C에서 부분 문자열 얻기
Satishkumar Bharadwaj
2023년10월12일
C
C Char
C String
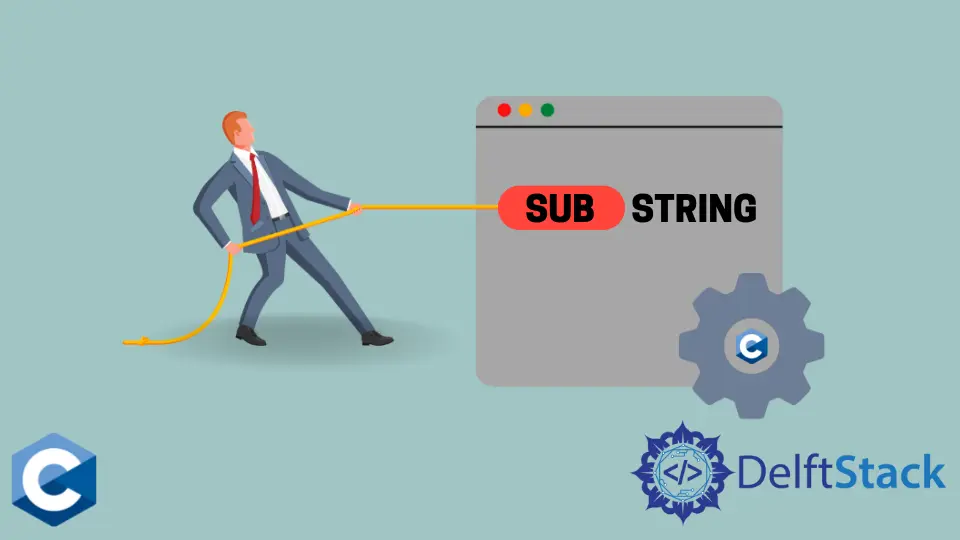
이 튜토리얼에서는 C의 문자 값에서 하위 문자열을 가져 오는 방법을 소개합니다.memcpy()
및strncpy()
와 같은 문자에서 하위 문자열을 가져 오는 다른 방법이 있습니다.
C에서 하위 문자열을 가져 오는memcpy()
함수
memcpy()
함수는 소스에서 대상의 메모리 영역으로 문자 수를 복사합니다. 이 함수는<string.h>
헤더 파일에서 사용할 수 있습니다.
이 기능은 소스와 목적지의 주소가 겹칠 때 문제를 발생시킵니다. 이 함수는 null 주소 또는 오버플로 발생 여부를 확인하지 않습니다.
memcpy()
함수는 대상 문자열에 대한 포인터를 반환합니다. 오류를 표시 할 반환 값이 없습니다.
memcpy()
구문
void *memcpy(void *destination_string, const void *source_string,
size_t number);
destination_string
은 대상 문자열에 대한 포인터입니다.source_string
은 원래 문자 유형 값의 배열에 대한 포인터입니다.number
는 문자 수입니다.
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
memcpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
출력:
The original string is: The test string is here
Substring is: string
C에서 하위 문자열을 가져 오는strncpy()
함수
strncpy()
함수는strcpy()
함수와 동일합니다. 유일한 차이점은strncpy()
함수가 지정된 수의 문자를 소스 문자열에서 대상 문자열로 복사한다는 것입니다. strncpy()
함수는<string.h>
헤더 파일에서 사용할 수 있습니다.
이 함수는 소스 문자열을 복사 한 후 대상 문자열에 대한 포인터를 반환합니다.
strncpy()
구문
void *strncpy(void *destination_string, const void *source_string,
size_t number);
destination_string
은 대상 문자열에 대한 포인터입니다.source_string
은 원래char
값의 배열에 대한 포인터입니다.number
는 문자 수입니다.
strncpy()
함수가source_string
에서 널 문자를 발견하면 함수는destination_string
에 널 문자를 추가합니다.
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
strncpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
출력:
The original string is: The test string is here
Substring is: string
튜토리얼이 마음에 드시나요? DelftStack을 구독하세요 YouTube에서 저희가 더 많은 고품질 비디오 가이드를 제작할 수 있도록 지원해주세요. 구독하다