如何在 C 語言中獲取子字串
Satishkumar Bharadwaj
2023年10月12日
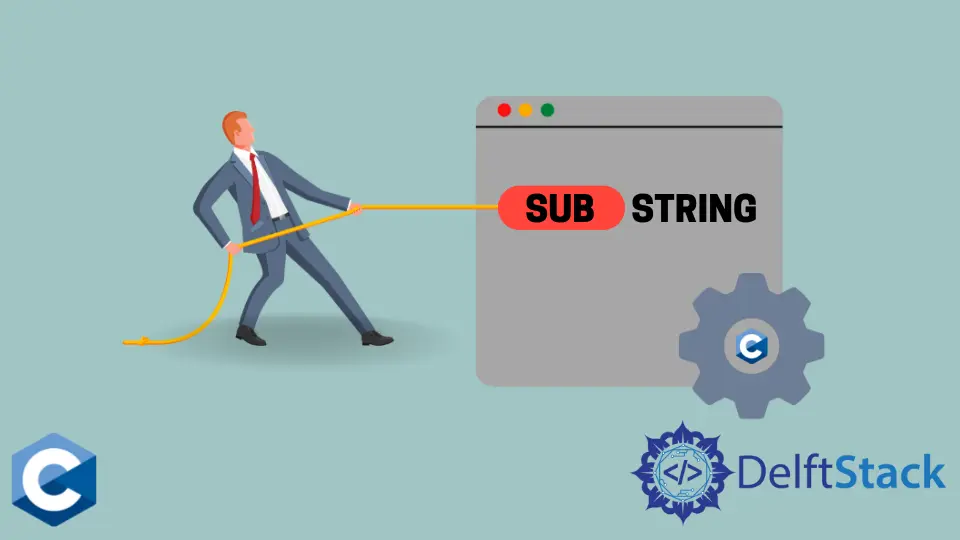
本教程介紹瞭如何在 C 語言中從字串中獲取子字串,從字元中獲取子字串的方法有 memcpy()
和 strncpy()
等。
memcpy()
函式在 C 語言中獲取子字串
memcpy()
函式將原始檔中的字元數複製到目標檔案的記憶體區。這個函式在 <string.h>
標頭檔案中可用。
當源地址和目標地址重疊時,這個函式會產生問題。這個函式不檢查地址是否為空,也不檢查是否發生溢位。
memcpy()
函式返回一個指向目標字串的指標。沒有返回值來顯示錯誤。
memcpy()
的語法
void *memcpy(void *destination_string, const void *source_string,
size_t number);
destination_string
是指向目標字串的指標。source_string
是指向原始字元型別值陣列的指標。number
是字元數。
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
memcpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
輸出:
The original string is: The test string is here
Substring is: string
strncpy()
函式在 C 語言中獲取子字串
strncpy()
函式與 strcpy()
函式相同。唯一不同的是,strncpy()
函式將給定數量的字元從源字串複製到目標字串。strncpy()
函式在 <string.h>
標頭檔案中可用。
該函式在複製源字串後返回一個指向目標字串的指標。
strncpy()
的語法
void *strncpy(void *destination_string, const void *source_string,
size_t number);
destination_string
是指向目標字串的指標。source_string
是指向原始字元值陣列的指標。number
是字元數。
如果 strncpy()
函式在 source_string
中遇到一個空字元,該函式將在 destination_string
中新增空字元。
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
strncpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
輸出:
The original string is: The test string is here
Substring is: string