtext
) using printf()
statements. The original string is printed as is, and the extracted substring is printed accordingly.
When the program is run, it will output:
The original string is: The test string is here
Extracted substring is: string
Use Pointer Arithmetic to Get a Substring in C
Another way to extract a substring is through the use of pointer arithmetic. Pointer arithmetic allows us to manipulate memory addresses and navigate through the character array to extract the desired substring efficiently.
In the context of extracting substrings, we can use pointer arithmetic to navigate through the character array and selectively copy characters to form the substring.
To extract a substring using pointer arithmetic, follow these steps:
-
Determine the length of the substring you want to extract.
-
Create a destination buffer (an array) to hold the extracted substring.
-
Utilize pointer arithmetic to navigate through the source character array and copy the desired characters to the destination buffer.
-
Ensure the destination buffer is null-terminated to create a valid C string.
Let’s illustrate how to extract a substring using pointer arithmetic in C:
#include <stdio.h>
void extractSubstring(const char *source, int start_index, int length, char *substring) {
// Pointer arithmetic to copy the substring
for (int i = 0; i < length; i++) {
*(substring + i) = *(source + start_index + i);
}
substring[length] = '\0'; // Null-terminate the substring
}
int main() {
const char str[] = "Hello, World!";
int start_index = 7; // Start index of the substring
int length = 5; // Length of the substring
char substring[length + 1]; // +1 for null terminator
// Extract and print the substring using pointer arithmetic
extractSubstring(str, start_index, length, substring);
printf("Extracted Substring: %s\n", substring);
return 0;
}
In this C program, we begin by declaring a function extractSubstring
which takes four parameters: source
(the original string), start_index
(the starting index of the substring), length
(the length of the substring), and substring
(the buffer to hold the extracted substring).
The extractSubstring
function uses pointer arithmetic to navigate through the source
character array. It iterates over the characters of the substring using a for
loop and uses pointer dereferencing to copy each character from the source
to the substring
.
After copying the required characters, it null-terminates the substring
by placing a null character ('\0'
) at the end.
In the main
function, we initialize a character array str
with a sample text, "Hello, World!"
. We specify the start_index
(7th index) and length
(5 characters) for the desired substring.
We create a substring
array to store the extracted substring, ensuring it is large enough to accommodate the substring and the null terminator.
We then call the extractSubstring
function and pass the necessary parameters to extract the substring using pointer arithmetic.
Finally, we print the extracted substring using printf
.
When the program is executed, it will output:
Extracted Substring: World
Use Array Indexing and Null Termination to Get a Substring in C
Extracting a substring from a character array (string) can also be done by utilizing array indexing and null termination.
Array indexing involves accessing elements of an array using an index or position within square brackets. In C, array indexing starts from 0 for the first element, allowing us to easily traverse through the characters of a string.
Null termination, represented by the null character ('\0'
), signifies the end of a C string. It is essential for creating valid strings in C and is used to terminate the string in memory.
To extract a substring using array indexing and null termination, follow these steps:
-
Determine the length of the substring you want to extract.
-
Create a destination buffer (an array) to hold the extracted substring.
-
Utilize array indexing to navigate through the source character array and copy the desired characters to the destination buffer.
-
Ensure the destination buffer is null-terminated to create a valid C string.
Let’s illustrate how to extract a substring using array indexing and null termination in C:
#include <stdio.h>
void extractSubstring(const char *source, int start_index, int end_index) {
// Calculate the length of the substring
int length = end_index - start_index + 1;
char substring[length + 1]; // +1 for null terminator
// Copy the substring using array indexing
for (int i = 0; i < length; i++) {
substring[i] = source[start_index + i];
}
substring[length] = '\0'; // Null-terminate the substring
// Print the extracted substring
printf("Extracted Substring: %s\n", substring);
}
int main() {
const char str[] = "Hello, World!";
int start_index = 7; // Start index of the substring
int end_index = 12; // End index of the substring
// Extract and print the substring
extractSubstring(str, start_index, end_index);
return 0;
}
Here, the extractSubstring
function is defined to extract a substring from a given source string. It takes three parameters:
Source
: The original string.Start_index
: The starting index of the substring.End_index
: The ending index of the substring.
First, the function calculates the length of the desired substring based on the provided indices.
Next, a character array named substring
is created with a size equal to the length of the substring plus one to account for the null terminator. The null terminator ('\0'
) is essential to mark the end of the substring and create a valid C string.
The function then uses a for
loop to copy characters from the source
array to the substring
array using array indexing. It iterates through the characters of the substring and copies them one by one.
After copying the required characters, the function adds a null terminator at the end of the substring
to ensure it’s a valid C string.
In the main
function, a sample text str
is defined, and indices for the desired substring (start_index
and end_index
) are specified. The extractSubstring
function is called, passing the necessary parameters to extract the substring.
Finally, the extracted substring is printed to the console using printf
.
When the program is executed, it will output:
Extracted Substring: World
Conclusion
In C programming, extracting substrings is a fundamental operation with several approaches available. We discussed the utilization of the memcpy()
function, strncpy()
function, pointer arithmetic, and array indexing with null termination.
The memcpy()
and strncpy()
functions offer direct ways to copy substrings with specified lengths. Alternatively, pointer arithmetic and array indexing with null termination provide flexibility in navigating through the character array and extracting the desired substring efficiently.
By understanding and implementing these techniques, developers can efficiently obtain substrings from larger strings, a crucial functionality in numerous C programming applications.
Related Article - C Char
- How to Get Length of Char Array in C
- How to Use the getchar Function in C
- How to Compare Char in C
- How to Convert Integer to Char in C